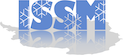 |
Ice Sheet System Model
4.18
Code documentation
|
: core of a non-linear solution, using fixed-point method
More...
Go to the source code of this file.
: core of a non-linear solution, using fixed-point method
Definition in file solutionsequence_nonlinear.cpp.
◆ solutionsequence_nonlinear()
void solutionsequence_nonlinear |
( |
FemModel * |
femmodel, |
|
|
bool |
conserve_loads |
|
) |
| |
Definition at line 11 of file solutionsequence_nonlinear.cpp.
23 Loads* savedloads=NULL;
25 int constraints_converged;
26 int num_unstable_constraints;
30 int min_mechanical_constraints;
31 int max_nonlinear_iterations;
32 int configuration_type;
68 delete old_uf;old_uf=uf;
83 convergence(&converged,Kff,pf,uf,old_uf,eps_res,eps_rel,eps_abs);
89 delete Kfs;
delete Kff;
delete pf;
delete df;
96 if (!constraints_converged) {
98 if (num_unstable_constraints <= min_mechanical_constraints) converged=
true;
109 if(count>=max_nonlinear_iterations){
110 _printf0_(
" maximum number of nonlinear iterations (" << max_nonlinear_iterations <<
") exceeded\n");
129 delete Kff;
delete pf;
delete df;
delete Kfs;
void Mergesolutionfromftogx(Vector< IssmDouble > **pug, Vector< IssmDouble > *uf, Vector< IssmDouble > *ys, Nodes *nodes, Parameters *parameters, bool flag_ys0)
void ConstraintsStatex(int *pconverged, int *pnum_unstable_constraints, FemModel *femmodel)
#define _printf0_(StreamArgs)
bool VerboseConvergence(void)
@ StressbalanceConvergenceNumStepsEnum
void Solverx(Vector< IssmDouble > **puf, Matrix< IssmDouble > *Kff, Vector< IssmDouble > *pf, Vector< IssmDouble > *uf0, Vector< IssmDouble > *df, Parameters *parameters)
void AllocateSystemMatricesx(Matrix< IssmDouble > **pKff, Matrix< IssmDouble > **pKfs, Vector< IssmDouble > **pdf, Vector< IssmDouble > **ppf, FemModel *femmodel)
@ StressbalanceRiftPenaltyThresholdEnum
@ StressbalanceMaxiterEnum
void UpdateConstraintsx(void)
@ StressbalanceAbstolEnum
@ StressbalanceReltolEnum
int AddResult(ExternalResult *result)
void Stop(int tagenum, bool dontmpisync=true)
void Reducevectorgtofx(Vector< IssmDouble > **puf, Vector< IssmDouble > *ug, Nodes *nodes, Parameters *parameters)
void Reduceloadx(Vector< IssmDouble > *pf, Matrix< IssmDouble > *Kfs, Vector< IssmDouble > *y_s, bool flag_ys0)
Declaration of Loads class.
void Start(int tagenum, bool dontmpisync=true)
@ StressbalanceRestolEnum
void FindParam(bool *pinteger, int enum_type)
void CreateNodalConstraintsx(Vector< IssmDouble > **pys, Nodes *nodes)
void SystemMatricesx(Matrix< IssmDouble > **pKff, Matrix< IssmDouble > **pKfs, Vector< IssmDouble > **ppf, Vector< IssmDouble > **pdf, IssmDouble *pkmax, FemModel *femmodel, bool isAllocated)
void convergence(bool *pconverged, Matrix< IssmDouble > *Kff, Vector< IssmDouble > *pf, Vector< IssmDouble > *uf, Vector< IssmDouble > *old_uf, IssmDouble eps_res, IssmDouble eps_rel, IssmDouble eps_abs)
void Set(doubletype value)