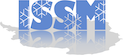 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the source code of this file.
◆ solutionsequence_thermal_nonlinear()
void solutionsequence_thermal_nonlinear |
( |
FemModel * |
femmodel | ) |
|
Definition at line 11 of file solutionsequence_thermal_nonlinear.cpp.
27 bool isenthalpy, isdynamicbasalspc;
28 int constraints_converged;
29 int num_unstable_constraints;
31 int thermal_penalty_threshold;
63 delete tf_old;tf_old=tf;
68 if(isdynamicbasalspc){
82 convergence(&converged,Kff,pf,tf,tf_old,0.05,eps_rel,NAN);
85 delete Kff;
delete pf;
delete df;
95 bool max_iteration_state=
false;
96 if(count>=thermal_maxiter){
97 _printf0_(
" maximum number of nonlinear iterations (" << thermal_maxiter <<
") exceeded\n");
101 max_iteration_state=
true;
113 if(num_unstable_constraints<=thermal_penalty_threshold) converged=
true;
114 if(count>=thermal_maxiter){
116 _printf0_(
" maximum number of iterations (" << thermal_maxiter <<
") exceeded\n");
◆ solutionsequence_hydro_nonlinear()
void solutionsequence_hydro_nonlinear |
( |
FemModel * |
femmodel | ) |
|
Definition at line 12 of file solutionsequence_hydro_nonlinear.cpp.
38 bool sedconverged,eplconverged,hydroconverged;
39 bool isefficientlayer;
40 int constraints_converged;
41 int num_unstable_constraints;
42 int sedcount,eplcount,hydrocount;
57 if(!isefficientlayer) hydroconverged=
true;
62 if(isefficientlayer) {
84 ug_sed->
Copy(ug_sed_main_iter);
86 ug_epl->
Copy(ug_epl_main_iter);
101 uf_sed->
Copy(uf_sed_sub_iter);
115 delete Kff;
delete pf;
delete df;
123 if(num_unstable_constraints==0) sedconverged =
true;
124 if (sedcount>=hydro_maxiter){
125 _error_(
" maximum number of Sediment iterations (" << hydro_maxiter <<
") exceeded");
130 if(sedconverged)
break;
138 uf_sed_sub_iter->
Copy(duf);
139 duf->
AYPX(uf_sed,-1.0);
143 if (xIsNan<IssmDouble>(ndu_sed) || xIsNan<IssmDouble>(nu_sed))
_error_(
"convergence criterion is NaN!");
144 if (ndu_sed==0.0 && nu_sed==0.0) nu_sed=1.0e-6;
145 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Inner Sediment Convergence criterion:" << ndu_sed/nu_sed*100 <<
"%, aiming lower than " << eps_hyd*10*100 <<
" %\n");
146 if((ndu_sed/nu_sed)<eps_hyd*10.){
150 delete uf_sed_sub_iter;
161 if(isefficientlayer){
172 ug_epl->
Copy(ug_epl_sub_iter);
198 delete Kff;
delete pf;
delete df;
199 delete uf_epl_sub_iter;
201 uf_epl->
Copy(uf_epl_sub_iter);
208 ug_epl_sub_iter->
Copy(dug);
209 dug->
AYPX(ug_epl,-1.0);
213 if (xIsNan<IssmDouble>(ndu_epl) || xIsNan<IssmDouble>(nu_epl))
_error_(
"convergence criterion is NaN!");
214 if (ndu_epl==0.0 && nu_epl==0.0) nu_epl=1.0e-6;
215 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Inner EPL Convergence criterion:" << ndu_epl/nu_epl*100 <<
"%, aiming lower than " << eps_hyd*10*100 <<
" %\n");
216 if((ndu_epl/nu_epl)<eps_hyd*10.) eplconverged=
true;
217 if (eplcount>=hydro_maxiter){
218 _error_(
