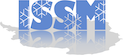 |
Ice Sheet System Model
4.18
Code documentation
|
#include <IoModel.h>
|
| ~IoModel () |
|
| IoModel () |
|
| IoModel (FILE *iomodel_handle, int solution_enum_in, bool trace, IssmPDouble *X) |
|
void | AddConstant (IoConstant *constant_in) |
|
void | AddConstantIndependent (IoConstant *constant_in) |
|
void | AddData (IoData *data_in) |
|
void | AddDataIndependent (IoData *data_in) |
|
void | FetchIndependentConstant (int *pXcount, IssmPDouble *X, const char *name) |
|
void | FetchIndependentData (int *pXcount, IssmPDouble *X, const char *name) |
|
void | FillIndependents (IssmDouble *xp) |
|
void | FindConstant (bool *pvalue, const char *constant_name) |
|
void | FindConstant (int *pvalue, const char *constant_name) |
|
void | FindConstant (IssmDouble *pvalue, const char *constant_name) |
|
void | FindConstant (char **pvalue, const char *constant_name) |
|
void | FindConstant (char ***pvalue, int *psize, const char *constant_name) |
|
int | NumIndependents () |
|
void | CheckFile (void) |
|
Param * | CopyConstantObject (const char *constant_name, int param_enum) |
|
IssmDouble * | Data (const char *data_name) |
|
void | DeclareIndependents (bool trace, IssmPDouble *X) |
|
void | DeleteData (int num,...) |
|
void | DeleteData (IssmDouble *vector, const char *data_name) |
|
void | DeleteData (char ***pstringarray, int numstrings, const char *data_name) |
|
void | FetchConstants (void) |
|
void | FetchData (bool *pboolean, const char *data_name) |
|
void | FetchData (int *pinteger, const char *data_name) |
|
void | FetchData (IssmDouble *pscalar, const char *data_name) |
|
void | FetchData (char **pstring, const char *data_name) |
|
void | FetchData (char ***pstrings, int *pnumstrings, const char *data_name) |
|
void | FetchData (int **pmatrix, int *pM, int *pN, const char *data_name) |
|
void | FetchData (IssmDouble **pscalarmatrix, int *pM, int *pN, const char *data_name) |
|
void | FetchData (IssmDouble ***pmatrixarray, int **pmdims, int **pndims, int *pnumrecords, const char *data_name) |
|
void | FetchData (Options *options, const char *data_name) |
|
void | FetchData (int num,...) |
|
void | FetchDataToInput (Inputs2 *inputs2, Elements *elements, const char *vector_name, int input_enum) |
|
void | FetchDataToInput (Inputs2 *inputs2, Elements *elements, const char *vector_name, int input_enum, IssmDouble default_value) |
|
void | FetchDataToDatasetInput (Inputs2 *inputs2, Elements *elements, const char *vector_name, int input_enum) |
|
void | FetchIndependent (const char *dependent_name) |
|
void | FetchMultipleData (char ***pstringarray, int *pnumstrings, const char *data_name) |
|
void | FetchMultipleData (IssmDouble ***pmatrixarray, int **pmdims, int **pndims, int *pnumrecords, const char *data_name) |
|
void | FetchMultipleData (int ***pmatrices, int **pmdims, int **pndims, int *pnumrecords, const char *data_name) |
|
void | FetchMultipleData (int **pvector, int *pnum_instances, const char *data_name) |
|
void | FetchMultipleData (IssmDouble **pvector, int *pnum_instances, const char *data_name) |
|
fpos_t * | SetFilePointersToData (int **pcodes, int **pvector_types, int *pnum_instances, const char *data_name) |
|
FILE * | SetFilePointerToData (int *pcode, int *pvector_type, const char *data_name) |
|
void | StartTrace (bool trace) |
|
Definition at line 48 of file IoModel.h.
◆ ~IoModel()
Definition at line 240 of file IoModel.cpp.
243 vector<IoConstant*>::iterator iter1;
250 vector<IoData*>::iterator iter2;
251 for(iter2=
data.begin();iter2<
data.end();iter2++){
252 #if defined(_ISSM_DEBUG_)
253 if(!(*iter2)->isindependent){
254 _printf0_(
"WARNING: IoData \"" << (*iter2)->name <<
"\" has not been freed (DeleteData has not been called)\n");
269 xDelete<int>(this->
epart);
272 xDelete<int>(this->
faces);
274 xDelete<int>(this->
edges);
◆ IoModel() [1/2]
◆ IoModel() [2/2]
IoModel::IoModel |
( |
FILE * |
iomodel_handle, |
|
|
int |
solution_enum_in, |
|
|
bool |
trace, |
|
|
IssmPDouble * |
X |
|
) |
| |
Definition at line 171 of file IoModel.cpp.
174 bool iscontrol=
false;
177 this->
fid=iomodel_handle;
196 this->
FindConstant(&iscontrol,
"md.inversion.iscontrol");
201 if(autodiff && !iscontrol)
◆ AddConstant()
void IoModel::AddConstant |
( |
IoConstant * |
constant_in | ) |
|
Definition at line 288 of file IoModel.cpp.
293 vector<IoConstant*>::iterator iter;
296 if(strcmp((*iter)->name,in_constant->name)==0){
◆ AddConstantIndependent()
void IoModel::AddConstantIndependent |
( |
IoConstant * |
constant_in | ) |
|
Definition at line 305 of file IoModel.cpp.
310 in_constant->isindependent =
true;
◆ AddData()
void IoModel::AddData |
( |
IoData * |
data_in | ) |
|
Definition at line 316 of file IoModel.cpp.
321 vector<IoData*>::iterator iter;
323 for(iter=
data.begin();iter<
data.end();iter++){
324 if(strcmp((*iter)->name,in_data->name)==0){
330 this->
data.push_back(in_data);
◆ AddDataIndependent()
void IoModel::AddDataIndependent |
( |
IoData * |
data_in | ) |
|
Definition at line 333 of file IoModel.cpp.
338 in_data->isindependent =
true;
◆ FetchIndependentConstant()
void IoModel::FetchIndependentConstant |
( |
int * |
pXcount, |
|
|
IssmPDouble * |
X, |
|
|
const char * |
name |
|
) |
| |
Definition at line 1814 of file IoModel.cpp.
1821 if(X) Xcount=*pXcount;
1823 #ifdef _HAVE_AD_ //cannot come here unless you are running AD mode, from DeclaredIndependents:
1832 if(code!=3)
_error_(
"expecting a IssmDouble for \"" << constant_name<<
"\"");
1840 #if defined(_HAVE_CODIPACK_)
1847 auto& tape_codi = IssmDouble::getGlobalTape();
1848 tape_codi.registerInput(scalar);
1849 codi_global.input_indices.push_back(scalar.getGradientData());
1864 Xcount++; *pXcount=Xcount;
◆ FetchIndependentData()
void IoModel::FetchIndependentData |
( |
int * |
pXcount, |
|
|
IssmPDouble * |
X, |
|
|
const char * |
name |
|
) |
| |
Definition at line 1868 of file IoModel.cpp.
