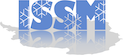 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
23 #include "../../shared/Exceptions/exceptions.h"
24 #include "../../shared/Enum/Enum.h"
35 Seg(
int seg_id,
int seg_sid,
int seg_lid,
IoModel* iomodel,
int nummodels);
40 void Marshall(
char** pmarshalled_data,
int* pmarshalled_data_size,
int marshall_direction);
173 #ifdef _HAVE_SEALEVELRISE_
184 void InputUpdateFromMatrixDakota(
IssmDouble* matrix,
int nows,
int ncols,
int name,
int type){
_error_(
"not implemented yet");};
185 void InputUpdateFromVectorDakota(
IssmDouble* vector,
int name,
int type){
_error_(
"not implemented yet");};
void ElementSizes(IssmDouble *hx, IssmDouble *hy, IssmDouble *hz)
Declaration of Vertices class.
void ComputeStressTensor()
Gauss * NewGauss(int point1, IssmDouble fraction1, IssmDouble fraction2, int order)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
Declaration of Nodes class.
void ValueP1DerivativesOnGauss(IssmDouble *dvalue, IssmDouble *values, IssmDouble *xyz_list, Gauss *gauss)
void NormalSection(IssmDouble *normal, IssmDouble *xyz_list)
bool IsFaceOnBoundary(void)
void SetControlInputsFromVector(IssmDouble *vector, int control_enum, int control_index)
void Update(Inputs2 *inputs2, int index, IoModel *iomodel, int analysis_counter, int analysis_type, int finitelement)
void GetVectorFromControlInputs(Vector< IssmDouble > *gradient, int control_enum, int control_index, const char *data, int offset)
void NodalFunctionsPressure(IssmDouble *basis, Gauss *gauss)
Declaration of Parameters class.
int NumberofNodesPressure(void)
Gauss * NewGauss(IssmDouble *xyz_list, IssmDouble *xyz_list_front, int order_horiz, int order_vert)
void NormalTop(IssmDouble *normal, IssmDouble *xyz_list)
void GetVectorFromControlInputs(Vector< IssmDouble > *gradient, int control_enum, int control_index, const char *data)
Declaration of Elements class.
void NodalFunctionsP2(IssmDouble *basis, Gauss *gauss)
void NormalBase(IssmDouble *normal, IssmDouble *xyz_list)
void StressIntensityFactor(void)
void UpdateConstraintsExtrudeFromBase()
void NodalFunctionsDerivatives(IssmDouble *dbasis, IssmDouble *xyz_list, Gauss *gauss)
void VerticalSegmentIndicesBase(int **pindices, int *pnumseg)
Gauss * NewGaussLine(int vertex1, int vertex2, int order)
Gauss * NewGauss(int point1, IssmDouble fraction1, IssmDouble fraction2, bool mainlyfloating, int order)
IssmDouble GetGroundedPortion(IssmDouble *xyz_list)
IssmDouble Misfit(int modelenum, int observationenum, int weightsenum)
int NodalValue(IssmDouble *pvalue, int index, int natureofdataenum)
IssmDouble MinEdgeLength(IssmDouble *xyz_list)
abstract class for Element object This class is a place holder for the Tria and the Penta elements....
void NodalFunctionsVelocity(IssmDouble *basis, Gauss *gauss)
void NodalFunctions(IssmDouble *basis, Gauss *gauss)
abstract class for handling Seg oriented routines, like nodal functions, strain rate generation,...
void NodalFunctionsTensor(IssmDouble *basis, Gauss *gauss)
Gauss * NewGaussBase(int order)
void GetLevelsetPositivePart(int *point1, IssmDouble *fraction1, IssmDouble *fraction2, bool *mainlynegative, IssmDouble *levelsetvalues)
Declaration of Materials class.
void ControlInputSetGradient(IssmDouble *gradient, int enum_type, int control_index)
int NumberofNodesVelocity(void)
IssmDouble CharacteristicLength(void)
void NodalFunctionsP1(IssmDouble *basis, Gauss *gauss)
void StabilizationParameterAnisotropic(IssmDouble *tau_parameter_anisotropic, IssmDouble u, IssmDouble v, IssmDouble w, IssmDouble hx, IssmDouble hy, IssmDouble hz, IssmDouble kappa)
IssmDouble GroundedArea(bool scaled)
int GetNumberOfNodes(void)
IssmDouble GetAreaSpherical(void)
int PressureInterpolation(void)
void VerticalSegmentIndices(int **pindices, int *pnumseg)
int GetNumberOfNodes(int enum_type)
IssmDouble GetArea3D(void)
int VertexConnectivity(int vertexindex)
void MaterialUpdateFromTemperature(void)
void UpdateConstraintsExtrudeFromTop()
void GetVerticesCoordinatesBase(IssmDouble **pxyz_list)
void GetIcefrontCoordinates(IssmDouble **pxyz_front, IssmDouble *xyz_list, int levelsetenum)
void SetCurrentConfiguration(Elements *elements, Loads *loads, Nodes *nodes, Materials *materials, Parameters *parameters)
int UpdatePotentialUngrounding(IssmDouble *vertices_potentially_ungrounding, Vector< IssmDouble > *vec_nodes_on_iceshelf, IssmDouble *nodes_on_iceshelf)
void GetLevelCoordinates(IssmDouble **pxyz_front, IssmDouble *xyz_list, int levelsetenum, IssmDouble level)
void GetGroundedPart(int *point1, IssmDouble *fraction1, IssmDouble *fraction2, bool *mainlyfloating)
IssmDouble FloatingArea(bool scaled)
void ResetFSBasalBoundaryCondition(void)
IssmDouble TotalFloatingBmb(bool scaled)
void CalvingRateLevermann(void)
void ValueP1OnGauss(IssmDouble *pvalue, IssmDouble *values, Gauss *gauss)
void GetInputListOnVertices(IssmDouble *pvalue, Input2 *input, IssmDouble default_value)
IssmDouble TotalSmb(bool scaled)
void SetTemporaryElementType(int element_type_in)
bool IsNodeOnShelfFromFlags(IssmDouble *flags)
IssmDouble MassFlux(IssmDouble x1, IssmDouble y1, IssmDouble x2, IssmDouble y2, int segment_id)
Declaration of Loads class.