" maximum number of EPL iterations (" << hydro_maxiter <<
") exceeded");
222 delete ug_epl_sub_iter;
237 ug_sed_main_iter->
Copy(dug);
238 dug->
AYPX(ug_sed,-1.0);
242 delete ug_sed_main_iter;
243 if (xIsNan<IssmDouble>(ndu_sed) || xIsNan<IssmDouble>(nu_sed))
_error_(
"Sed convergence criterion is NaN!");
244 if (ndu_sed==0.0 && nu_sed==0.0) nu_sed=1.0e-6;
246 ug_epl_main_iter->
Copy(dug);
247 dug->
AYPX(ug_epl,-1.0);
251 delete ug_epl_main_iter;
252 if (xIsNan<IssmDouble>(ndu_epl) || xIsNan<IssmDouble>(nu_epl))
_error_(
"EPL convergence criterion is NaN!");
253 if (ndu_epl==0.0 && nu_epl==0.0) nu_epl=1.0e-6;
254 if (!xIsNan<IssmDouble>(eps_hyd)){
255 if ((ndu_epl/nu_epl)<eps_hyd && (ndu_sed/nu_sed)<(eps_hyd)){
260 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Sediment Convergence criterion:" << ndu_sed/nu_sed*100 <<
"%, aiming lower than " << eps_hyd*100 <<
" %\n");
261 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" EPL Convergence criterion:" << ndu_epl/nu_epl*100 <<
"%, aiming lower than " << eps_hyd*100 <<
" %\n");
262 hydroconverged=
false;
265 else _printf0_(setw(50) << left <<
" Convergence criterion:" << ndu_sed/nu_sed*100 <<
" %\n");
266 if (hydrocount>=hydro_maxiter){
267 _error_(
" maximum number for hydrological global iterations (" << hydro_maxiter <<
") exceeded");
271 if(hydroconverged)
break;
282 delete uf_epl_sub_iter;
◆ solutionsequence_shakti_nonlinear()
void solutionsequence_shakti_nonlinear |
( |
FemModel * |
femmodel | ) |
|
Definition at line 11 of file solutionsequence_shakti_nonlinear.cpp.
24 int max_nonlinear_iterations;
43 delete old_uf;old_uf=uf;
54 convergence(&converged,Kff,pf,uf,old_uf,eps_res,eps_rel,eps_abs);
delete Kff;
delete pf;
delete df;
59 if(count>=max_nonlinear_iterations){
60 _printf0_(
" maximum number of nonlinear iterations (" << max_nonlinear_iterations <<
") exceeded\n");
◆ solutionsequence_glads_nonlinear()
void solutionsequence_glads_nonlinear |
( |
FemModel * |
femmodel | ) |
|
Definition at line 11 of file solutionsequence_glads_nonlinear.cpp.
24 int max_nonlinear_iterations;
36 bool converged_out=
false;
38 bool converged_in=
false;
44 while(!converged_out){
51 delete old_uf;old_uf=uf;
62 convergence(&converged_in,Kff,pf,uf,old_uf,eps_res,eps_rel,eps_abs);
delete Kff;
delete pf;
delete df;
67 if(count_in>=max_nonlinear_iterations && !converged_in){
68 _printf0_(
" maximum number of nonlinear iterations of inner loop (" << max_nonlinear_iterations <<
") exceeded\n");
79 if(count_in==1) converged_out =
true;
83 if(count_out>=max_nonlinear_iterations && !converged_out){
84 _printf0_(
" maximum number of nonlinear iterations of outer loop (" << max_nonlinear_iterations <<
") exceeded\n");
◆ solutionsequence_nonlinear()
void solutionsequence_nonlinear |
( |
FemModel * |
femmodel, |
|
|
bool |
conserve_loads |
|
) |
| |
Definition at line 11 of file solutionsequence_nonlinear.cpp.