1875 if(X) Xcount=*pXcount;
1877 #ifdef _HAVE_AD_ //cannot come here unless you are running AD mode, from DeclaredIndependents:
1887 if((code!=5) && (code!=6) && (code!=7))
_error_(
"expecting a IssmDouble, integer or boolean matrix for \"" << data_name<<
"\"");
1892 if(fread(&M,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of rows for matrix ");
1897 if(fread(&N,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of columns for matrix ");
1903 buffer=xNew<IssmPDouble>(M*N);
1907 matrix=xNew<IssmDouble>(M*N,
"t");
1909 matrix=xNew<IssmDouble>(M*N);
1918 #if defined(_HAVE_CODIPACK_)
1920 auto& tape_codi = IssmDouble::getGlobalTape();
1922 for (
int i=0;i<M*N;i++) {
1923 matrix[i]=X[Xcount+i];
1924 tape_codi.registerInput(matrix[i]);
1925 codi_global.input_indices.push_back(matrix[i].getGradientData());
1929 for (
int i=0;i<M*N;i++) {
1930 matrix[i]=buffer[i];
1931 tape_codi.registerInput(matrix[i]);
1932 codi_global.input_indices.push_back(matrix[i].getGradientData());
1937 for(
int i=0;i<M*N;i++) matrix[i]<<=X[Xcount+i];
1940 for(
int i=0;i<M*N;i++) matrix[i]<<=buffer[i];
1946 xDelete<IssmPDouble>(buffer);
1948 else _error_(
"cannot declare the independent variable \"" << data_name <<
"\" if it's empty!");
1956 Xcount+=M*N; *pXcount=Xcount;
◆ FillIndependents()
Definition at line 2334 of file IoModel.cpp.
2339 int local_num_ind = 0;
2342 for(vector<IoConstant*>::iterator iter=
constants.begin();iter<
constants.end();iter++){
2343 if((*iter)->isindependent){
2344 (*iter)->constant->GetParameterValue(&xp[local_num_ind]);
2350 for(vector<IoData*>::iterator iter=
data.begin();iter<
data.end();iter++){
2351 if((*iter)->isindependent){
2352 for(
int i=0;i<(*iter)->M*(*iter)->N;i++){
2353 xp[local_num_ind+i] = (*iter)->data[i];
2355 local_num_ind += (*iter)->M*(*iter)->N;
◆ FindConstant() [1/5]
void IoModel::FindConstant |
( |
bool * |
pvalue, |
|
|
const char * |
constant_name |
|
) |
| |
Definition at line 2362 of file IoModel.cpp.
2365 vector<IoConstant*>::iterator iter;
2370 if(strcmp(ioconstant->
name,constant_name)==0){
2376 for(vector<IoConstant*>::iterator iter=
constants.begin();iter<
constants.end();iter++) (*iter)->constant->Echo();
2377 _error_(
"Could not find constant \""<<constant_name<<
"\"");
◆ FindConstant() [2/5]
void IoModel::FindConstant |
( |
int * |
pvalue, |
|
|
const char * |
constant_name |
|
) |
| |
Definition at line 2380 of file IoModel.cpp.
2383 vector<IoConstant*>::iterator iter;
2388 if(strcmp(ioconstant->
name,constant_name)==0){
2394 _error_(
"Could not find constant \""<<constant_name <<
"\"");
◆ FindConstant() [3/5]
void IoModel::FindConstant |
( |
IssmDouble * |
pvalue, |
|
|
const char * |
constant_name |
|
) |
| |
Definition at line 2397 of file IoModel.cpp.
2400 vector<IoConstant*>::iterator iter;
2405 if(strcmp(ioconstant->
name,constant_name)==0){
2411 _error_(
"Could not find constant \""<<constant_name <<
"\"");
◆ FindConstant() [4/5]
void IoModel::FindConstant |
( |
char ** |
pvalue, |
|
|
const char * |
constant_name |
|
) |
| |
Definition at line 2414 of file IoModel.cpp.
2417 vector<IoConstant*>::iterator iter;
2422 if(strcmp(ioconstant->
name,constant_name)==0){
2428 _error_(
"Could not find constant \""<<constant_name <<
"\"");
◆ FindConstant() [5/5]
void IoModel::FindConstant |
( |
char *** |
pvalue, |
|
|
int * |
psize, |
|
|
const char * |
constant_name |
|
) |
| |
Definition at line 2431 of file IoModel.cpp.
2434 vector<IoConstant*>::iterator iter;
2439 if(strcmp(ioconstant->
name,constant_name)==0){
2445 _error_(
"Could not find constant \""<<constant_name <<
"\"");
◆ NumIndependents()
int IoModel::NumIndependents |
( |
void |
| ) |
|
Definition at line 2448 of file IoModel.cpp.
2451 int num_independents = 0;
2454 for(vector<IoConstant*>::iterator iter=
constants.begin();iter<
constants.end();iter++){
2455 if((*iter)->isindependent){
2456 num_independents+= 1;
2461 for(vector<IoData*>::iterator iter=
data.begin();iter<
data.end();iter++){
2462 if((*iter)->isindependent){
2463 num_independents+= (*iter)->M*(*iter)->N;
2468 return num_independents;
◆ CheckFile()
void IoModel::CheckFile |
( |
void |
| ) |
|
Definition at line 344 of file IoModel.cpp.
347 int record_enum,record_name_size;
348 long long record_length;
349 char *record_name = NULL;
350 const char *mddot =
"md.";
362 fseek(this->
fid,0,SEEK_SET);
366 if(fread(&record_name_size,
sizeof(
int),1,
fid)==0){
368 xDelete<char>(record_name);
371 if(record_name_size<3 || record_name_size>80){
372 _error_(
"error while looking in binary file. Found a string of size "<<record_name_size);
376 record_name=xNew<char>(record_name_size+1);
377 record_name[record_name_size]=
'\0';
380 if(fread(record_name,record_name_size*
sizeof(
char),1,
fid)==0){
383 xDelete<char>(record_name);
386 if(strncmp(record_name,mddot,3)!=0){
387 _error_(
"error while reading binary file: record does not start with \"md.\": "<<record_name);
391 if(strncmp(record_name,
"md.EOF",6)==0){
393 xDelete<char>(record_name);
398 if(fread(&record_length,
sizeof(
long long),1,
fid)!=1)
_error_(
"Could not read record_length");
399 fseek(
fid,record_length,SEEK_CUR);
400 xDelete<char>(record_name);
404 _printf0_(
"=========================================================================\n");
405 _printf0_(
" Marshalled file is corrupted \n");
407 _printf0_(
" * Last record found is : \n");
408 _printf0_(
" the corresponding model field has probably been marshalled \n");
411 _printf0_(
"=========================================================================\n");
413 _error_(
"Binary file corrupted (See error message above)");
◆ CopyConstantObject()
Param * IoModel::CopyConstantObject |
( |
const char * |
constant_name, |
|
|
int |
param_enum |
|
) |
| |
Definition at line 418 of file IoModel.cpp.
421 vector<IoConstant*>::iterator iter;
426 if(strcmp(ioconstant->
name,constant_name)==0){
433 _error_(
"Constant \"" << constant_name <<
"\" not found in iomodel");
◆ Data()
IssmDouble * IoModel::Data |
( |
const char * |
data_name | ) |
|
Definition at line 437 of file IoModel.cpp.
440 vector<IoData*>::iterator iter;
442 for(iter=
data.begin();iter<
data.end();iter++){
444 if(strcmp(iodata->
name,data_name)==0)
return iodata->
data;
◆ DeclareIndependents()
void IoModel::DeclareIndependents |
( |
bool |
trace, |
|
|
IssmPDouble * |
X |
|
) |
| |
Definition at line 450 of file IoModel.cpp.
