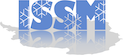 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the source code of this file.
|
void | GroundinglineMigrationx (Elements *elements, Nodes *nodes, Vertices *vertices, Loads *loads, Materials *materials, Parameters *parameters) |
|
IssmDouble * | ContactFSLevelset (Elements *elements, Vertices *vertices) |
|
IssmDouble * | PotentialUngrounding (Elements *elements, Vertices *vertices, Parameters *parameters) |
|
IssmDouble * | PropagateFloatingiceToGroundedNeighbors (Elements *elements, Nodes *nodes, Vertices *vertices, Parameters *parameters, IssmDouble *vertices_potentially_ungrounding) |
|
◆ GroundinglineMigrationx()
Definition at line 10 of file GroundinglineMigrationx.cpp.
12 int migration_style,analysis_type;
13 IssmDouble *vertices_potentially_ungrounding = NULL;
21 if(migration_style==
NoneEnum)
return;
28 switch(migration_style){
48 for(
int i=0;i<elements->
Size();i++){
54 xDelete<IssmDouble>(vertices_potentially_ungrounding);
55 xDelete<IssmDouble>(phi_ungrounding);
◆ ContactFSLevelset()
Definition at line 58 of file GroundinglineMigrationx.cpp.
63 IssmDouble* serial_vertex_waterpressure = NULL;
70 phi = xNew<IssmDouble>(numberofvertices);
73 for(
int i=0;i<elements->
Size();i++){
80 serial_vertex_sigmann=vertex_sigmann->
ToMPISerial();
81 serial_vertex_waterpressure=vertex_waterpressure->
ToMPISerial();
83 for(
int i=0;i<numberofvertices;i++){
84 if (serial_vertex_waterpressure[i] > serial_vertex_sigmann[i]) phi[i]=-1;
89 delete vertex_sigmann;
90 delete vertex_waterpressure;
91 xDelete<IssmDouble>(serial_vertex_sigmann);
92 xDelete<IssmDouble>(serial_vertex_waterpressure);
◆ PotentialUngrounding()
Definition at line 97 of file GroundinglineMigrationx.cpp.
99 int i,numberofvertices;
100 IssmDouble* vertices_potentially_ungrounding = NULL;
109 for(i=0;i<elements->
Size();i++){
115 vec_vertices_potentially_ungrounding->
Assemble();
116 vertices_potentially_ungrounding=vec_vertices_potentially_ungrounding->
ToMPISerial();
119 delete vec_vertices_potentially_ungrounding;
120 return vertices_potentially_ungrounding;
◆ PropagateFloatingiceToGroundedNeighbors()
Definition at line 123 of file GroundinglineMigrationx.cpp.
125 int nflipped,local_nflipped;
127 IssmDouble* elements_neighboring_floatingce = NULL;
137 for(i=0;i<elements->
Size();i++){
151 for(i=0;i<elements->
Size();i++){
157 vec_elements_neighboring_floatingice->
Assemble();
158 elements_neighboring_floatingce=vec_elements_neighboring_floatingice->
ToMPISerial();
162 for(i=0;i<elements->
Size();i++){
164 if(reCast<int,IssmDouble>(elements_neighboring_floatingce[element->
Sid()])){
174 xDelete<IssmDouble>(elements_neighboring_floatingce);
175 xDelete<IssmDouble>(phi);
178 delete vec_elements_neighboring_floatingice;
184 xDelete<IssmDouble>(elements_neighboring_floatingce);
IssmDouble * ContactFSLevelset(Elements *elements, Vertices *vertices)
@ GroundinglineMigrationEnum
#define _printf0_(StreamArgs)
int ISSM_MPI_Allreduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, ISSM_MPI_Comm comm)
bool VerboseConvergence(void)
static ISSM_MPI_Comm GetComm(void)
virtual void PotentialUngrounding(Vector< IssmDouble > *potential_sheet_ungrounding)=0
void ToolkitsOptionsFromAnalysis(Parameters *parameters, int analysis_type)
int NumberOfVertices(void)
virtual bool IsNodeOnShelfFromFlags(IssmDouble *flags)=0
IssmDouble * PotentialUngrounding(Elements *elements, Vertices *vertices, Parameters *parameters)
const char * EnumToStringx(int enum_in)
void GetVectorFromInputs(Vector< IssmDouble > *vector, int name_enum, int type)
IssmDouble * PropagateFloatingiceToGroundedNeighbors(Elements *elements, Nodes *nodes, Vertices *vertices, Parameters *parameters, IssmDouble *vertices_potentially_ungrounding)
void MigrateGroundingLine(IssmDouble *sheet_ungrounding)
#define _error_(StreamArgs)
Object * GetObjectByOffset(int offset)
void FindParam(bool *pinteger, int enum_type)
int NumberOfElements(void)
@ SubelementMigrationEnum
doubletype * ToMPISerial(void)
@ AggressiveMigrationEnum
void SetValue(int dof, doubletype value, InsMode mode)
virtual void FSContactMigration(Vector< IssmDouble > *vertex_sigmann, Vector< IssmDouble > *vertex_waterpressure)=0
virtual int UpdatePotentialUngrounding(IssmDouble *potential_sheet_ungrounding, Vector< IssmDouble > *vec_nodes_on_iceshelf, IssmDouble *nodes_on_iceshelf)=0