23 Loads* savedloads=NULL;
25 int constraints_converged;
26 int num_unstable_constraints;
30 int min_mechanical_constraints;
31 int max_nonlinear_iterations;
32 int configuration_type;
68 delete old_uf;old_uf=uf;
83 convergence(&converged,Kff,pf,uf,old_uf,eps_res,eps_rel,eps_abs);
89 delete Kfs;
delete Kff;
delete pf;
delete df;
96 if (!constraints_converged) {
98 if (num_unstable_constraints <= min_mechanical_constraints) converged=
true;
109 if(count>=max_nonlinear_iterations){
110 _printf0_(
" maximum number of nonlinear iterations (" << max_nonlinear_iterations <<
") exceeded\n");
129 delete Kff;
delete pf;
delete df;
delete Kfs;
◆ solutionsequence_newton()
void solutionsequence_newton |
( |
FemModel * |
femmodel | ) |
|
Definition at line 11 of file solutionsequence_newton.cpp.
31 int max_nonlinear_iterations;
55 delete old_ug;old_ug=ug;
56 delete old_uf;old_uf=uf;
59 if(count==1 || newton==2){
68 delete old_ug;old_ug=ug;
69 delete old_uf;old_uf=uf;
86 uf->
AXPY(duf, 1.0);
delete duf;
91 convergence(&converged,Kff,pf,uf,old_uf,eps_res,eps_rel,eps_abs);
92 delete Kff;
delete pf;
96 if(count>=max_nonlinear_iterations){
97 _printf0_(
" maximum number of Newton iterations (" << max_nonlinear_iterations <<
") exceeded\n");
◆ solutionsequence_fct()
void solutionsequence_fct |
( |
FemModel * |
femmodel | ) |
|
Definition at line 388 of file solutionsequence_fct.cpp.
392 int configuration_type,analysis_type;
409 switch(analysis_type){
421 default:
_error_(
"analysis type " <<
EnumToStringx(analysis_type) <<
" not supported for FCT\n");
434 Mat K_petsc = K->pmatrix->matrix;
435 Mat Mc_petsc = Mc->pmatrix->matrix;
436 Vec p_petsc = p->pvector->vector;
445 VecDuplicate(uf->pvector->vector,&Ml_petsc);
446 MatGetRowSum(Mc_petsc,Ml_petsc);
449 CreateDMatrix(&D_petsc,K_petsc);
452 CreateLHS(&LHS,&dmax,K_petsc,D_petsc,Ml_petsc,theta,deltat,
femmodel,configuration_type);
455 CreateRHS(&RHS,K_petsc,D_petsc,Ml_petsc,p_petsc,uf->pvector->vector,theta,deltat,dmax,
femmodel,configuration_type);
467 RichardsonUdot(&udot,u,Ml_petsc,K_petsc,Mc_petsc);
485 CreateRis(&Ri_plus_serial,&Ri_minus_serial,Mc_petsc,D_petsc,ml_serial,u,u_serial,udot_serial,ulmin,ulmax,deltat);
486 CreateFbar(&Fbar,Ri_plus_serial,Ri_minus_serial,Mc_petsc,D_petsc,udot_serial,u_serial,u);
487 xDelete<IssmDouble>(Ri_plus_serial);
488 xDelete<IssmDouble>(Ri_minus_serial);
489 xDelete<IssmDouble>(ulmin);
490 xDelete<IssmDouble>(ulmax);
495 xDelete<IssmDouble>(udot_serial);
496 xDelete<IssmDouble>(u_serial);
497 xDelete<IssmDouble>(ml_serial);
500 UpdateSolution(u,udot,Ml_petsc,Fbar,deltat);
512 _error_(
"PETSc needs to be installed");
◆ solutionsequence_FScoupling_nonlinear()
void solutionsequence_FScoupling_nonlinear |
( |
FemModel * |
femmodel, |
|
|
bool |
conserve_loads |
|
) |
| |
Definition at line 11 of file solutionsequence_stokescoupling_nonlinear.cpp.
