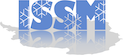 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
14 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
17 #include "../../shared/Enum/Enum.h"
18 #include "../petsc/petscincludes.h"
19 #include "../issm/issmtoolkit.h"
24 template <
class doubletype>
41 Vector(
int M,
bool fromlocalsize=
false){
47 this->pvector=
new PetscVec(M,fromlocalsize);
66 Vector(doubletype* serial_vec,
int M){
72 this->pvector=
new PetscVec(serial_vec,M);
93 this->pvector=
new PetscVec(petsc_vector);
110 if(strcmp(toolkittype,
"petsc")==0){
114 _error_(
"cannot create petsc vector without PETSC compiled!");
117 else if(strcmp(toolkittype,
"issm")==0){
122 _error_(
"unknow toolkit type ");
126 xDelete<char>(toolkittype);
135 this->pvector->
Echo();
138 else this->ivector->
Echo();
156 this->pvector->
SetValues(ssize,list,values,mode);
159 else this->ivector->
SetValues(ssize,list,values,mode);
167 this->pvector->
SetValue(dof,value,mode);
170 else this->ivector->
SetValue(dof,value,mode);
178 this->pvector->
GetValue(pvalue,dof);
181 else this->ivector->
GetValue(pvalue,dof);
192 else this->ivector->
GetSize(pM);
223 this->pvector->GetLocalVector(pvector,pindices);
238 output->pvector=this->pvector->
Duplicate();
249 this->pvector->
Set(value);
252 else this->ivector->
Set(value);
260 this->pvector->
AXPY(X->pvector,a);
271 this->pvector->
AYPX(X->pvector,a);
279 doubletype* vec_serial=NULL;
295 doubletype* vec_serial=NULL;
313 this->pvector->
Shift(shift);
316 else this->ivector->
Shift(shift);
323 this->pvector->
Copy(to->pvector);
338 else _error_(
"operation not supported yet");
348 norm=this->pvector->
Norm(norm_type);
351 else norm=this->ivector->
Norm(norm_type);
359 this->pvector->
Scale(scale_factor);
362 else this->ivector->
Scale(scale_factor);
371 dot=this->pvector->
Dot(vector->pvector);
389 #endif //#ifndef _VECTOR_H_
void AYPX(PetscVec *X, IssmDouble a)
void AYPX(IssmVec *X, doubletype a)
void SetValues(int ssize, int *list, IssmDouble *values, InsMode mode)
void SetValue(int dof, doubletype value, InsMode mode)
void Shift(doubletype shift)
void GetValue(doubletype *pvalue, int dof)
void GetLocalSize(int *pM)
void GetLocalVector(doubletype **pvector, int **pindices)
Vector< doubletype > * Duplicate(void)
void GetValue(IssmDouble *pvalue, int dof)
IssmDouble Dot(PetscVec *vector)
void SetValues(int ssize, int *list, doubletype *values, InsMode mode)
void Scale(IssmDouble scale_factor)
Vector(doubletype *serial_vec, int M)
void Shift(IssmDouble shift)
doubletype Norm(NormMode norm_type)
void GetLocalSize(int *pM)
doubletype Norm(NormMode mode)
void Scale(doubletype scale_factor)
void Set(doubletype value)
void AXPY(Vector *X, doubletype a)
void Scale(doubletype scale_factor)
void GetLocalSize(int *pM)
void SetValues(int ssize, int *list, doubletype *values, InsMode mode)
IssmDouble * ToMPISerial0(void)
doubletype * ToMPISerial0(void)
doubletype Dot(IssmVec *input)
doubletype Dot(Vector *vector)
PetscVec * Duplicate(void)
void PointwiseDivide(IssmVec *x, IssmVec *y)
IssmDouble Norm(NormMode norm_type)
void AXPY(IssmVec *X, doubletype a)
void PointwiseDivide(PetscVec *x, PetscVec *y)
void AYPX(Vector *X, doubletype a)
void AXPY(PetscVec *X, IssmDouble a)
#define _error_(StreamArgs)
IssmVec< doubletype > * ivector
Vector(int M, bool fromlocalsize=false)
doubletype * ToMPISerial0(void)
IssmDouble * ToMPISerial(void)
void PointwiseDivide(Vector *x, Vector *y)
doubletype * ToMPISerial(void)
doubletype * ToMPISerial(void)
void Set(IssmDouble value)
IssmDouble max(IssmDouble a, IssmDouble b)
void Shift(doubletype shift)
void GetValue(doubletype *pvalue, int dof)
void SetValue(int dof, doubletype value, InsMode mode)
void Set(doubletype value)
void GetLocalVector(doubletype **pvector, int **pindices)
void SetValue(int dof, IssmDouble value, InsMode mode)
void InitCheckAndSetType(void)
IssmVec< doubletype > * Duplicate(void)