452 bool autodiff,iscontrol;
453 int num_independent_objects,temp;
460 this->
FetchData(&autodiff,
"md.autodiff.isautodiff");
461 this->
FetchData(&iscontrol,
"md.inversion.iscontrol");
463 if(trace || (autodiff && !iscontrol)){
467 this->
FetchData(&num_independent_objects,
"md.autodiff.num_independent_objects");
468 if(num_independent_objects){
469 this->
FetchData(&names,&temp,
"md.autodiff.independent_object_names");
470 _assert_(temp==num_independent_objects);
471 this->
FetchData(&types,NULL,NULL,
"md.autodiff.independent_object_types");
475 for(
int i=0;i<num_independent_objects;i++){
481 else if(types[i]==1){
486 _error_(
"Independent cannot be of size " << types[i]);
489 for(
int i=0;i<num_independent_objects;i++) xDelete<char>(names[i]);
490 xDelete<char*>(names);
495 _error_(
"Cannot carry out AD mode computations without support of ADOLC or CoDiPack compiled in!");
◆ DeleteData() [1/3]
void IoModel::DeleteData |
( |
int |
num, |
|
|
|
... |
|
) |
| |
Definition at line 500 of file IoModel.cpp.
504 char *data_name = NULL;
505 const char *mddot =
"md.";
506 vector<IoData *>::iterator iter;
510 for(
int i=0;i<num;i++){
511 data_name=va_arg(ap,
char*);
513 if(strncmp(data_name,mddot,3)!=0)
_error_(
"String provided does not start with \"md.\" ("<<data_name<<
")");
515 for(iter=
data.begin();iter<
data.end();iter++){
519 this->
data.erase(iter);
◆ DeleteData() [2/3]
void IoModel::DeleteData |
( |
IssmDouble * |
vector, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 526 of file IoModel.cpp.
528 vector<IoData*>::iterator iter;
531 if(!vector_in)
return;
534 for(iter=
data.begin();iter<
data.end();iter++){
542 xDelete<IssmDouble>(vector_in);
◆ DeleteData() [3/3]
void IoModel::DeleteData |
( |
char *** |
pstringarray, |
|
|
int |
numstrings, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 544 of file IoModel.cpp.
546 char** stringarray=*pstringarray;
549 for(
int i=0;i<numstrings;i++){
550 char*
string=stringarray[i];
551 xDelete<char>(
string);
553 xDelete<char*>(stringarray);
◆ FetchConstants()
void IoModel::FetchConstants |
( |
void |
| ) |
|
Definition at line 557 of file IoModel.cpp.
560 const char* mddot =
"md.";
561 char* record_name = NULL;
562 int record_name_size;
563 long long record_length;
572 char **strings = NULL;
573 int string_size,numstrings;
585 fseek(this->
fid,0,SEEK_SET);
591 if(fread(&record_name_size,
sizeof(
int),1,
fid)==0){
597 if(record_name_size<3 || record_name_size>80){
599 record_name=xNew<char>(record_name_size+1);
600 record_name[record_name_size]=
'\0';
603 fread(record_name,record_name_size*
sizeof(
char),1,
fid);
605 _error_(
"error while looking in binary file. String " << record_name <<
" a string of size "<<record_name_size);
609 record_name=xNew<char>(record_name_size+1);
610 record_name[record_name_size]=
'\0';
613 if(fread(record_name,record_name_size*
sizeof(
char),1,
fid)==0){
614 _error_(
"Could not read record name");
617 if(strncmp(record_name,mddot,3)!=0){
618 _error_(
"error while reading binary file: record does not start with \"md.\": "<<record_name);
622 if(fread(&record_length,
sizeof(
long long),1,this->
fid)!=1)
_error_(
"Cound not read record_length");
623 if(fread(&record_code ,
sizeof(
int),1,this->
fid)!=1)
_error_(
"Cound not read record_code");
636 if(fread(&booleanint,
sizeof(
int),1,this->
fid)!=1)
_error_(
"could not read boolean ");
645 if(fread(&integer,
sizeof(
int),1,this->
fid)!=1)
_error_(
"could not read integer ");
648 if(strcmp(record_name,
"md.smb.model")==0) integer =
IoCodeToEnumSMB(integer);
649 if(strcmp(record_name,
"md.basalforcings.model")==0) integer =
IoCodeToEnumBasal(integer);
654 if(strcmp(record_name,
"md.materials.nature")==0) integer =
IoCodeToEnumNature(integer);
656 if(strcmp(record_name,
"md.amr.type")==0) integer =
IoCodeToEnumAmr(integer);
669 vector<IoConstant*>::iterator iter;
672 if(strcmp(ioconstant->
name,record_name)==0){
689 if(fread(&string_size,
sizeof(
int),1,this->
fid)!=1)
_error_(
"could not read length of string ");
693 string=xNew<char>(string_size+1);
694 string[string_size]=
'\0';
697 if(fread(
string,string_size*
sizeof(
char),1,this->
fid)!=1)
_error_(
" could not read string ");
701 string=xNew<char>(1);
705 if(strcmp(record_name,
"md.flowequation.fe_SSA")==0){
707 }
else if(strcmp(record_name,
"md.flowequation.fe_HO")==0){
709 }
else if(strcmp(record_name,
"md.flowequation.fe_FS")==0){
711 }
else if(strcmp(record_name,
"md.thermal.fe")==0){
713 }
else if(strcmp(record_name,
"md.levelset.fe")==0){
715 }
else if(strcmp(record_name,
"md.groundingline.migration")==0){
717 }
else if(strcmp(record_name,
"md.groundingline.friction_interpolation")==0){
719 }
else if(strcmp(record_name,
"md.groundingline.melt_interpolation")==0){
721 }
else if(strcmp(record_name,
"md.masstransport.hydrostatic_adjustment")==0){
723 }
else if(strcmp(record_name,
"md.materials.rheology_law")==0){
725 }
else if(strcmp(record_name,
"md.damage.elementinterp")==0){
727 }
else if(strcmp(record_name,
"md.mesh.domain_type")==0){
729 }
else if(strcmp(record_name,
"md.mesh.elementtype")==0){
737 xDelete<char>(
string);
746 fseek(
fid,-
sizeof(
int),SEEK_CUR);
747 fseek(
fid,record_length,SEEK_CUR);
751 if(fread(&numstrings,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read length of string array");
755 strings=xNew<char*>(numstrings);
756 for(
int i=0;i<numstrings;i++)strings[i]=NULL;
759 for(
int i=0;i<numstrings;i++){
761 if(fread(&string_size,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read length of string ");
764 string=xNew<char>((string_size+1));
765 string[string_size]=
'\0';
766 if(fread(
string,string_size*
sizeof(
char),1,
fid)!=1)
_error_(
" could not read string ");
770 string=xNew<char>(1);
781 for(
int i=0;i<numstrings;i++) xDelete<char>(strings[i]);
782 xDelete<char*>(strings);
785 _error_(
"unknown record type:" << record_code);
788 xDelete<char>(record_name);
800 record_name=xNew<char>((record_name_size+1)); record_name[record_name_size]=
'\0';
824 vector<IoConstant*>::iterator iter;
827 if(strcmp(ioconstant->
name,record_name)==0){
843 string=xNew<char>((string_size+1));
844 string[string_size]=
'\0';
850 string=xNew<char>(1);
854 if(strcmp(record_name,
"md.flowequation.fe_SSA")==0){
856 }
else if(strcmp(record_name,
"md.flowequation.fe_HO")==0){
858 }
else if(strcmp(record_name,
"md.flowequation.fe_FS")==0){
860 }
else if(strcmp(record_name,
"md.thermal.fe")==0){
862 }
else if(strcmp(record_name,
"md.levelset.fe")==0){
864 }
else if(strcmp(record_name,
"md.groundingline.migration")==0){
866 }
else if(strcmp(record_name,
"md.groundingline.friction_interpolation")==0){
868 }
else if(strcmp(record_name,
"md.groundingline.melt_interpolation")==0){
870 }
else if(strcmp(record_name,
"md.masstransport.hydrostatic_adjustment")==0){
872 }
else if(strcmp(record_name,
"md.materials.rheology_law")==0){
874 }
else if(strcmp(record_name,
"md.damage.elementinterp")==0){
876 }
else if(strcmp(record_name,
"md.mesh.domain_type")==0){
878 }
else if(strcmp(record_name,
"md.mesh.elementtype")==0){
886 xDelete<char>(
string);
897 strings=xNew<char*>(numstrings);
898 for(
int i=0;i<numstrings;i++)strings[i]=NULL;
901 for(
int i=0;i<numstrings;i++){
905 string=xNew<char>((string_size+1));
906 string[string_size]=
'\0';
910 string=xNew<char>(1);
921 for(
int i=0;i<numstrings;i++) xDelete<char>(strings[i]);
922 xDelete<char*>(strings);
925 _error_(
"unknown record type:" << record_code);
928 xDelete<char>(record_name);
◆ FetchData() [1/10]
void IoModel::FetchData |
( |
bool * |
pboolean, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 933 of file IoModel.cpp.