32 int min_mechanical_constraints;
33 int max_nonlinear_iterations;
62 delete old_uf_horiz; old_uf_horiz=uf_horiz;
67 Reduceloadx(pf_horiz, Kfs_horiz, ys);
delete Kfs_horiz;
74 convergence(&converged,Kff_horiz,pf_horiz,uf_horiz,old_uf_horiz,eps_res,eps_rel,eps_abs);
delete Kff_horiz;
delete pf_horiz;
delete df_horiz;
82 Reduceloadx(pf_vert, Kfs_vert, ys);
delete Kfs_vert;
84 Solverx(&uf_vert, Kff_vert, pf_vert, NULL, df_vert,
femmodel->
parameters);
delete Kff_vert;
delete pf_vert;
delete df_vert;
94 if(converged==
true)
break;
95 if(count>=max_nonlinear_iterations){
96 _printf0_(
" maximum number of iterations (" << max_nonlinear_iterations <<
") exceeded\n");
◆ solutionsequence_linear()
void solutionsequence_linear |
( |
FemModel * |
femmodel | ) |
|
◆ solutionsequence_la()
void solutionsequence_la |
( |
FemModel * |
femmodel | ) |
|
Definition at line 10 of file solutionsequence_la.cpp.
23 int max_nonlinear_iterations;
24 bool vel_converged =
false;
25 bool pressure_converged =
false;
53 delete pug_old;pug_old=pug;
54 pressure_converged=
false; vel_converged=
false;
58 delete vel_old_local;vel_old_local=vel;
69 delete Kff;
delete pf;
delete df;
79 if (xIsNan<IssmDouble>(ndu) || xIsNan<IssmDouble>(nu))
_error_(
"convergence criterion is NaN!");
81 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" < " << eps_rel*100 <<
" %\n");
85 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" > " << eps_rel*100 <<
" %\n");
87 if(count_local>=max_nonlinear_iterations){
88 _printf0_(
" maximum number of nonlinear iterations (" << max_nonlinear_iterations <<
") exceeded\n");
103 delete Kff;
delete pf;
delete df;
114 if (xIsNan<IssmDouble>(ndu) || xIsNan<IssmDouble>(nu))
_error_(
"convergence criterion is NaN!");
115 if((ndu/nu)<eps_rel){
116 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" < " << eps_rel*100 <<
" %\n");
120 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" > " << eps_rel*100 <<
" %\n");
126 if (xIsNan<IssmDouble>(ndp) || xIsNan<IssmDouble>(np))
_error_(
"convergence criterion is NaN!");
127 if((ndp/np)<eps_rel){
128 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(dp)/norm(p)" << ndp/np*100 <<
" < " << eps_rel*100 <<
" %\n");
129 pressure_converged=
true;
132 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(dp/)/norm(p)" << ndp/np*100 <<
" > " << eps_rel*100 <<
" %\n");
133 pressure_converged=
false;
136 if(pressure_converged && vel_converged)
break;
137 if(count>=max_nonlinear_iterations){
138 _printf0_(
" maximum number of nonlinear iterations (" << max_nonlinear_iterations <<
") exceeded\n");
149 delete vel_old_local;
150 delete stressanalysis;
151 delete pressureanalysis;
◆ solutionsequence_la_theta()
void solutionsequence_la_theta |
( |
FemModel * |
femmodel | ) |
|
Definition at line 10 of file solutionsequence_la_theta.cpp.
22 int max_nonlinear_iterations;
48 delete ug_old;ug_old=ug;
49 delete vx_old;vx_old=vx;
65 delete Kff;
delete pf;
delete df;
83 if (xIsNan<IssmDouble>(ndu) || xIsNan<IssmDouble>(nu))
_error_(
"convergence criterion is NaN!");
85 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" < " << eps_rel*100 <<
" %\n");
89 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" > " << eps_rel*100 <<
" %\n");
92 if(count>=max_nonlinear_iterations){
93 _printf0_(
" maximum number of nonlinear iterations (" << max_nonlinear_iterations <<
") exceeded\n");
◆ solutionsequence_adjoint_linear()
void solutionsequence_adjoint_linear |
( |
FemModel * |
femmodel | ) |
|
◆ solutionsequence_schurcg()
void solutionsequence_schurcg |
( |
FemModel * |
femmodel | ) |
|
◆ convergence()
Definition at line 9 of file convergence.cpp.
