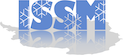 |
Ice Sheet System Model
4.18
Code documentation
|
: numerical core of la solutions
More...
Go to the source code of this file.
: numerical core of la solutions
Definition in file solutionsequence_la.cpp.
◆ solutionsequence_la()
void solutionsequence_la |
( |
FemModel * |
femmodel | ) |
|
Definition at line 10 of file solutionsequence_la.cpp.
23 int max_nonlinear_iterations;
24 bool vel_converged =
false;
25 bool pressure_converged =
false;
53 delete pug_old;pug_old=pug;
54 pressure_converged=
false; vel_converged=
false;
58 delete vel_old_local;vel_old_local=vel;
69 delete Kff;
delete pf;
delete df;
79 if (xIsNan<IssmDouble>(ndu) || xIsNan<IssmDouble>(nu))
_error_(
"convergence criterion is NaN!");
81 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" < " << eps_rel*100 <<
" %\n");
85 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" > " << eps_rel*100 <<
" %\n");
87 if(count_local>=max_nonlinear_iterations){
88 _printf0_(
" maximum number of nonlinear iterations (" << max_nonlinear_iterations <<
") exceeded\n");
103 delete Kff;
delete pf;
delete df;
114 if (xIsNan<IssmDouble>(ndu) || xIsNan<IssmDouble>(nu))
_error_(
"convergence criterion is NaN!");
115 if((ndu/nu)<eps_rel){
116 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" < " << eps_rel*100 <<
" %\n");
120 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(du)/norm(u)" << ndu/nu*100 <<
" > " << eps_rel*100 <<
" %\n");
126 if (xIsNan<IssmDouble>(ndp) || xIsNan<IssmDouble>(np))
_error_(
"convergence criterion is NaN!");
127 if((ndp/np)<eps_rel){
128 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(dp)/norm(p)" << ndp/np*100 <<
" < " << eps_rel*100 <<
" %\n");
129 pressure_converged=
true;
132 if(
VerboseConvergence())
_printf0_(setw(50) << left <<
" Convergence criterion: norm(dp/)/norm(p)" << ndp/np*100 <<
" > " << eps_rel*100 <<
" %\n");
133 pressure_converged=
false;
136 if(pressure_converged && vel_converged)
break;
137 if(count>=max_nonlinear_iterations){
138 _printf0_(
" maximum number of nonlinear iterations (" << max_nonlinear_iterations <<
") exceeded\n");
149 delete vel_old_local;
150 delete stressanalysis;
151 delete pressureanalysis;
void Mergesolutionfromftogx(Vector< IssmDouble > **pug, Vector< IssmDouble > *uf, Vector< IssmDouble > *ys, Nodes *nodes, Parameters *parameters, bool flag_ys0)
#define _printf0_(StreamArgs)
@ StressbalanceAnalysisEnum
bool VerboseConvergence(void)
void GetVectorFromInputsx(IssmDouble **pvector, int *pvector_size, FemModel *femmodel, int name)
void Solverx(Vector< IssmDouble > **puf, Matrix< IssmDouble > *Kff, Vector< IssmDouble > *pf, Vector< IssmDouble > *uf0, Vector< IssmDouble > *df, Parameters *parameters)
Vector< doubletype > * Duplicate(void)
@ StressbalanceMaxiterEnum
doubletype Norm(NormMode norm_type)
@ UzawaPressureAnalysisEnum
void UpdateConstraintsx(void)
@ StressbalanceReltolEnum
void Stop(int tagenum, bool dontmpisync=true)
void Reduceloadx(Vector< IssmDouble > *pf, Matrix< IssmDouble > *Kfs, Vector< IssmDouble > *y_s, bool flag_ys0)
void AYPX(Vector *X, doubletype a)
#define _error_(StreamArgs)
void Start(int tagenum, bool dontmpisync=true)
void SetCurrentConfiguration(int configuration_type)
void FindParam(bool *pinteger, int enum_type)
void CreateNodalConstraintsx(Vector< IssmDouble > **pys, Nodes *nodes)
void SystemMatricesx(Matrix< IssmDouble > **pKff, Matrix< IssmDouble > **pKfs, Vector< IssmDouble > **ppf, Vector< IssmDouble > **pdf, IssmDouble *pkmax, FemModel *femmodel, bool isAllocated)