945 if(code!=1)
_error_(
"expecting a boolean for \"" << data_name<<
"\"");
949 if(fread(&booleanint,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read boolean ");
955 *pboolean=(bool)booleanint;
◆ FetchData() [2/10]
void IoModel::FetchData |
( |
int * |
pinteger, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 959 of file IoModel.cpp.
970 if(code!=2)
_error_(
"expecting an integer for \"" << data_name<<
"\"");
974 if(fread(&integer,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read integer ");
◆ FetchData() [3/10]
void IoModel::FetchData |
( |
IssmDouble * |
pscalar, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 983 of file IoModel.cpp.
995 if(code!=3)
_error_(
"expecting a IssmDouble for \""<<data_name<<
"\"");
◆ FetchData() [4/10]
void IoModel::FetchData |
( |
char ** |
pstring, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 1008 of file IoModel.cpp.
1021 if(code!=4)
_error_(
"expecting a string for \""<<data_name<<
"\"");
1027 if(fread(&string_size,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read length of string ");
1034 string=xNew<char>((string_size+1));
1035 string[string_size]=
'\0';
1039 if(fread(
string,string_size*
sizeof(
char),1,
fid)!=1)
_error_(
" could not read string ");
1044 string=xNew<char>(1);
◆ FetchData() [5/10]
void IoModel::FetchData |
( |
char *** |
pstrings, |
|
|
int * |
pnumstrings, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 1052 of file IoModel.cpp.
1055 char** strings = NULL;
1056 char*
string = NULL;
1067 if(code!=9)
_error_(
"expecting a string array for \""<<data_name<<
"\"");
1072 if(fread(&numstrings,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read length of string array");
1078 strings=xNew<char*>(numstrings);
1079 for(
int i=0;i<numstrings;i++) strings[i]=NULL;
1082 for(
int i=0;i<numstrings;i++){
1085 if(fread(&string_size,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read length of string ");
1089 string=xNew<char>((string_size+1));
1090 string[string_size]=
'\0';
1092 if(fread(
string,string_size*
sizeof(
char),1,
fid)!=1)
_error_(
" could not read string ");
1097 string=xNew<char>(1);
1105 *pstrings = strings;
1106 if(pnumstrings) *pnumstrings = numstrings;
◆ FetchData() [6/10]
void IoModel::FetchData |
( |
int ** |
pmatrix, |
|
|
int * |
pM, |
|
|
int * |
pN, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 1109 of file IoModel.cpp.
1115 int* integer_matrix=NULL;
1124 if(code!=5 && code!=6 && code!=7)
_error_(
"expecting a IssmDouble, integer or boolean matrix for \""<<data_name<<
"\""<<
" (Code is "<<code<<
")");
1131 if(fread(&M,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of rows for matrix ");
1137 if(fread(&N,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of columns for matrix ");
1143 matrix=xNew<IssmPDouble>(M*N);
1155 integer_matrix=xNew<int>(M*N);
1158 integer_matrix[i*N+j]=(int)matrix[i*N+j];
1163 integer_matrix=NULL;
1166 xDelete<IssmPDouble>(matrix);
1169 *pmatrix=integer_matrix;
◆ FetchData() [7/10]
void IoModel::FetchData |
( |
IssmDouble ** |
pscalarmatrix, |
|
|
int * |
pM, |
|
|
int * |
pN, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 1175 of file IoModel.cpp.
1178 vector<IoData*>::iterator iter;
1179 for(iter=
data.begin();iter<
data.end();iter++){
1181 if(strcmp(iodata->
name,data_name)==0){
1182 *pmatrix=iodata->
data;
1183 if(pM) *pM=iodata->
M;
1184 if(pN) *pN=iodata->
N;
1199 if(code!=5 && code!=6 && code!=7 && code!=10)
_error_(
"expecting a IssmDouble, integer or boolean matrix for \""<<data_name<<
"\""<<
" (Code is "<<code<<
")");
1206 if(fread(&M,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of rows for matrix ");
1211 if(fread(&N,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of columns for matrix ");
1226 *pmatrix=xNew<IssmDouble>(M*N);
1229 uint8_t* rawmatrix=xNew<uint8_t>((M-1)*N);
1230 if(my_rank==0)
if(fread(rawmatrix,(M-1)*N*
sizeof(
char),1,
fid)!=1)
_error_(
"could not read matrix ");
1233 for(
int i=0;i<(M-1)*N;++i) (*pmatrix)[i]=offset+range*reCast<IssmDouble>(rawmatrix[i])/255.;
1234 xDelete<uint8_t>(rawmatrix);
1238 if(my_rank==0)
if(fread(timematrix,N*
sizeof(
IssmPDouble),1,
fid)!=1)
_error_(
"could not read time in compressed matrix");
1241 for(
int i=0;i<N;++i) (*pmatrix)[(M-1)*N+i]=timematrix[i];
1242 xDelete<IssmPDouble>(timematrix);
1247 matrix=xNew<IssmPDouble>(M*N);
1248 if(my_rank==0)
if(fread(matrix,M*N*
sizeof(
IssmPDouble),1,
fid)!=1)
_error_(
"could not read matrix ");
1251 *pmatrix=xNew<IssmDouble>(M*N);
1252 for(
int i=0;i<M*N;++i) (*pmatrix)[i]=matrix[i];
1253 xDelete<IssmPDouble>(matrix);
◆ FetchData() [8/10]
void IoModel::FetchData |
( |
IssmDouble *** |
pmatrixarray, |
|
|
int ** |
pmdims, |
|
|
int ** |
pndims, |
|
|
int * |
pnumrecords, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 1264 of file IoModel.cpp.
