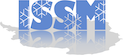 |
Ice Sheet System Model
4.18
Code documentation
|
GeomEdge & operator()(long i)
place holder for optimization function arguments
const GeomEdge & operator()(long i) const
GeomSubDomain * subdomains
GeomEdge * ProjectOnCurve(const Edge &, double, BamgVertex &, VertexOnGeom &) const
void PostRead(bool checkcurve=false)
void ReadGeometry(BamgGeom *bamggeom, BamgOpts *bamgopts)
long GetId(const GeomVertex &t) const
GeomVertex & operator[](long i)
I2 R2ToI2(const R2 &P) const
const GeomVertex & operator[](long i) const
void WriteGeometry(BamgGeom *bamggeom, BamgOpts *bamgopts)