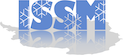 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
10 #include "../toolkits/toolkits.h"
23 #if defined(_HAVE_NEOPZ_) && !defined(_HAVE_AD_)
26 #if defined(_HAVE_BAMG_) && !defined(_HAVE_AD_)
60 #if defined(_HAVE_NEOPZ_) && !defined(_HAVE_AD_)
64 #if defined(_HAVE_BAMG_) && !defined(_HAVE_AD_)
80 void InitFromFiles(
char* rootpath,
char* inputfilename,
char* outputfilename,
char* petscfilename,
char* lockfilename,
char* restartfilename,
const int solution_type,
bool trace,
IssmPDouble* X=NULL);
81 void InitFromFids(
char* rootpath, FILE* IOMODEL, FILE* toolkitsoptionsfid,
int in_solution_type,
bool trace,
IssmPDouble* X=NULL);
82 void Marshall(
char** pmarshalled_data,
int* pmarshalled_data_size,
int marshall_direction);
142 void DakotaResponsesx(
double* d_responses,
char** responses_descriptors,
int numresponsedescriptors,
int d_numresponses);
164 #ifdef _HAVE_SEALEVELRISE_
183 #ifdef _HAVE_JAVASCRIPT_
185 void CleanUpJs(
char** poutput,
size_t* psize);
186 void InitFromBuffers(
char* buffer,
int buffersize,
char* toolkits,
int solution_type,
bool trace,
IssmPDouble* X=NULL);
190 #if !defined(_HAVE_AD_)
201 void GetInputs(
int* pnumP0inputs,
IssmDouble** pP0inputs,
int** pP0input_enums,
int** pP0input_interp,
int* pnumP1inputs,
IssmDouble** pP1inputs,
int** pP1input_enums,
int** pP1input_interp);
215 #if defined(_HAVE_BAMG_) && !defined(_HAVE_AD_)
216 void ReMeshBamg(
int* pnewnumberofvertices,
int* pnewnumberofelements,
IssmDouble** pnewx,
IssmDouble** pnewy,
IssmDouble** pnewz,
int** pnewelementslist);
217 void InitializeAdaptiveRefinementBamg(
void);
218 void GethmaxVerticesFromZeroLevelSetDistance(
IssmDouble* hmaxvertices,
int levelset_type);
219 void GethmaxVerticesFromEstimators(
IssmDouble* hmaxvertices,
int errorestimator_type);
220 void GetVerticeDistanceToZeroLevelSet(
IssmDouble** pverticedistance,
int leveset_type);
223 #if defined(_HAVE_NEOPZ_) && !defined(_HAVE_AD_)
224 void ReMeshNeopz(
int* pnewnumberofvertices,
int* pnewnumberofelements,
IssmDouble** pnewx,
IssmDouble** pnewy,
IssmDouble** pnewz,
int** pnewelementslist);
225 void InitializeAdaptiveRefinementNeopz(
void);
226 void GetElementDistanceToZeroLevelSet(
IssmDouble** pelementdistance,
int levelset_type);
227 void SetRefPatterns(
void);
void TotalCalvingFluxLevelsetx(IssmDouble *pGbmb, bool scaled)
void MaxAbsVzx(IssmDouble *presponse)
Declaration of Vertices class.
void CreateElements(int newnumberofelements, int elementswidth, int *newelementslist, bool *my_elements, Elements *elements)
void ElementOperationx(void(Element::*function)(void))
void InitFromFiles(char *rootpath, char *inputfilename, char *outputfilename, char *petscfilename, char *lockfilename, char *restartfilename, const int solution_type, bool trace, IssmPDouble *X=NULL)
void MaxVelx(IssmDouble *presponse)
Declaration of Nodes class.
void MaxAbsVyx(IssmDouble *presponse)
void GetLocalVectorWithClonesGset(IssmDouble **plocal_ug, Vector< IssmDouble > *ug)
void InputMakeDiscontinuous(int enum_in)
void MinVyx(IssmDouble *presponse)
void InitTransientInputx(int *transientinput_enum, int numoutputs)
void BalancethicknessMisfitx(IssmDouble *pV)
Declaration of Parameters class.
void EtaDiffx(IssmDouble *pJ)
Declaration of Constraints class.
void StrainRateeffectivex()
void CostFunctionx(IssmDouble *pJ, IssmDouble **pJlist, int *pn)
void GetMeshOnPartition(Vertices *femmodel_vertices, Elements *femmodel_elements, IssmDouble **px, IssmDouble **py, IssmDouble **pz, int **pelementslist, int **psidtoindex)
Declaration of Elements class.
void SolutionAnalysesList(int **panalyses, int *pnumanalyses, IoModel *iomodel, int solutiontype)
void CalvingMeltingFluxLevelsetx()
void TotalCalvingMeltingFluxLevelsetx(IssmDouble *pGbmb, bool scaled)
void AdjustBaseThicknessAndMask(void)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
void OutputControlsx(Results **presults)
void CalvingFluxLevelsetx()
void TimeAdaptx(IssmDouble *pdt)
Constraints ** constraints_list
void SmoothedDeviatoricStressTensor(IssmDouble **ptauxx, IssmDouble **ptauyy, IssmDouble **ptauxy)
void TotalSmbx(IssmDouble *pSmb, bool scaled)
void RequestedDependentsx(void)
void GetLocalVectorWithClonesVertices(IssmDouble **plocal_vector, Vector< IssmDouble > *vector)
void InitFromFids(char *rootpath, FILE *IOMODEL, FILE *toolkitsoptionsfid, int in_solution_type, bool trace, IssmPDouble *X=NULL)
void MeanGroundedIceLevelSet(IssmDouble **pmasklevelset)
void Divergencex(IssmDouble *pdiv)
void CalvingRateLevermannx()
void OmegaAbsGradientx(IssmDouble *pJ)
void MaxAbsVxx(IssmDouble *presponse)
void SyncLocalVectorWithClonesVertices(IssmDouble *local_vector)
void HydrologyIDSupdateDomainx(IssmDouble *pIDScount)
void HydrologyEPLupdateDomainx(IssmDouble *pEplcount)
Declaration of Materials class.