37 KUold=uf->
Duplicate(); Kff->MatMult(old_uf,KUold);
42 if (xIsNan<IssmDouble>(res)){
43 _printf0_(
"norm nf = " << nF <<
"f and norm kuold = " << nKUoldF <<
"f\n");
44 _error_(
"mechanical equilibrium convergence criterion is NaN!");
53 if(
VerboseConvergence())
_printf0_(setw(50)<<left<<
" mechanical equilibrium convergence criterion"<<res*100<<
" < "<<eps_res*100<<
" %\n");
57 if(
VerboseConvergence())
_printf0_(setw(50)<<left<<
" mechanical equilibrium convergence criterion"<<res*100<<
" > "<<eps_res*100<<
" %\n");
68 if (xIsNan<IssmDouble>(ndu) || xIsNan<IssmDouble>(nu))
_error_(
"convergence criterion is NaN!");
74 if (!xIsNan<IssmDouble>(eps_rel)){
76 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" < " << eps_rel*100 <<
" %\n");
79 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" > " << eps_rel*100 <<
" %\n");
83 else _printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" %\n");
93 if (xIsNan<IssmDouble>(ndu) || xIsNan<IssmDouble>(nu))
_error_(
"convergence criterion is NaN!");
99 if (!xIsNan<IssmDouble>(eps_abs)){
100 if ((nduinf)<eps_abs){
108 else _printf0_(setw(50) << left <<
" Convergence criterion: max(du)" << nduinf <<
"\n");
113 *pconverged=converged;
void Mergesolutionfromftogx(Vector< IssmDouble > **pug, Vector< IssmDouble > *uf, Vector< IssmDouble > *ys, Nodes *nodes, Parameters *parameters, bool flag_ys0)
void ConstraintsStatex(int *pconverged, int *pnum_unstable_constraints, FemModel *femmodel)
static void UpdateBasalConstraints(FemModel *femmodel)
@ MasstransportAnalysisEnum
#define _printf0_(StreamArgs)
@ StressbalanceAnalysisEnum
bool VerboseConvergence(void)
void ResetZigzagCounterx(FemModel *femmodel)
static ISSM_MPI_Comm GetComm(void)
@ TimesteppingTimeStepEnum
@ DamageEvolutionAnalysisEnum
@ ThermalIsdynamicbasalspcEnum
@ HydrologydcIsefficientlayerEnum
void GetVectorFromInputsx(IssmDouble **pvector, int *pvector_size, FemModel *femmodel, int name)
@ StressbalanceConvergenceNumStepsEnum
void UpdateSheetThickness(FemModel *femmodel)
static void ComputeBasalMeltingrate(FemModel *femmodel)
void Solverx(Vector< IssmDouble > **puf, Matrix< IssmDouble > *Kff, Vector< IssmDouble > *pf, Vector< IssmDouble > *uf0, Vector< IssmDouble > *df, Parameters *parameters)
Vector< doubletype > * Duplicate(void)
void MatMult(Vector< doubletype > *X, Vector< doubletype > *AX)
void AllocateSystemMatricesx(Matrix< IssmDouble > **pKff, Matrix< IssmDouble > **pKfs, Vector< IssmDouble > **pdf, Vector< IssmDouble > **ppf, FemModel *femmodel)
@ ThermalPenaltyThresholdEnum
@ StressbalanceRiftPenaltyThresholdEnum
@ AugmentedLagrangianThetaEnum
void solutionsequence_linear(FemModel *femmodel)
@ StressbalanceMaxiterEnum
void SolverxPetsc(Vec *puf, Mat Kff, Vec pf, Vec uf0, Vec df, Parameters *parameters)
@ HydrologyDCInefficientAnalysisEnum
doubletype Norm(NormMode norm_type)
