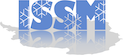 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
59 Mesh(
int* index,
double* x,
double* y,
int nods,
int nels,
BamgOpts* bamgopts);
107 void ReadMesh(
int* index,
double* x,
double* y,
int nods,
int nels,
BamgOpts* bamgopts);
123 for (
int i=0;i<
nbt;i++)
triangles[i].SetSingleVertexToTriangleConnectivity();
126 for (
int i=0;i<
nbt;i++)
131 for (
int i=0;i<
nbv;i++)
vertices[i].GeomEdgeHook=NULL;
136 for (
int i=0;i<
nbv;i++)
vertices[i].GeomEdgeHook=NULL;
149 long long & detsa,
long long & detsb,
int & nbswap);
154 void swap(Triangle *t1,
short a1,
155 Triangle *t2,
short a2,
156 BamgVertex *s1,BamgVertex *s2,
long long det1,
long long det2);
long GetId(const Triangle &t) const
Edge ** MakeGeomEdgeToEdge()
long NbVerticesOnGeomVertex
static const short PreviousEdge[3]
BamgVertex & operator[](long i)
void Triangulate(double *x, double *y, int nods, BamgOpts *bamgopts)
BamgVertex * NearestVertex(int i, int j)
place holder for optimization function arguments
Mesh(BamgGeom *bamggeom, BamgMesh *bamgmesh, BamgOpts *bamgopts)
long RandomNumber(long max)
AdjacentTriangle Adj() const
int ForceEdge(BamgVertex &a, BamgVertex &b, AdjacentTriangle &taret)
void ReadMesh(int *index, double *x, double *y, int nods, int nels, BamgOpts *bamgopts)
AdjacentTriangle Adj(const AdjacentTriangle &a)
CrackedEdge * CrackedEdges
VertexOnEdge * VertexOnBThEdge
Metric MetricAt(const R2 &)
void AddVertex(BamgVertex &s, Triangle *t, long long *=0)
void ForceBoundary(BamgOpts *bamgopts)
void CrackMesh(BamgOpts *bamgopts)
VertexOnGeom * VerticesOnGeomVertex
ListofIntersectionTriangles lIntTria
void SmoothMetric(BamgOpts *bamgopts, double raisonmax)
void SetIntCoor(const char *from=0)
void TriangulateFromGeom0(BamgOpts *bamgopts)
void SetVertexFieldOnBTh()
void BoundAnisotropy(BamgOpts *bamgopts, double anisomax, double hminaniso=1e-100)
void BuildMetric1(BamgOpts *bamgopts)
void BuildGeometryFromMesh(BamgOpts *bamgopts=NULL)
void ReconstructExistingMesh(BamgOpts *bamgopts)
void WriteMetric(BamgOpts *bamgopts)
AdjacentTriangle Next(const AdjacentTriangle &ta)
void CreateSingleVertexToTriangleConnectivity()
void BuildMetric0(BamgOpts *bamgopts)
GeomEdge * ProjectOnCurve(Edge &AB, BamgVertex &A, BamgVertex &B, double theta, BamgVertex &R, VertexOnEdge &BR, VertexOnGeom &GR)
void MaxSubDivision(BamgOpts *bamgopts, double maxsubdiv)
void SmoothingVertex(BamgOpts *bamgopts, int=3, double=0.3)
void WriteIndex(int **pindex, int *pnels)
void NewPoints(Mesh &, BamgOpts *bamgopts, int KeepVertices=1)
void TriangleIntNumbering(long *renumbering)
void UnMarkUnSwapTriangle()
R2 I2ToR2(const I2 &P) const
AdjacentTriangle CloseBoundaryEdge(I2 A, Triangle *t, double &a, double &b)
I2 R2ToI2(const R2 &P) const
void ReadMetric(const BamgOpts *bamgopts)
long NbVerticesOnGeomEdge
long SplitInternalEdgeWithBorderVertices()
int SwapForForcingEdge(BamgVertex *&pva, BamgVertex *&pvb, AdjacentTriangle &tt1, long long &dets1, long long &detsa, long long &detsb, int &nbswap)
void FindSubDomain(BamgOpts *bamgopts, int OutSide=0)
void WriteMesh(BamgMesh *bamgmesh, BamgOpts *bamgopts)
long TriangleReferenceList(long *) const
Triangle & operator()(long i)
void AddMetric(BamgOpts *bamgopts)
long InsertNewPoints(long nbvold, long &NbTSwap, BamgOpts *bamgopts)
Triangle * TriangleFindFromCoord(const I2 &, long long[3], Triangle *tstart=0)
void swap(Triangle *t1, short a1, Triangle *t2, short a2, BamgVertex *s1, BamgVertex *s2, long long det1, long long det2)
IssmDouble max(IssmDouble a, IssmDouble b)
void TriangulateFromGeom1(BamgOpts *bamgopts, int KeepVertices=1)
AdjacentTriangle Previous(const AdjacentTriangle &ta)
BamgVertex ** orderedvertices
const Triangle & operator()(long i) const
void TrianglesRenumberBySubDomain(bool justcompress=false)
void Insert(BamgOpts *bamgopts)
VertexOnVertex * VertexOnBThVertex
const BamgVertex & operator[](long i) const
static const short NextEdge[3]
VertexOnGeom * VerticesOnGeomEdge