void StressIntensityFactorx()
void UpdateConstraintsx(void)
void IceVolumex(IssmDouble *pV, bool scaled)
void MinVelx(IssmDouble *presponse)
void GetInputLocalMinMaxOnNodesx(IssmDouble **pmin, IssmDouble **pmax, IssmDouble *ug)
void MinVxx(IssmDouble *presponse)
void CalvingRateVonmisesx()
void TotalFloatingBmbx(IssmDouble *pFbmb, bool scaled)
void ZZErrorEstimator(IssmDouble **pelementerror)
void AverageTransientInputx(int *transientinput_enum, int *averagedinput_enum, IssmDouble init_time, IssmDouble end_time, int numoutputs, int averaging_method)
void MaxVyx(IssmDouble *presponse)
void MaxVxx(IssmDouble *presponse)
void ElementResponsex(IssmDouble *presponse, int response_enum)
Constraints * constraints
void UpdateConstraintsExtrudeFromBasex()
void GroundinglineMassFluxx(IssmDouble *presponse, bool scaled)
void GetLocalVectorWithClonesNodes(IssmDouble **plocal_vector, Vector< IssmDouble > *vector)
void UpdateConstraintsL2ProjectionEPLx(IssmDouble *pL2count)
void BedrockFromMismipPlus(void)
void StackTransientInputx(int *input_enum, int *transientinput_enum, IssmDouble hydrotime, int numoutputs)
void Responsex(IssmDouble *presponse, int response_descriptor_enum)
void RignotMeltParameterizationx()
void TotalGroundedBmbx(IssmDouble *pGbmb, bool scaled)
void GetZeroLevelSetPoints(IssmDouble **pzerolevelset_points, int &numberofpoints, int levelset_type)
void IceMassx(IssmDouble *pV, bool scaled)
void ThicknessPositivex(IssmDouble *pJ)
void IcefrontMassFluxx(IssmDouble *presponse, bool scaled)
void MinVzx(IssmDouble *presponse)
void StrainRateperpendicularx()
void SyncLocalVectorWithClonesVerticesAdd(IssmDouble *local_vector)
void IceVolumeAboveFloatationx(IssmDouble *pV, bool scaled)
Declaration of Loads class.
void CreateMaterials(int newnumberofelements, bool *my_elements, Materials *materials)
void GetInputs(int *pnumP0inputs, IssmDouble **pP0inputs, int **pP0input_enums, int **pP0input_interp, int *pnumP1inputs, IssmDouble **pP1inputs, int **pP1input_enums, int **pP1input_interp)
void UpdateElements(int newnumberofelements, int *newelementslist, bool *my_elements, int analysis_counter, Elements *newelements)
void MassFluxx(IssmDouble *presponse)
void CreateConstraints(Vertices *newfemmodel_vertices, int analysis_enum, Constraints *newfemmodel_constraints)
void MaxVzx(IssmDouble *presponse)
void SurfaceAbsMisfitx(IssmDouble *pJ)
void RequestedOutputsx(Results **presults, char **requested_outputs, int numoutputs, bool save_results=true)
void SetCurrentConfiguration(int configuration_type)
void GetElementCenterCoordinates(IssmDouble **pxc, IssmDouble **pyc)
void FloatingAreax(IssmDouble *pV, bool scaled)
void InterpolateInputs(Vertices *newfemmodel_vertices, Elements *newfemmodel_elements, Inputs2 *new_inputs)
void StrainRateparallelx()
void IcefrontMassFluxLevelsetx(IssmDouble *presponse, bool scaled)
void ThicknessAbsGradientx(IssmDouble *pJ)
void ThicknessZZErrorEstimator(IssmDouble **pelementerror)
Declaration of DataSet class.
void SetMesh(int **elementslist, IssmDouble **x, IssmDouble **y, int *numberofvertices, int *numberofelements)
void DistanceToFieldValue(int fieldenum, IssmDouble fieldvalue, int distanceenum)
void SmoothedGradThickness(IssmDouble **pdHdx, IssmDouble **pdHdy)
void WriteMeshInResults(void)
Declaration of Results class.
void UpdateConstraintsExtrudeFromTopx()
void MaxDivergencex(IssmDouble *pdiv)
void GetMesh(Vertices *femmodel_vertices, Elements *femmodel_elements, IssmDouble **px, IssmDouble **py, int **pelementslist)
int UpdateVertexPositionsx(void)
void WriteErrorEstimatorsInResults(void)
FemModel(int argc, char **argv, ISSM_MPI_Comm comm_init, bool trace=false)
void GroundedAreax(IssmDouble *pV, bool scaled)