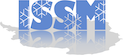 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
9 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
14 #include "../classes.h"
15 #include "../Inputs2/SegInput2.h"
16 #include "../Inputs2/TriaInput2.h"
17 #include "../../shared/shared.h"
74 for(i=0;i<nanalyses;i++){
88 else seg->
hnodes[i] = NULL;
109 unsigned int num_nodes = 3;
110 seg->
nodes = xNew<Node*>(num_nodes);
111 for(i=0;i<num_nodes;i++)
if(this->
nodes[i]) seg->
nodes[i]=this->
nodes[i];
else seg->
nodes[i] = NULL;
113 else seg->
nodes = NULL;
122 void Seg::Marshall(
char** pmarshalled_data,
int* pmarshalled_data_size,
int marshall_direction){
134 SegRef::Marshall(pmarshalled_data,pmarshalled_data_size,marshall_direction);
149 x1=xyz_list[0][0]; y1=xyz_list[0][1];
150 x2=xyz_list[1][0]; y2=xyz_list[1][1];
152 return sqrt((x2-x1)*(x2-x1) + (y2-y1)*(y2-y1));
162 int nrfrontnodes,index;
182 for(
int dir=0;dir<3;dir++){
183 xyz_front[dir]=xyz_list[3*index+dir];
186 *pxyz_front=xyz_front;
192 if(!input)
return input;
200 switch(interpolation){
203 indices[0] = this->
lid;
204 input->
Serve(numindices,&indices[0]);
208 for(
int i=0;i<numindices;i++) indices[i] =
vertices[i]->
lid;
209 input->
Serve(numindices,&indices[0]);
224 if(!input)
return input;
232 switch(interpolation){
235 indices[0] = this->
lid;
236 input->
Serve(numindices,&indices[0]);
240 for(
int i=0;i<3;i++) indices[i] =
vertices[i]->
lid;
241 input->
Serve(numindices,&indices[0]);
255 _error_(
"not implemented yet");
260 bool mainlyfloating =
true;
269 if(gl[0]==0.) gl[0]=gl[0]+epsilon;
270 if(gl[1]==0.) gl[1]=gl[1]+epsilon;
272 if(gl[0]>0 && gl[1]>0) phi=1;
273 else if(gl[0]<0 && gl[1]<0) phi=0;
274 else if(gl[0]<0 && gl[1]>0){
275 phi=1./(1.-gl[0]/gl[1]);
277 else if(gl[1]<0 && gl[0]>0){
278 phi=1./(1.-gl[1]/gl[0]);
281 if(phi>1 || phi<0)
_error_(
"Error. Problem with portion of grounded element: value should be between 0 and 1");
300 *pxyz_list = xyz_list;
317 for(
int iv=0;iv<
NUMVERTICES;iv++) pvalue[iv] = default_value;
333 for(
int iv=0;iv<numnodes;iv++){
339 for(
int iv=0;iv<numnodes;iv++) pvalue[iv] = default_value;
357 if(ls[i]<0.) nrice++;
358 if(nrice==1) isicefront=
true;
388 if(xyz_list_front[0] == xyz_list[0]){
391 else if(xyz_list_front[0] == xyz_list[3*1+0]){
395 _error_(
"front is not located on element edge");
434 if(xyz_list_front[0]>xyz_list[0])
439 xDelete<IssmDouble>(xyz_list);
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
void NormalSection(IssmDouble *normal, IssmDouble *xyz_list)
#define MARSHALLING_ENUM(EN)
void NodalFunctionsP2(IssmDouble *basis, Gauss *gauss)
void GetNodalFunctionsDerivatives(IssmDouble *dbasis, IssmDouble *xyz_list, GaussSeg *gauss, int finiteelement)
void NodalFunctionsDerivatives(IssmDouble *dbasis, IssmDouble *xyz_list, Gauss *gauss)
IssmDouble GetGroundedPortion(IssmDouble *xyz_list)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
void NodalFunctions(IssmDouble *basis, Gauss *gauss)
IssmDouble CharacteristicLength(void)
void NodalFunctionsP1(IssmDouble *basis, Gauss *gauss)
int GetNumberOfNodes(void)
#define MARSHALLING(FIELD)
void GetJacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, GaussSeg *gauss)
void GetIcefrontCoordinates(IssmDouble **pxyz_front, IssmDouble *xyz_list, int levelsetenum)
int NumberofNodes(int finiteelement)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
void GetInputListOnVertices(IssmDouble *pvalue, Input2 *input, IssmDouble default_value)
#define _error_(StreamArgs)
void JacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, Gauss *gauss)
void GetVerticesCoordinates(IssmDouble **pxyz_list)
Input2 * GetInput2(int enumtype)
void JacobianDeterminantSurface(IssmDouble *pJdet, IssmDouble *xyz_list, Gauss *gauss)
void GetInputListOnNodes(IssmDouble *pvalue, Input2 *input, IssmDouble default_value)
void MarshallElement(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction, int numanalyses)
void GaussNode(int finitelement, int iv)
void GetInputListOnVertices(IssmDouble *pvalue, int enumtype)
void GetNodalFunctions(IssmDouble *basis, GaussSeg *gauss, int finiteelement)
int GetNumberOfVertices(void)