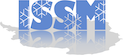 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
5 #include "../shared/shared.h"
31 Metric(
double a,
double b,
double c);
37 inline void Box(
double &hx,
double &hy)
const;
43 double Length(
double Ax,
double Ay)
const;
91 double L[1024],
S[1024];
102 if (coef<=1.00000000001){
116 double v00=M.
vx*M.
vx;
117 double v11=M.
vy*M.
vy;
118 double v01=M.
vx*M.
vy;
129 return (
Abs(la - lb) < 1.0e-6*
Max3(la,lb,1.0e-20) ) ? (la+lb)/2 : la*lb*log(la/lb)/(la-lb);
132 return (
Abs(la - lb) <1.0e-6*
Max3(la,lb,1.0e-20)) ? s : (exp(s*lab*(la-lb)/(la*lb))-1)*lb/(la-lb);
SaveMetricInterpole LastMetricInterpole
R2 Orthogonal(const I2 x)
double operator()(R2 x, R2 y) const
void Box(double &hx, double &hy) const
void SimultaneousMatrixReduction(Metric M1, Metric M2, D2xD2 &V)
double abscisseInterpole(const Metric &Ma, const Metric &Mb, R2 AB, double s, int optim)
void operator*=(double coef)
Metric operator/(double c) const
friend double abscisseInterpole(const Metric &Ma, const Metric &Mb, R2, double s, int optim)
T Max3(const T &a, const T &b, const T &c)
R2 Orthogonal(const R2 x)
T Max(const T &a, const T &b)
void BoundAniso2(const double coef)
double LengthInterpole(const Metric &Ma, const Metric &Mb, R2 AB)
EigenMetric(const Metric &)
double Length(double Ax, double Ay) const
P2xP2< double, double > D2xD2
int IntersectWith(const Metric &M2)
T Min(const T &a, const T &b)
Metric operator*(double c) const
friend double LengthInterpole(const Metric &Ma, const Metric &Mb, R2 AB)