void Scale(doubletype scale_factor)
void InitZigZagCounter(FemModel *femmodel)
@ UzawaPressureAnalysisEnum
@ StressbalanceIsnewtonEnum
void SetParam(bool boolean, int enum_type)
void AXPY(Vector *X, doubletype a)
void MassMatrix(Matrix< IssmDouble > **pMff, FemModel *femmodel)
@ HydrologySedimentKmaxEnum
void HydrologyEPLupdateDomainx(IssmDouble *pEplcount)
void UpdateConstraintsx(void)
void ElementizeIdsMask(FemModel *femmodel)
int VecToMPISerial(double **pgathered_vector, Vec vector, ISSM_MPI_Comm comm, bool broadcast=true)
void GetInputLocalMinMaxOnNodesx(IssmDouble **pmin, IssmDouble **pmax, IssmDouble *ug)
const char * EnumToStringx(int enum_in)
@ StressbalanceAbstolEnum
void CreateJacobianMatrixx(Matrix< IssmDouble > **pJff, FemModel *femmodel, IssmDouble kmax)
@ StressbalanceReltolEnum
void ComputeEPLThickness(FemModel *femmodel)
void UpdateChannelCrossSection(FemModel *femmodel)
int AddResult(ExternalResult *result)
void FctKMatrix(Matrix< IssmDouble > **pKff, Matrix< IssmDouble > **pKfs, FemModel *femmodel)
void UpdateConstraintsL2ProjectionEPLx(IssmDouble *pL2count)
void Stop(int tagenum, bool dontmpisync=true)
@ HydrologyDCEfficientAnalysisEnum
void Reducevectorgtofx(Vector< IssmDouble > **puf, Vector< IssmDouble > *ug, Nodes *nodes, Parameters *parameters)
void InputUpdateFromSolutionFSXTH_tau(Elements *elements, Parameters *parameters)
void FctKMatrix(Matrix< IssmDouble > **pKff, Matrix< IssmDouble > **pKfs, FemModel *femmodel)
void Reduceloadx(Vector< IssmDouble > *pf, Matrix< IssmDouble > *Kfs, Vector< IssmDouble > *y_s, bool flag_ys0)
Declaration of Loads class.
void InputUpdateFromSolutionFSXTH_d(Elements *elements, Parameters *parameters)
@ L2ProjectionEPLAnalysisEnum
void AYPX(Vector *X, doubletype a)
void InitializeXTH(Elements *elements, Parameters *parameters)
@ StressbalanceVerticalAnalysisEnum
#define _error_(StreamArgs)
void Start(int tagenum, bool dontmpisync=true)
@ StressbalanceRestolEnum
bool VerboseSolution(void)
void ResetCounter(FemModel *femmodel)
void SetCurrentConfiguration(int configuration_type)
void FindParam(bool *pinteger, int enum_type)
void ResetConstraintsx(FemModel *femmodel)
@ AugmentedLagrangianREnum
void MassMatrix(Matrix< IssmDouble > **pMff, FemModel *femmodel)
void ElementizeEplMask(FemModel *femmodel)
void CreateNodalConstraintsx(Vector< IssmDouble > **pys, Nodes *nodes)
void FctPVector(Vector< IssmDouble > **ppf, FemModel *femmodel)
void SystemMatricesx(Matrix< IssmDouble > **pKff, Matrix< IssmDouble > **pKfs, Vector< IssmDouble > **ppf, Vector< IssmDouble > **pdf, IssmDouble *pkmax, FemModel *femmodel, bool isAllocated)
void convergence(bool *pconverged, Matrix< IssmDouble > *Kff, Vector< IssmDouble > *pf, Vector< IssmDouble > *uf, Vector< IssmDouble > *old_uf, IssmDouble eps_res, IssmDouble eps_rel, IssmDouble eps_abs)
void Set(doubletype value)