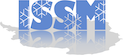 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
10 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
13 #include "../classes.h"
14 #include "../../shared/shared.h"
55 for(
int i=0;i<numnodes;i++) dpx += dbasis[0*numnodes+i]*plist[i];
77 for(
int i=0;i<numnodes;i++) value += basis[i]*plist[i];
99 if(*Jdet<0)
_error_(
"negative jacobian determinant!");
120 switch(finiteelement){
125 basis[0]=(1.-gauss->
coord1)/2.;
126 basis[1]=(1.+gauss->
coord1)/2.;
157 for(
int i=0;i<numnodes;i++){
158 dbasis[i] = Jinv*dbasis_ref[i];
168 switch(finiteelement){
183 dbasis[2] = -2.*gauss->
coord1;
193 switch(finiteelement){
void GetInputValue(IssmDouble *p, IssmDouble *plist, GaussSeg *gauss, int finiteelement)
void GetNodalFunctionsDerivatives(IssmDouble *dbasis, IssmDouble *xyz_list, GaussSeg *gauss, int finiteelement)
void GetNodalFunctionsDerivativesReference(IssmDouble *dbasis, GaussSeg *gauss, int finiteelement)
const char * EnumToStringx(int enum_in)
void GetJacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, GaussSeg *gauss)
void GetInputDerivativeValue(IssmDouble *p, IssmDouble *plist, IssmDouble *xyz_list, GaussSeg *gauss, int finiteelement)
int NumberofNodes(int finiteelement)
void GetJacobian(IssmDouble *J, IssmDouble *xyz_list, GaussSeg *gauss)
#define _error_(StreamArgs)
void GetJacobianInvert(IssmDouble *Jinv, IssmDouble *xyz_list, GaussSeg *gauss)
void GetNodalFunctions(IssmDouble *basis, GaussSeg *gauss, int finiteelement)