IssmDouble MisfitArea(int weightsenum)
void StrainRateparallel(void)
IssmDouble TotalGroundedBmb(bool scaled)
#define _error_(StreamArgs)
IssmDouble IceVolumeAboveFloatation(bool scaled)
void NodalFunctionsDerivativesVelocity(IssmDouble *dbasis, IssmDouble *xyz_list, Gauss *gauss)
IssmDouble IceVolume(bool scaled)
void ControlToVectors(Vector< IssmPDouble > *vector_control, Vector< IssmPDouble > *vector_gradient, int control_enum)
Gauss * NewGaussTop(int order)
void SetControlInputsFromVector(IssmDouble *vector, int control_enum, int control_index, int offset, int N, int M)
void JacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, Gauss *gauss)
void NodalFunctionsMINIDerivatives(IssmDouble *dbasis, IssmDouble *xyz_list, Gauss *gauss)
int TensorInterpolation(void)
void ReduceMatrices(ElementMatrix *Ke, ElementVector *pe)
void InputUpdateFromVector(IssmDouble *vector, int name, int type)
Element * GetBasalElement(void)
void PotentialUngrounding(Vector< IssmDouble > *potential_sheet_ungrounding)
void JacobianDeterminantBase(IssmDouble *pJdet, IssmDouble *xyz_list_base, Gauss *gauss)
void InputUpdateFromIoModel(int index, IoModel *iomodel)
void GetVerticesCoordinates(IssmDouble **pxyz_list)
Input2 * GetInput2(int enumtype)
void GetVerticesCoordinatesTop(IssmDouble **pxyz_list)
void JacobianDeterminantTop(IssmDouble *pJdet, IssmDouble *xyz_list_base, Gauss *gauss)
void InputUpdateFromSolutionOneDof(IssmDouble *solution, int inputenum)
void NodalFunctionsP1Derivatives(IssmDouble *dbasis, IssmDouble *xyz_list, Gauss *gauss)
void JacobianDeterminantSurface(IssmDouble *pJdet, IssmDouble *xyz_list, Gauss *gauss)
Element * SpawnBasalElement(void)
void InputDepthAverageAtBase(int enum_type, int average_enum_type)
void GetInputListOnNodes(IssmDouble *pvalue, Input2 *input, IssmDouble default_value)
void JacobianDeterminantLine(IssmDouble *Jdet, IssmDouble *xyz_list, Gauss *gauss)
Element * SpawnTopElement(void)
void ElementResponse(IssmDouble *presponse, int response_enum)
void InputUpdateFromSolutionOneDofCollapsed(IssmDouble *solution, int inputenum)
void ControlInputSetGradient(IssmDouble *gradient, int enum_type, int control_index, int offset, int N, int M)
IssmDouble SurfaceArea(void)
void FSContactMigration(Vector< IssmDouble > *vertexgrounded, Vector< IssmDouble > *vertexfloating)
IssmDouble Masscon(IssmDouble *levelset)
Declaration of Results class.
prototypes for ElementHook.h
IssmDouble MassFlux(IssmDouble *segment)
void ComputeDeviatoricStressTensor()
void AverageOntoPartition(Vector< IssmDouble > *partition_contributions, Vector< IssmDouble > *partition_areas, IssmDouble *vertex_response, IssmDouble *qmu_part)
IssmDouble StabilizationParameter(IssmDouble u, IssmDouble v, IssmDouble w, IssmDouble diameter, IssmDouble kappa)
void StrainRateperpendicular(void)
int GetNumberOfVertices(void)
int VelocityInterpolation(void)
void InputExtrude(int enum_type, int start)
void ComputeEsaStrainAndVorticity()
void GetInputValue(IssmDouble *pvalue, Vertex *vertex, int enumtype)
Input2 * GetInput2(int inputenum, IssmDouble start_time, IssmDouble end_time, int averaging_method)
void Configure(Elements *elements, Loads *loads, Nodes *nodesin, Vertices *verticesin, Materials *materials, Parameters *parameters, Inputs2 *inputs2in)
bool IsZeroLevelset(int levelset_enum)