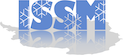 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the source code of this file.
◆ solutionsequence_newton()
void solutionsequence_newton |
( |
FemModel * |
femmodel | ) |
|
Definition at line 11 of file solutionsequence_newton.cpp.
31 int max_nonlinear_iterations;
55 delete old_ug;old_ug=ug;
56 delete old_uf;old_uf=uf;
59 if(count==1 || newton==2){
68 delete old_ug;old_ug=ug;
69 delete old_uf;old_uf=uf;
86 uf->
AXPY(duf, 1.0);
delete duf;
91 convergence(&converged,Kff,pf,uf,old_uf,eps_res,eps_rel,eps_abs);
92 delete Kff;
delete pf;
96 if(count>=max_nonlinear_iterations){
97 _printf0_(
" maximum number of Newton iterations (" << max_nonlinear_iterations <<
") exceeded\n");
void Mergesolutionfromftogx(Vector< IssmDouble > **pug, Vector< IssmDouble > *uf, Vector< IssmDouble > *ys, Nodes *nodes, Parameters *parameters, bool flag_ys0)
#define _printf0_(StreamArgs)
bool VerboseConvergence(void)
void Solverx(Vector< IssmDouble > **puf, Matrix< IssmDouble > *Kff, Vector< IssmDouble > *pf, Vector< IssmDouble > *uf0, Vector< IssmDouble > *df, Parameters *parameters)
Vector< doubletype > * Duplicate(void)
void MatMult(Vector< doubletype > *X, Vector< doubletype > *AX)
@ StressbalanceMaxiterEnum
void Scale(doubletype scale_factor)
@ StressbalanceIsnewtonEnum
void AXPY(Vector *X, doubletype a)
void UpdateConstraintsx(void)
@ StressbalanceAbstolEnum
void CreateJacobianMatrixx(Matrix< IssmDouble > **pJff, FemModel *femmodel, IssmDouble kmax)
@ StressbalanceReltolEnum
void Stop(int tagenum, bool dontmpisync=true)
void Reducevectorgtofx(Vector< IssmDouble > **puf, Vector< IssmDouble > *ug, Nodes *nodes, Parameters *parameters)
void Reduceloadx(Vector< IssmDouble > *pf, Matrix< IssmDouble > *Kfs, Vector< IssmDouble > *y_s, bool flag_ys0)
void Start(int tagenum, bool dontmpisync=true)
@ StressbalanceRestolEnum
void FindParam(bool *pinteger, int enum_type)
void CreateNodalConstraintsx(Vector< IssmDouble > **pys, Nodes *nodes)
void SystemMatricesx(Matrix< IssmDouble > **pKff, Matrix< IssmDouble > **pKfs, Vector< IssmDouble > **ppf, Vector< IssmDouble > **pdf, IssmDouble *pkmax, FemModel *femmodel, bool isAllocated)
void convergence(bool *pconverged, Matrix< IssmDouble > *Kff, Vector< IssmDouble > *pf, Vector< IssmDouble > *uf, Vector< IssmDouble > *old_uf, IssmDouble eps_res, IssmDouble eps_rel, IssmDouble eps_abs)