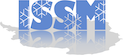 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
8 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
11 #include "../classes.h"
12 #include "../../shared/shared.h"
38 this->
Reset(interp_in);
65 this->
values = xNewZeroInit<IssmDouble>(this->
M*this->
N);
74 xMemCpy<IssmDouble>(output->
values,this->values,this->M*this->N);
143 this->
values[row] = value_in;
152 for(
int i=0;i<numindices;i++){
153 int row = indices[i];
156 this->
values[row] = values_in[i];
161 for(
int i=0;i<numindices;i++){
162 int row = indices[i];
165 this->
values[row] = values_in[i];
169 this->
Reset(interp_in);
170 for(
int i=0;i<numindices;i++){
171 int row = indices[i];
174 this->
values[row] = values_in[i];
190 this->
Reset(interp_in);
193 for(
int i=0;i<numindices;i++) this->
values[row*this->
N+i] = values_in[i];
202 for(
int i=0;i<numindices;i++){
203 int row = indices[i];
220 for(
int i=0;i<numindices;i++){
235 IssmDouble numnodesd = reCast<int,IssmDouble>(numnodes);
239 value = value/numnodesd;
250 for(
int i=1;i<numnodes;i++){
262 for(
int i=1;i<numnodes;i++){
274 for(
int i=1;i<numnodes;i++){
321 SegInput2* xseginput=xDynamicCast<SegInput2*>(xinput);
325 for(
int i=0;i<this->
M*this->
N;i++) this->
values[i] =
alpha*xseginput->
values[i] + this->values[i];
333 SegInput2* xseginput=xDynamicCast<SegInput2*>(xinput);
337 if(xseginput->
M!=this->M||xseginput->
N!=this->N)
_error_(
"Operation not permitted because the inputs have different sizes");
340 for(
int i=0;i<this->
M*this->
N;i++) this->
values[i] = xseginput->
values[i] * this->values[i];
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
void GetInputValue(IssmDouble *p, IssmDouble *plist, GaussSeg *gauss, int finiteelement)
#define MARSHALLING_DYNAMIC(FIELD, TYPE, SIZE)
const char * EnumToStringx(int enum_in)
IssmDouble alpha(IssmDouble x, IssmDouble y, IssmDouble z, int testid)
#define MARSHALLING(FIELD)
void GetInputDerivativeValue(IssmDouble *p, IssmDouble *plist, IssmDouble *xyz_list, GaussSeg *gauss, int finiteelement)
int NumberofNodes(int finiteelement)
virtual int ObjectEnum()=0
#define _error_(StreamArgs)
void printarray(IssmPDouble *array, int lines, int cols=1)
IssmDouble min(IssmDouble a, IssmDouble b)
IssmDouble max(IssmDouble a, IssmDouble b)