1283 if(code!=8)
_error_(
"expecting a IssmDouble mat array for \""<<data_name<<
"\"");
1287 if(fread(&numrecords,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of records in matrix array ");
1294 matrices=xNew<IssmDouble*>(numrecords);
1295 mdims=xNew<int>(numrecords);
1296 ndims=xNew<int>(numrecords);
1298 for(i=0;i<numrecords;i++){
1305 for(i=0;i<numrecords;i++){
1308 if(fread(&M,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of rows in " << i <<
"th matrix of matrix array");
1313 if(fread(&N,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of columns in " << i <<
"th matrix of matrix array");
1319 matrix=xNew<IssmPDouble>(M*N);
1327 matrices[i]=xNew<IssmDouble>(M*N);
1328 for (
int j=0;j<M*N;++j) {matrices[i][j]=matrix[j];}
1329 xDelete<IssmPDouble>(matrix);
1340 *pmatrices=matrices;
1343 *pnumrecords=numrecords;
◆ FetchData() [9/10]
void IoModel::FetchData |
( |
Options * |
options, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 1346 of file IoModel.cpp.
1349 const char* mddot =
"md.";
1350 char* record_name = NULL;
1351 int record_name_size;
1352 long long record_length;
1357 char *
string = NULL;
1365 fseek(
fid,0,SEEK_SET);
1368 if(fread(&record_name_size,
sizeof(
int),1,
fid)==0)
_error_(
"could not read record_name");
1369 if(record_name_size<3 || record_name_size>80)
_error_(
"error while looking in binary file. Found a string of size "<<record_name_size);
1372 record_name=xNew<char>(record_name_size+1);
1373 record_name[record_name_size]=
'\0';
1376 if(fread(record_name,record_name_size*
sizeof(
char),1,
fid)==0)
_error_(
"Could not find field "<<lastnonoption);
1377 if(strncmp(record_name,mddot,3)!=0)
_error_(
"error while reading binary file: record does not start with \"md.\": "<<record_name);
1380 if(strcmp(record_name,lastnonoption)==0){
1381 if(fread(&record_length,
sizeof(
long long),1,
fid)!=1)
_error_(
"Could not read record_length");
1382 fseek(
fid,record_length,SEEK_CUR);
1383 xDelete<char>(record_name);
1387 if(fread(&record_length,
sizeof(
long long),1,
fid)!=1)
_error_(
"Could not read record_length");
1388 fseek(
fid,record_length,SEEK_CUR);
1389 xDelete<char>(record_name);
1401 if(fread(&record_name_size,
sizeof(
int),1,
fid)==0){
1407 if(record_name_size<3 || record_name_size>80){
1408 _error_(
"error while looking in binary file. Found a string of size "<<record_name_size);
1412 record_name=xNew<char>(record_name_size+1);
1413 record_name[record_name_size]=
'\0';
1416 if(fread(record_name,record_name_size*
sizeof(
char),1,
fid)==0){
1417 _error_(
"Could not read record name");
1419 if(strncmp(record_name,mddot,3)!=0){
1420 _error_(
"error while reading binary file: record does not start with \"md.\": "<<record_name);
1422 if(strcmp(record_name,
"md.EOF")==0){
1423 xDelete<char>(record_name);
1430 if(fread(&record_length,
sizeof(
long long),1,this->
fid)!=1)
_error_(
"Cound not read record_length");
1431 if(fread(&record_code ,
sizeof(
int),1,this->
fid)!=1)
_error_(
"Cound not read record_code");
1441 switch(record_code){
1447 char* optionname=xNew<char>(strlen(record_name)-3+1);
1448 xMemCpy(optionname,&record_name[3],strlen(record_name)-3+1);
1449 option->
value = scalar;
1450 option->
name = optionname;
1451 option->
size[0] = 1;
1452 option->
size[1] = 1;
1459 if(fread(&string_size,
sizeof(
int),1,this->
fid)!=1)
_error_(
"could not read length of string ");
1463 string=xNew<char>(string_size+1);
1464 string[string_size]=
'\0';
1467 if(fread(
string,string_size*
sizeof(
char),1,this->
fid)!=1)
_error_(
" could not read string ");
1471 string=xNew<char>(1);
1477 char* optionname=xNew<char>(strlen(record_name)-3+1);
1478 xMemCpy(optionname,&record_name[3],strlen(record_name)-3+1);
1479 option->
value = string;
1480 option->
name = optionname;
1481 option->
size[0] = 1;
1482 option->
size[1] = 1;
1488 _error_(
"record type not supported:" << record_code);
1491 xDelete<char>(record_name);
1503 record_name=xNew<char>((record_name_size+1)); record_name[record_name_size]=
'\0';
1506 switch(record_code){
1511 char* optionname=xNew<char>(strlen(record_name)-3+1);
1512 xMemCpy(optionname,&record_name[3],strlen(record_name)-3+1);
1514 option->
value = scalar;
1515 option->
name = optionname;
1516 option->
size[0] = 1;
1517 option->
size[1] = 1;
1524 if(fread(&string_size,
sizeof(
int),1,this->
fid)!=1)
_error_(
"could not read length of string ");
1528 string=xNew<char>(string_size+1);
1529 string[string_size]=
'\0';
1532 if(fread(
string,string_size*
sizeof(
char),1,this->
fid)!=1)
_error_(
" could not read string ");
1536 string=xNew<char>(1);
1541 char* optionname=xNew<char>(strlen(record_name)-3+1);
1542 xMemCpy(optionname,&record_name[3],strlen(record_name)-3+1);
1544 option->
value = string;
1545 option->
name = optionname;
1546 option->
size[0] = 1;
1547 option->
size[1] = 1;
1552 _error_(
"record type not supported:" << record_code);
◆ FetchData() [10/10]
void IoModel::FetchData |
( |
int |
num, |
|
|
|
... |
|
) |
| |
Definition at line 1562 of file IoModel.cpp.
1570 const char *mddot =
"md.";
1571 vector<IoData*>::iterator iter;
1577 for(
int i=0; i<num; i++){
1579 data_name=va_arg(ap,
char*);
1580 if(strncmp(data_name,mddot,3)!=0)
_error_(
"String provided does not start with \"md.\" ("<<data_name<<
")");
1584 for(iter=
data.begin();iter<
data.end();iter++){
1586 if(strcmp(iodata->
name,data_name)==0){
1601 this->
FetchData(&matrix,&M,&N,data_name);
1602 this->
AddData(
new IoData(matrix,code,layout,M,N,data_name));
◆ FetchDataToInput() [1/2]
void IoModel::FetchDataToInput |
( |
Inputs2 * |
inputs2, |
|
|
Elements * |
elements, |
|
|
const char * |
vector_name, |
|
|
int |
input_enum |
|
) |
| |
Definition at line 1651 of file IoModel.cpp.
