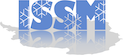 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
14 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
17 #include "../../shared/Enum/Enum.h"
18 #include "../petsc/petscincludes.h"
19 #include "../issm/issmtoolkit.h"
24 template <
class doubletype>
class Vector;
26 template <
class doubletype>
57 Matrix(
int m,
int n,
int M,
int N,
int* d_nnz,
int* o_nnz){
63 this->pmatrix=
new PetscMat(m,n,M,N,d_nnz,o_nnz);
78 this->pmatrix=
new PetscMat(M,N,sparsity);
92 this->pmatrix=
new PetscMat(serial_mat,M,N,sparsity);
101 Matrix(
int M,
int N,
int connectivity,
int numberofdofspernode){
107 this->pmatrix=
new PetscMat(M,N,connectivity,numberofdofspernode);
120 delete this->pmatrix;
138 if (strcmp(toolkittype,
"petsc")==0){
142 _error_(
"cannot create petsc matrix without PETSC compiled!");
145 else if(strcmp(toolkittype,
"issm")==0){
150 _error_(
"unknow toolkit type ");
154 xDelete<char>(toolkittype);
164 this->pmatrix->
Echo();
168 this->imatrix->
Echo();
203 norm=this->pmatrix->
Norm(norm_type);
207 norm=this->imatrix->
Norm(norm_type);
243 this->pmatrix->
MatMult(X->pvector,AX->pvector);
258 output->pmatrix=this->pmatrix->
Duplicate();
270 doubletype* output=NULL;
288 this->pmatrix->
SetValues(m,idxm,n,idxn,values,mode);
292 this->imatrix->
SetValues(m,idxm,n,idxn,values,mode);
300 this->pmatrix->
Convert(newtype);
304 this->imatrix->
Convert(newtype);
325 #endif //#ifndef _MATRIX_H_
void AllocationInfo(void)
Matrix(int M, int N, double sparsity)
Matrix(IssmPDouble *serial_mat, int M, int N, IssmPDouble sparsity)
doubletype Norm(NormMode mode)
void MatMult(Vector< doubletype > *X, Vector< doubletype > *AX)
void SetValues(int m, int *idxm, int n, int *idxn, doubletype *values, InsMode mode)
void GetLocalSize(int *pM, int *pN)
void MatMult(PetscVec *X, PetscVec *AX)
IssmMat< doubletype > * Duplicate(void)
void SetValues(int m, int *idxm, int n, int *idxn, IssmDouble *values, InsMode mode)
IssmDouble * ToSerial(void)
Matrix(int M, int N, int connectivity, int numberofdofspernode)
void GetLocalSize(int *pM, int *pN)
void AllocationInfo(void)
IssmDouble Norm(NormMode norm_type)
void InitCheckAndSetType(void)
doubletype * ToSerial(void)
void SetValues(int m, int *idxm, int n, int *idxn, IssmDouble *values, InsMode mode)
IssmDouble Norm(NormMode norm_type)
Matrix(int m, int n, int M, int N, int *d_nnz, int *o_nnz)
Matrix< doubletype > * Duplicate(void)
#define _error_(StreamArgs)
IssmVec< doubletype > * ivector
void MatMult(IssmVec< doubletype > *X, IssmVec< doubletype > *AX)
void Convert(MatrixType type)
void GetSize(int *pM, int *pN)
IssmMat< doubletype > * imatrix
void Convert(MatrixType newtype)
doubletype * ToSerial(void)
void GetSize(int *pM, int *pN)
void Convert(MatrixType type)
void GetLocalSize(int *pM, int *pN)
void GetSize(int *pM, int *pN)
PetscMat * Duplicate(void)