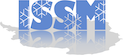 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
10 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
17 #include "../../shared/io/Comm/IssmComm.h"
18 #include "../../shared/Numerics/recast.h"
19 #include "../../shared/Enum/EnumDefinitions.h"
20 #include "../../shared/Exceptions/exceptions.h"
21 #include "../../shared/MemOps/MemOps.h"
22 #include "../../shared/io/Marshalling/Marshalling.h"
33 this->numpenalties = 0;
48 output->
sorted=this->sorted;
51 for(vector<Object*>::iterator obj=this->objects.begin() ; obj < this->objects.end(); obj++ ){
56 int objsize = this->numsorted;
59 if(this->sorted && objsize>0 && this->id_offsets){
61 xMemCpy<int>(output->
id_offsets,this->id_offsets,objsize);
63 if(this->sorted && objsize>0 && this->sorted_ids){
65 xMemCpy<int>(output->
sorted_ids,this->sorted_ids,objsize);
75 void Loads::Marshall(
char** pmarshalled_data,
int* pmarshalled_data_size,
int marshall_direction){
90 vector<Object*>::iterator object;
91 for(
object=objects.begin() ;
object < objects.end();
object++){
92 Load* load=xDynamicCast<Load*>(*
object);
93 load->
Configure(elements,loads,nodes,vertices,materials,parameters);
105 for(
int i=0;i<this->Size();i++){
106 Load* load=xDynamicCast<Load*>(this->GetObjectByOffset(i));
117 this->numpenalties = allcount;
120 this->numrifts= allcount;
126 if(this->numpenalties>0)
138 for(
int i=0;i<this->Size();i++){
139 Load* load=xDynamicCast<Load*>(this->GetObjectByOffset(i));
141 if(numnodes>
max)
max=numnodes;
155 localloads=this->Size();
161 return numberofloads;
166 vector<Object*>::iterator object;
169 for (
object=objects.begin() ;
object < objects.end();
object++ ){
171 load=xDynamicCast<Load*>((*
object));
180 vector<Object*>::iterator object;
183 for (
object=objects.begin() ;
object < objects.end();
object++ ){
184 load=xDynamicCast<Load*>(*
object);
Declaration of Vertices class.
Declaration of Nodes class.
int ISSM_MPI_Allreduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, ISSM_MPI_Comm comm)
int AddObject(Object *object)
Declaration of Parameters class.
#define MARSHALLING_ENUM(EN)
static ISSM_MPI_Comm GetComm(void)
Declaration of Elements class.
Declaration of Materials class.
virtual void Configure(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters)=0
virtual void SetCurrentConfiguration(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters)=0
abstract class for Load object This class is a place holder for the Icefront and the Penpair loads....
void Configure(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters)
#define MARSHALLING(FIELD)
virtual int GetNumberOfNodes(void)=0
int ISSM_MPI_Bcast(void *buffer, int count, ISSM_MPI_Datatype datatype, int root, ISSM_MPI_Comm comm)
virtual int ObjectEnum()=0
Declaration of Loads class.
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
virtual bool IsPenalty(void)=0
int ISSM_MPI_Reduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, int root, ISSM_MPI_Comm comm)
IssmDouble max(IssmDouble a, IssmDouble b)
void SetCurrentConfiguration(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters)
virtual void ResetHooks()=0
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)