1654 vector<IoData*>::iterator iter;
1655 for(iter=
data.begin();iter<
data.end();iter++){
1657 if(strcmp(iodata->
name,vector_name)==0){
1658 for(
int i=0;i<
elements->Size();i++){
1659 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1668 int code,vector_layout;
1684 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1691 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1698 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1703 this->
FetchData(&doublearray,&M,&N,vector_name);
1704 if(!doublearray)
_error_(
"\""<<vector_name<<
"\" not found in binary file");
1706 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1707 element->
InputCreate(doublearray,inputs2,
this,M,N,vector_layout,input_enum,code);
1711 this->
FetchData(&doublearray,&M,&N,vector_name);
1712 if(!doublearray)
_error_(
"\""<<vector_name<<
"\" not found in binary file");
1714 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1715 element->
InputCreate(doublearray,inputs2,
this,M,N,vector_layout,input_enum,code);
1720 this->
FetchData(&doublearray,&M,&N,vector_name);
1721 if(!doublearray)
_error_(
"\""<<vector_name<<
"\" not found in binary file");
1723 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1724 element->
InputCreate(doublearray,inputs2,
this,M,N,vector_layout,input_enum,code);
1728 _error_(
"data code " << code <<
" not supported yet (detected while processing \""<<vector_name<<
"\")");
1732 xDelete<IssmDouble>(doublearray);
◆ FetchDataToInput() [2/2]
void IoModel::FetchDataToInput |
( |
Inputs2 * |
inputs2, |
|
|
Elements * |
elements, |
|
|
const char * |
vector_name, |
|
|
int |
input_enum, |
|
|
IssmDouble |
default_value |
|
) |
| |
Definition at line 1608 of file IoModel.cpp.
1611 vector<IoData*>::iterator iter;
1612 for(iter=
data.begin();iter<
data.end();iter++){
1614 if(strcmp(iodata->
name,vector_name)==0){
1616 for(
int i=0;i<
elements->Size();i++){
1617 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1625 int code,vector_layout;
1633 if(code!=7 && code!=10)
_error_(vector_name<<
" is not a double array");
1635 this->
FetchData(&doublearray,&M,&N,vector_name);
1637 for(
int i=0;i<
elements->Size();i++){
1638 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1643 element->
InputCreate(doublearray,inputs2,
this,M,N,vector_layout,input_enum,code);
1648 xDelete<IssmDouble>(doublearray);
◆ FetchDataToDatasetInput()
void IoModel::FetchDataToDatasetInput |
( |
Inputs2 * |
inputs2, |
|
|
Elements * |
elements, |
|
|
const char * |
vector_name, |
|
|
int |
input_enum |
|
) |
| |
Definition at line 1735 of file IoModel.cpp.
1738 vector<IoData*>::iterator iter;
1739 for(iter=
data.begin();iter<
data.end();iter++){
1741 if(strcmp(iodata->
name,vector_name)==0){
1742 for(
int i=0;i<
elements->Size();i++){
1743 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1744 _error_(
"to be implemented...");
1752 int code,vector_layout;
1761 _error_(
"not implemented yet");
1764 _error_(
"not implemented yet");
1767 _error_(
"not implemented yet");
1770 _error_(
"not implemented yet");
1773 _error_(
"not implemented yet");
1777 this->
FetchData(&doublearray,&M,&N,vector_name);
1778 if(!doublearray)
_error_(
"\""<<vector_name<<
"\" not found in binary file");
1780 int* ids = xNew<int>(N);
1781 for(
int i=0;i<N;i++) ids[i] = i;
1783 for(
int i=0;i<
elements->Size();i++){
1784 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1792 this->
FetchData(&doublearray,&M,&N,vector_name);
1793 if(!doublearray)
_error_(
"\""<<vector_name<<
"\" not found in binary file");
1795 int* ids = xNew<int>(N);
1796 for(
int i=0;i<N;i++) ids[i] = i;
1798 for(
int i=0;i<
elements->Size();i++){
1799 Element* element=xDynamicCast<Element*>(
elements->GetObjectByOffset(i));
1807 _error_(
"data code " << code <<
" not supported yet (detected while processing \""<<vector_name<<
"\")");
1811 xDelete<IssmDouble>(doublearray);
◆ FetchIndependent()
void IoModel::FetchIndependent |
( |
const char * |
dependent_name | ) |
|
◆ FetchMultipleData() [1/5]
void IoModel::FetchMultipleData |
( |
char *** |
pstringarray, |
|
|
int * |
pnumstrings, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 1960 of file IoModel.cpp.
1966 char **strings = NULL;
1969 char *
string = NULL;
1973 fpos_t *file_positions = NULL;
1982 strings=xNew<char*>(num_instances);
1984 for(
int i=0;i<num_instances;i++){
1988 if(codes[i]!=4)
_error_(
"expecting a string for \""<<data_name<<
"\" but code is "<<codes[i]<<
" not 4");
1991 fsetpos(
fid,file_positions+i);
1992 if(fread(&string_size,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read length of string ");
1999 string=xNew<char>((string_size+1));
2000 string[string_size]=
'\0';
2004 if(fread(
string,string_size*
sizeof(
char),1,
fid)!=1)
_error_(
" could not read string ");
2009 string=xNew<char>(1);
2016 xDelete<int>(codes);
2017 xDelete<fpos_t>(file_positions);
2021 *pnumstrings=num_instances;
◆ FetchMultipleData() [2/5]
void IoModel::FetchMultipleData |
( |
IssmDouble *** |
pmatrixarray, |
|
|
int ** |
pmdims, |
|
|
int ** |
pndims, |
|
|
int * |
pnumrecords, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 2130 of file IoModel.cpp.
2133 fpos_t* file_positions=NULL;
2156 matrices=xNew<IssmDouble*>(num_instances);
2157 mdims=xNew<int>(num_instances);
2158 ndims=xNew<int>(num_instances);
2160 for(
int i=0;i<num_instances;i++){
2165 if((code!=5) && (code!=6) && (code!=7))
_error_(
"expecting a IssmDouble, integer or boolean matrix for \""<<data_name<<
"\"");
2169 fsetpos(
fid,file_positions+i);
2170 if(fread(&M,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of rows for matrix ");
2175 if(fread(&N,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of columns for matrix ");
2181 pmatrix=xNew<IssmPDouble>(M*N);
2195 matrix=xNew<IssmDouble>(M*N);
2196 for (
int i=0;i<M*N;++i) matrix[i]=pmatrix[i];
2198 xDelete<IssmPDouble>(pmatrix);
2211 xDelete<fpos_t>(file_positions);
2212 xDelete<int>(codes);
2215 *pmatrices=matrices;
2220 xDelete<int>(mdims);
2226 xDelete<int>(ndims);
2228 *pnumrecords=num_instances;
◆ FetchMultipleData() [3/5]
void IoModel::FetchMultipleData |
( |
int *** |
pmatrices, |
|
|
int ** |
pmdims, |
|
|
int ** |
pndims, |
|
|
int * |
pnumrecords, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 2231 of file IoModel.cpp.
