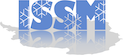 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
6 #include "../shared/shared.h"
10 template <
class R,
class RR>
class P2{
22 printf(
"Member of P2:\n");
23 std::cout<<
" x: "<<
x<<std::endl;
24 std::cout<<
" y: "<<
y<<std::endl;
40 template <
class R,
class RR>
class P2xP2{
53 printf(
"Member of P2xP2:\n");
54 printf(
" x.x: %g x.y: %g\n",
x.x,
x.y);
55 printf(
" y.x: %g y.x: %g\n",
y.x,
y.y);
57 RR
det()
const {
return (RR)
x.x* (RR)
y.y - (RR)
x.y * (RR)
y.x;}
69 x.x*c.
x.y +
x.y*c.
y.y,
70 y.x*c.
x.x +
y.y*c.
y.x,
71 y.x*c.
x.y +
y.y*c.
y.y);
76 template <
class R,
class RR>
78 return (RR) x.
x * (RR) y.
y - (RR) x.
y * (RR) y.
x ;
80 template <
class R,
class RR>
84 template <
class R,
class RR>
88 template <
class R,
class RR>
90 return (RR)x.
x*(RR)x.
x + (RR)x.
y*(RR)x.
y ;
92 template <
class R,
class RR>
94 return sqrt((RR)x.
x*(RR)x.
x + (RR)x.
y*(RR)x.
y) ;
96 template <
class R,
class RR>
P2< R, RR > operator+=(const P2< R, RR > &cc)
RR operator,(const P2< R, RR > &cc) const
P2< R, RR > operator-() const
P2< R, RR > operator-=(const P2< R, RR > &cc)
P2xP2(P2< R, RR > a, P2< R, RR > b)
P2< R, RR > operator/=(const R r)
P2< R, RR > operator*(const P2< R, RR > &c) const
P2xP2< R, RR > inv() const
RR Area2(const P2< R, RR > a, const P2< R, RR > b, const P2< R, RR > c)
RR Norme2(const P2< R, RR > x)
P2< R, RR > operator*(R cc) const
RR Det(const P2< R, RR > x, const P2< R, RR > y)
P2xP2< R, RR > operator*(P2xP2< R, RR > c) const
P2xP2(R xx, R xy, R yx, R yy)
P2< R, RR > operator/(R cc) const
P2< R, RR > operator-(const P2< R, RR > &cc) const
RR Norme2_2(const P2< R, RR > x)
P2xP2(P2< R, RR > a, P2< R, RR > b, P2< R, RR > c)
P2< R, RR > Orthogonal(const P2< R, RR > x)
P2< R, RR > operator+(const P2< R, RR > &cc) const
P2< R, RR > operator*=(const R r)
R Norme1(const P2< R, RR > x)