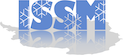 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
9 #include "../shared/shared.h"
10 #include "../datastructures/datastructures.h"
19 #define HYDROLOGYCORE 6
20 #define STRESSBALANCECORE 7
22 #define MOVINGFRONTCORE 9
23 #define MASSTRANSPORTCORE 10
25 #define GROUNDINGLINECORE 12
30 #define MAXPROFSIZE 17
54 void Marshall(
char** pmarshalled_data,
int* pmarshalled_data_size,
int marshall_direction);
64 void Start(
int tagenum,
bool dontmpisync=
true);
65 void Stop(
int tagenum,
bool dontmpisync=
true);
66 bool Used(
int tagenum);
int TotalTimeModSec(int tag)
IssmPDouble memory_start[MAXPROFSIZE]
IssmPDouble Memory(int tag)
IssmPDouble TotalFlops(int tag)
IssmPDouble time_start[MAXPROFSIZE]
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
IssmPDouble memory[MAXPROFSIZE]
IssmPDouble time[MAXPROFSIZE]
IssmPDouble flops[MAXPROFSIZE]
void Stop(int tagenum, bool dontmpisync=true)
void Start(int tagenum, bool dontmpisync=true)
IssmPDouble flops_start[MAXPROFSIZE]
int TotalTimeModHour(int tag)
bool running[MAXPROFSIZE]
int TotalTimeModMin(int tag)
IssmPDouble TotalTime(int tag)