2234 fpos_t* file_positions=NULL;
2237 int **matrices = NULL;
2245 int *integer_matrix=NULL;
2258 matrices=xNew<int*>(num_instances);
2259 mdims=xNew<int>(num_instances);
2260 ndims=xNew<int>(num_instances);
2262 for(
int i=0;i<num_instances;i++){
2267 if((code!=5) && (code!=6) && (code!=7))
_error_(
"expecting a IssmDouble, integer or boolean matrix for \""<<data_name<<
"\"");
2271 fsetpos(
fid,file_positions+i);
2272 if(fread(&M,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of rows for matrix ");
2277 if(fread(&N,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read number of columns for matrix ");
2283 pmatrix=xNew<IssmPDouble>(M*N);
2284 integer_matrix=xNew<int>(M*N);
2299 for (
int i=0;i<M*N;++i) integer_matrix[i]=pmatrix[i];
2301 xDelete<IssmPDouble>(pmatrix);
2304 integer_matrix=NULL;
2308 matrices[i]=integer_matrix;
2314 xDelete<fpos_t>(file_positions);
2315 xDelete<int>(codes);
2318 *pmatrices=matrices;
2323 xDelete<int>(mdims);
2329 xDelete<int>(ndims);
2331 *pnumrecords=num_instances;
◆ FetchMultipleData() [4/5]
void IoModel::FetchMultipleData |
( |
int ** |
pvector, |
|
|
int * |
pnum_instances, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 2024 of file IoModel.cpp.
2027 fpos_t* file_positions=NULL;
2046 vector=xNew<int>(num_instances);
2048 for(
int i=0;i<num_instances;i++){
2053 if(code!=2)
_error_(
"expecting an integer for \""<<data_name<<
"\"");
2056 fsetpos(
fid,file_positions+i);
2058 if(fread(&integer,
sizeof(
int),1,
fid)!=1)
_error_(
"could not read integer ");
2069 xDelete<fpos_t>(file_positions);
2070 xDelete<int>(codes);
2074 *pnum_instances=num_instances;
◆ FetchMultipleData() [5/5]
void IoModel::FetchMultipleData |
( |
IssmDouble ** |
pvector, |
|
|
int * |
pnum_instances, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 2077 of file IoModel.cpp.
2080 fpos_t* file_positions=NULL;
2099 vector=xNew<IssmDouble>(num_instances);
2101 for(
int i=0;i<num_instances;i++){
2106 if(code!=3)
_error_(
"expecting a double for \""<<data_name<<
"\"");
2109 fsetpos(
fid,file_positions+i);
2122 xDelete<fpos_t>(file_positions);
2123 xDelete<int>(codes);
2127 *pnum_instances=num_instances;
◆ SetFilePointersToData()
fpos_t * IoModel::SetFilePointersToData |
( |
int ** |
pcodes, |
|
|
int ** |
pvector_types, |
|
|
int * |
pnum_instances, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 2471 of file IoModel.cpp.
2474 const char* mddot =
"md.";
2475 char* record_name = NULL;
2476 int record_name_size;
2477 long long record_length;
2480 int *vector_types = NULL;
2482 int num_instances = 0;
2484 fpos_t *file_positions = NULL;
2488 _assert_(strncmp(data_name,mddot,3)==0);
2495 fseek(
fid,0,SEEK_SET);
2500 if(fread(&record_name_size,
sizeof(
int),1,
fid)==0){
2502 xDelete<char>(record_name);
2505 if(record_name_size<3 || record_name_size>80){
2506 _error_(
"error while looking in binary file. Found a string of size "<<record_name_size);
2510 record_name=xNew<char>(record_name_size+1);
2511 record_name[record_name_size]=
'\0';
2514 if(fread(record_name,record_name_size*
sizeof(
char),1,
fid)==0){
2517 if(strncmp(record_name,mddot,3)!=0){
2518 _error_(
"error while reading binary file: record does not start with \"md.\": "<<record_name);
2522 if(strcmp(record_name,data_name)==0) num_instances++;
2525 if(fread(&record_length,
sizeof(
long long),1,
fid)!=1)
_error_(
"Could not read record_length");
2526 fseek(
fid,record_length,SEEK_CUR);
2527 xDelete<char>(record_name);
2532 file_positions = xNew<fpos_t>(num_instances);
2533 codes = xNew<int>(num_instances);
2534 vector_types = xNew<int>(num_instances);
2540 fseek(
fid,0,SEEK_SET);
2544 if(fread(&record_name_size,
sizeof(
int),1,
fid)==0){
2548 if(record_name_size<3 || record_name_size>80){
2549 _error_(
"error while looking in binary file. Found a string of size "<<record_name_size);
2553 record_name=xNew<char>(record_name_size+1);
2554 record_name[record_name_size]=
'\0';
2557 if(fread(record_name,record_name_size*
sizeof(
char),1,
fid)==0){
2559 xDelete<char>(record_name);
2562 if(strncmp(record_name,mddot,3)!=0){
2563 _error_(
"error while reading binary file: record does not start with \"md.\": "<<record_name);
2567 if(strcmp(record_name,data_name)==0){
2569 fseek(
fid,
sizeof(
long long),SEEK_CUR);
2570 if(fread(&record_code,
sizeof(
int),1,
fid)!=1)
_error_(
"Could not read record_code");
2573 if(5<=record_code && record_code<=7){
2574 if(fread(&vector_type,
sizeof(
int),1,
fid)!=1)
_error_(
"Could not read vector_type");
2576 codes[counter] = record_code;
2577 vector_types[counter] = vector_type;
2578 fgetpos(
fid,file_positions+counter);
2581 if(5<=record_code && record_code<=7) fseek(
fid,-
sizeof(
int),SEEK_CUR);
2582 fseek(
fid,-
sizeof(
int),SEEK_CUR);
2583 fseek(
fid,-
sizeof(
long long),SEEK_CUR);
2590 if(fread(&record_length,
sizeof(
long long),1,
fid)!=1)
_error_(
"Could not read record_length");
2592 fseek(
fid,record_length,SEEK_CUR);
2593 xDelete<char>(record_name);
2602 *pnum_instances = num_instances;
2604 *pvector_types=vector_types;
2607 xDelete<int>(vector_types);
2609 return file_positions;
◆ SetFilePointerToData()
FILE * IoModel::SetFilePointerToData |
( |
int * |
pcode, |
|
|
int * |
pvector_type, |
|
|
const char * |
data_name |
|
) |
| |
Definition at line 2612 of file IoModel.cpp.
2617 const char* mddot =
"md.";
2618 char* record_name = NULL;
2619 int record_name_size;
2620 long long record_length;
2622 int vector_type = 0;
2626 if(strncmp(data_name,mddot,3)!=0){
2627 _error_(
"Cannot fetch \""<<data_name<<
"\" does not start with \""<<mddot<<
"\"");
2635 fseek(
fid,0,SEEK_SET);
2640 if(fread(&record_name_size,
sizeof(
int),1,
fid)==0){
2642 xDelete<char>(record_name);
2645 if(record_name_size<3 || record_name_size>80){
2646 _error_(
"error while looking in binary file. Found a string of size "<<record_name_size);
2650 record_name=xNew<char>(record_name_size+1);
2651 record_name[record_name_size]=
'\0';
2654 if(fread(record_name,record_name_size*
sizeof(
char),1,
fid)==0){
2657 xDelete<char>(record_name);
2660 if(strncmp(record_name,mddot,3)!=0){
2661 _error_(
"error while reading binary file: record does not start with \"md.\": "<<record_name);
2665 if(strcmp(record_name,data_name)==0){
2667 fseek(
fid,
sizeof(
long long),SEEK_CUR);
2668 if(fread(&record_code,
sizeof(
int),1,
fid)!=1)
_error_(
"Could not read record_code");
2670 if((5<=record_code && record_code<=7) || record_code==10){
2671 if(fread(&vector_type,
sizeof(
int),1,
fid)!=1)
_error_(
"Could not read vector_type");
2674 xDelete<char>(record_name);
2679 if(fread(&record_length,
sizeof(
long long),1,
fid)!=1)
_error_(
"Could not read record_length");
2681 fseek(
fid,record_length,SEEK_CUR);
2682 xDelete<char>(record_name);
2687 if(!found)
_error_(
"could not find data with name \"" << data_name <<
"\" in binary file");
2695 if(pvector_type)*pvector_type=vector_type;
◆ StartTrace()
void IoModel::StartTrace |
( |
bool |
trace | ) |
|
Definition at line 2700 of file IoModel.cpp.
