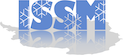 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
12 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
16 #include "../../shared/Numerics/types.h"
18 #if defined(_HAVE_MPI_)
20 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
21 #if defined(_HAVE_ADJOINTMPI_)
22 #include <ampi_tape.hpp>
24 #elif defined(_HAVE_MEDIPACK_)
25 #include "medi/medi.hpp"
27 #if defined(_HAVE_CODIPACK_)
34 #include <codi/externals/codiMpiTypes.hpp>
35 using MpiTypes = CoDiMpiTypes<IssmDouble>;
36 extern MpiTypes* mpiTypes;
37 #define AMPI_ADOUBLE mpiTypes->MPI_TYPE
38 #elif defined(_HAVE_ADOLC_)
39 #include "adolc/medipacksupport.h"
40 #define TOOL AdolcTool
42 #error "don't know about AD tool"
46 #include <ampi/ampi.h>
48 #elif _HAVE_PETSC_MPI_ // PETSc now hides their MPI header. It can be reached through PETSc's header file.
57 #if defined(_HAVE_MEDIPACK_) && !defined(_WRAPPERS_)
69 #if defined(_HAVE_MEDIPACK_) && !defined(_WRAPPERS_)
70 #define ISSM_MPI_CHAR AMPI_CHAR
71 #define ISSM_MPI_DOUBLE AMPI_ADOUBLE // corresponds to IssmDouble
72 #define ISSM_MPI_PDOUBLE AMPI_DOUBLE // corresponds to IssmPDouble
73 #define ISSM_MPI_INT AMPI_INT
74 #define ISSM_MPI_LONG_LONG_INT AMPI_LONG_LONG_INT
77 #define ISSM_MPI_MAX AMPI_MAX
78 #define ISSM_MPI_MIN AMPI_MIN
79 #define ISSM_MPI_PROD AMPI_PROD
80 #define ISSM_MPI_SUM AMPI_SUM
83 #define ISSM_MPI_COMM_WORLD AMPI_COMM_WORLD
84 #define ISSM_MPI_STATUS_IGNORE AMPI_STATUS_IGNORE
85 #define ISSM_MPI_ANY_TAG AMPI_ANY_TAG
86 #define ISSM_MPI_ANY_SOURCE AMPI_ANY_SOURCE
89 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
90 #define ISSM_MPI_DOUBLE AMPI_ADOUBLE
92 #define ISSM_MPI_DOUBLE MPI_DOUBLE
94 #define ISSM_MPI_PDOUBLE MPI_DOUBLE
95 #define ISSM_MPI_INT MPI_INT
96 #define ISSM_MPI_LONG_LONG_INT MPI_LONG_LONG_INT
97 #define ISSM_MPI_CHAR MPI_CHAR
100 #define ISSM_MPI_MAX MPI_MAX
101 #define ISSM_MPI_MIN MPI_MIN
102 #define ISSM_MPI_PROD MPI_PROD
103 #define ISSM_MPI_SUM MPI_SUM
106 #define ISSM_MPI_COMM_WORLD MPI_COMM_WORLD
107 #define ISSM_MPI_STATUS_IGNORE MPI_STATUS_IGNORE
108 #define ISSM_MPI_ANY_TAG MPI_ANY_TAG
109 #define ISSM_MPI_ANY_SOURCE MPI_ANY_SOURCE
124 #define ISSM_MPI_CHAR 1
125 #define ISSM_MPI_DOUBLE 2
126 #define ISSM_MPI_PDOUBLE 3
127 #define ISSM_MPI_INT 4
128 #define ISSM_MPI_LONG_LONG_INT 5
131 #define ISSM_MPI_MAX 1
132 #define ISSM_MPI_MIN 2
133 #define ISSM_MPI_PROD 3
134 #define ISSM_MPI_SUM 4
137 #define ISSM_MPI_COMM_WORLD 1
139 #define ISSM_MPI_STATUS_IGNORE &ourIssmMPIStatusIgnore
140 #define ISSM_MPI_ANY_TAG 2
141 #define ISSM_MPI_ANY_SOURCE 3
148 #if defined(_HAVE_AD_) && !defined(_WRAPPERS_)
163 rc=AMPI_Bcast(buffer,
208 #endif //#ifndef _ISSM_MPI_H_
ISSM_MPI_Datatype TypeToMPIType< int >()
int ISSM_MPI_Allgatherv(void *sendbuf, int sendcount, ISSM_MPI_Datatype sendtype, void *recvbuf, int *recvcounts, int *displs, ISSM_MPI_Datatype recvtype, ISSM_MPI_Comm comm)
int ISSM_MPI_Comm_free(ISSM_MPI_Comm *comm)
int ISSM_MPI_Comm_split(ISSM_MPI_Comm comm, int color, int key, ISSM_MPI_Comm *newcomm)
void ISSM_MPI_ContiguousInAdolc(size_t aSize)
ISSM_MPI_Datatype TypeToMPIType()
int ISSM_MPI_Recv(void *buf, int count, ISSM_MPI_Datatype datatype, int source, int tag, ISSM_MPI_Comm comm, ISSM_MPI_Status *status)
int ISSM_MPI_Comm_rank(ISSM_MPI_Comm comm, int *rank)
int ISSM_MPI_Intercomm_create(ISSM_MPI_Comm comm, int local_leader, ISSM_MPI_Comm peer_comm, int remote_leader, int tag, ISSM_MPI_Comm *newintercomm)
int ISSM_MPI_Send(void *buf, int count, ISSM_MPI_Datatype datatype, int dest, int tag, ISSM_MPI_Comm comm)
int ISSM_MPI_Reduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Scatterv(void *sendbuf, int *sendcnts, int *displs, ISSM_MPI_Datatype sendtype, void *recvbuf, int recvcnt, ISSM_MPI_Datatype recvtype, int root, ISSM_MPI_Comm comm)
ISSM_MPI_Datatype TypeToMPIType< char >()
int ISSM_MPI_Scatter(void *sendbuf, int sendcnt, ISSM_MPI_Datatype sendtype, void *recvbuf, int recvcnt, ISSM_MPI_Datatype recvtype, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Gatherv(void *sendbuf, int sendcnt, ISSM_MPI_Datatype sendtype, void *recvbuf, int *recvcnts, int *displs, ISSM_MPI_Datatype recvtype, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Finalize(void)
int ISSM_MPI_Allgather(void *sendbuf, int sendcount, ISSM_MPI_Datatype sendtype, void *recvbuf, int recvcount, ISSM_MPI_Datatype recvtype, ISSM_MPI_Comm comm)
int ISSM_MPI_Comm_size(ISSM_MPI_Comm comm, int *size)
int ISSM_MPI_Init(int *argc, char ***argv)
int ISSM_MPI_Allreduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, ISSM_MPI_Comm comm)
double ISSM_MPI_Wtime(void)
int ISSM_MPI_Bcast(T *buffer, int count, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Barrier(ISSM_MPI_Comm comm)
ISSM_MPI_Status ourIssmMPIStatusIgnore
int ISSM_MPI_Gather(void *sendbuf, int sendcnt, ISSM_MPI_Datatype sendtype, void *recvbuf, int recvcnt, ISSM_MPI_Datatype recvtype, int root, ISSM_MPI_Comm comm)
ISSM_MPI_Datatype TypeToMPIType< IssmDouble >()