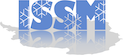 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
9 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
13 #include "../toolkits/toolkits.h"
25 this->
used[i] =
false;
66 void Profiler::Marshall(
char** pmarshalled_data,
int* pmarshalled_data_size,
int marshall_direction){
69 bool* bpointer = NULL;
72 pointer = &this->
time[0];
74 pointer = &this->
flops[0];
76 pointer = &this->
memory[0];
92 if(this->
running[tag])
_error_(
"Tag "<<tag<<
" has not been stopped");
94 return this->
flops[tag];
101 if(this->
running[tag])
_error_(
"Tag "<<tag<<
" has not been stopped");
104 return this->
time[tag];
106 return this->
time[tag]/CLOCKS_PER_SEC;
113 return int((reCast<int,IssmPDouble>(delta))/3600);
120 return int(
int(reCast<int,IssmPDouble>(delta))%3600/60);
126 return int(reCast<int,IssmPDouble>(delta)%60);
134 if(this->
running[tag])
_error_(
"Tag "<<tag<<
" has not been stopped");
144 if(this->
running[tag])
_error_(
"Tag "<<tag<<
" is already running");
163 PetscMemoryGetCurrentUsage(&m);
177 this->
used[tag] =
true;
203 PetscMemoryGetCurrentUsage(&m);
223 return this->
used[tag];
int TotalTimeModSec(int tag)
IssmPDouble memory_start[MAXPROFSIZE]
IssmPDouble Memory(int tag)
IssmPDouble TotalFlops(int tag)
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
static ISSM_MPI_Comm GetComm(void)
IssmPDouble time_start[MAXPROFSIZE]
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
IssmPDouble memory[MAXPROFSIZE]
#define MARSHALLING_DYNAMIC(FIELD, TYPE, SIZE)
IssmPDouble time[MAXPROFSIZE]
IssmPDouble flops[MAXPROFSIZE]
void Stop(int tagenum, bool dontmpisync=true)
#define _error_(StreamArgs)
: header file for node object
void Start(int tagenum, bool dontmpisync=true)
IssmPDouble flops_start[MAXPROFSIZE]
int TotalTimeModHour(int tag)
double ISSM_MPI_Wtime(void)
bool running[MAXPROFSIZE]
int TotalTimeModMin(int tag)
int ISSM_MPI_Barrier(ISSM_MPI_Comm comm)
IssmPDouble TotalTime(int tag)