2702 bool autodiff =
false;
2703 bool iscontrol =
false;
2714 this->
FetchData(&autodiff,
"md.autodiff.isautodiff");
2715 this->
FetchData(&iscontrol,
"md.inversion.iscontrol");
2717 if(trace || (autodiff && !iscontrol)){
2719 #if defined(_HAVE_ADOLC_)
2721 this->
FetchData(&keep,
"md.autodiff.keep");
2722 int keepTaylors=keep?1:0;
2723 this->
FetchData(&gcTriggerRatio,
"md.autodiff.gcTriggerRatio");
2724 this->
FetchData(&gcTriggerMaxSize,
"md.autodiff.gcTriggerMaxSize");
2725 this->
FetchData(&obufsize,
"md.autodiff.obufsize");
2726 this->
FetchData(&lbufsize,
"md.autodiff.lbufsize");
2727 this->
FetchData(&cbufsize,
"md.autodiff.cbufsize");
2728 this->
FetchData(&tbufsize,
"md.autodiff.tbufsize");
2731 setStoreManagerControl(reCast<IssmPDouble>(gcTriggerRatio),reCast<size_t>(gcTriggerMaxSize));
2734 int skipFileDeletion=1;
2735 trace_on(my_rank,keepTaylors,reCast<size_t>(obufsize),reCast<size_t>(lbufsize),reCast<size_t>(cbufsize),reCast<size_t>(tbufsize),skipFileDeletion);
2737 #elif defined(_HAVE_CODIPACK_)
2745 auto& tape_codi = IssmDouble::getGlobalTape();
2746 tape_codi.setActive();
2750 tape_codi.registerInput(y_t);
2751 int codi_allocn = 0;
2752 this->
FetchData(&codi_allocn,
"md.autodiff.tapeAlloc");
2753 for(
int i = 0;i < codi_allocn;++i) {
◆ constants
◆ data
std::vector<IoData*> IoModel::data |
|
private |
◆ fid
◆ solution_enum
int IoModel::solution_enum |
◆ my_elements
bool* IoModel::my_elements |
◆ my_faces
◆ my_vfaces
◆ my_edges
◆ my_vedges
◆ my_hedges
◆ my_vertices
bool* IoModel::my_vertices |
◆ my_vertices_lids
int* IoModel::my_vertices_lids |
◆ epart
◆ domaindim
◆ domaintype
◆ elements
◆ edges
◆ verticaledges
int* IoModel::verticaledges |
◆ horizontaledges
int* IoModel::horizontaledges |
◆ elementtoedgeconnectivity
int* IoModel::elementtoedgeconnectivity |
◆ elementtoverticaledgeconnectivity
int* IoModel::elementtoverticaledgeconnectivity |
◆ elementtohorizontaledgeconnectivity
int* IoModel::elementtohorizontaledgeconnectivity |
◆ elementtofaceconnectivity
int* IoModel::elementtofaceconnectivity |
◆ elementtoverticalfaceconnectivity
int* IoModel::elementtoverticalfaceconnectivity |
◆ faces
◆ verticalfaces
int* IoModel::verticalfaces |
◆ facescols
◆ meshelementtype
int IoModel::meshelementtype |
◆ numbernodetoelementconnectivity
int* IoModel::numbernodetoelementconnectivity |
◆ numberofedges
int IoModel::numberofedges |
◆ numberofverticaledges
int IoModel::numberofverticaledges |
◆ numberofhorizontaledges
int IoModel::numberofhorizontaledges |
◆ numberofelements
int IoModel::numberofelements |
◆ numberoffaces
int IoModel::numberoffaces |
◆ numberofverticalfaces
int IoModel::numberofverticalfaces |
◆ numberofvertices
int IoModel::numberofvertices |
◆ singlenodetoelementconnectivity
int* IoModel::singlenodetoelementconnectivity |
The documentation for this class was generated from the following files:
void AddConstantIndependent(IoConstant *constant_in)
void SetIntInput(Inputs2 *inputs2, int enum_in, int value)
#define _printf0_(StreamArgs)
void SetBoolInput(Inputs2 *inputs2, int enum_in, bool value)
int * numbernodetoelementconnectivity
int * elementtohorizontaledgeconnectivity
int * elementtofaceconnectivity
void DeclareIndependents(bool trace, IssmPDouble *X)
std::vector< IoConstant * > constants
static ISSM_MPI_Comm GetComm(void)
int IoCodeToEnumMaterials(int enum_in)
virtual void SetEnum(int enum_in)=0
std::vector< IoData * > data
int IoCodeToEnumHydrology(int enum_in)
void InputCreate(IssmDouble *vector, Inputs2 *inputs2, IoModel *iomodel, int M, int N, int vector_type, int vector_enum, int code)
void AddConstant(IoConstant *constant_in)
int IoCodeToEnumNature(int enum_in)
int * elementtoverticaledgeconnectivity
void AddDataIndependent(IoData *data_in)
int * elementtoedgeconnectivity
void FindConstant(bool *pvalue, const char *constant_name)
fpos_t * SetFilePointersToData(int **pcodes, int **pvector_types, int *pnum_instances, const char *data_name)
int IoCodeToEnumSMB(int enum_in)
void FetchData(bool *pboolean, const char *data_name)
int numberofhorizontaledges
void FetchIndependentConstant(int *pXcount, IssmPDouble *X, const char *name)
int ISSM_MPI_Bcast(void *buffer, int count, ISSM_MPI_Datatype datatype, int root, ISSM_MPI_Comm comm)
int StringToEnumx(const char *string_in, bool notfounderror=true)
int * singlenodetoelementconnectivity
virtual void SetElementInput(int enum_in, IssmDouble values)
void FetchIndependentData(int *pXcount, IssmPDouble *X, const char *name)
virtual void DatasetInputCreate(IssmDouble *array, int M, int N, int *individual_enums, int num_inputs, Inputs2 *inputs2, IoModel *iomodel, int input_enum)
#define _error_(StreamArgs)
int IoCodeToEnumFrontalforcings(int enum_in)
void StartTrace(bool trace)
int numberofverticalfaces
int numberofverticaledges
FILE * SetFilePointerToData(int *pcode, int *pvector_type, const char *data_name)
void AddData(IoData *data_in)
T * xMemCpy(T *dest, const T *src, unsigned int size)
int * elementtoverticalfaceconnectivity
int IoCodeToEnumTimestepping(int enum_in)
int AddOption(Option *in_oobject)
#define ISSM_MPI_LONG_LONG_INT
void FetchConstants(void)
int IoCodeToEnumAmr(int enum_in)
int IoCodeToEnumBasal(int enum_in)
virtual void GetParameterValue(bool *pbool)=0
int IoCodeToEnumCalving(int enum_in)