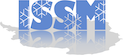 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
9 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
17 #if defined(_HAVE_MPI_) && defined(_HAVE_CODIPACK_)
21 #include "../../shared/Numerics/types.h"
32 return sizeof(double);
47 assert(sendcount==recvcount || sendtype==recvtype);
49 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
50 rc=AMPI_Allgather(sendbuf,
58 rc=MPI_Allgather(sendbuf,
71 for(
int i=0;i<sendcount;++i) activeRecvBuf[i]=activeSendBuf[i];
75 memcpy(recvbuf,sendbuf,
sizeHelper(sendtype)*sendcount);
81 assert(sendtype==recvtype);
83 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
84 rc=AMPI_Allgatherv(sendbuf,
93 rc=MPI_Allgatherv(sendbuf,
103 assert(sendcount==recvcounts[0]);
108 for(
int i=0;i<sendcount;++i) activeRecvBuf[i]=activeSendBuf[i];
120 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
121 rc=AMPI_Allreduce(sendbuf,
128 rc=MPI_Allreduce(sendbuf,
140 for(
int i=0;i<count;++i) activeRecvBuf[i]=activeSendBuf[i];
144 memcpy(recvbuf,sendbuf,
sizeHelper(datatype)*count);
152 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
153 rc=AMPI_Barrier(comm);
155 rc=MPI_Barrier(comm);
166 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
167 rc=AMPI_Bcast(buffer,
188 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
191 rc=MPI_Comm_free(comm);
202 rc=MPI_Comm_rank(comm,
213 rc=MPI_Comm_size(comm,
224 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
225 #if defined(_HAVE_ADJOINTMPI_)
227 #elif defined(_HAVE_MEDIPACK_)
234 rc=AMPI_Finalize_NT();
245 assert(sendtype==recvtype && sendcnt==recvcnt);
247 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
248 rc=AMPI_Gather(sendbuf,
257 rc=MPI_Gather(sendbuf,
271 for(
int i=0;i<sendcnt;++i) activeRecvBuf[i]=activeSendBuf[i];
275 memcpy(recvbuf,sendbuf,
sizeHelper(sendtype)*sendcnt);
282 assert(sendtype==recvtype);
284 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
285 rc=AMPI_Gatherv(sendbuf,
295 rc=MPI_Gatherv(sendbuf,
306 assert(sendcnt==recvcnts[0]);
311 for(
int i=0;i<sendcnt;++i) activeRecvBuf[i]=activeSendBuf[i];
323 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
324 #if defined(_HAVE_ADJOINTMPI_)
325 rc=AMPI_Init(argc,argv);
326 #elif defined(_HAVE_MEDIPACK_)
327 rc=AMPI_Init(argc,argv);
332 mpiTypes =
new MpiTypes();
334 rc=AMPI_Init_NT(argc,argv);
337 rc=MPI_Init(argc,argv);
346 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
352 #
if !defined(_HAVE_ADJOINTMPI_) && !defined(_HAVE_MEDIPACK_)
377 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
378 rc=AMPI_Reduce(sendbuf,
386 rc=MPI_Reduce(sendbuf,
399 for(
int i=0;i<count;++i) activeRecvBuf[i]=activeSendBuf[i];
403 memcpy(recvbuf,sendbuf,
sizeHelper(datatype)*count);
410 assert(sendtype==recvtype && sendcnt==recvcnt);
412 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
413 rc=AMPI_Scatter(sendbuf,
422 rc=MPI_Scatter(sendbuf,
436 for(
int i=0;i<recvcnt;++i) activeRecvBuf[i]=activeSendBuf[i];
440 memcpy(recvbuf,sendbuf,
sizeHelper(sendtype)*recvcnt);
447 assert(sendtype==recvtype);
449 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
450 rc=AMPI_Scatterv(sendbuf,
460 rc=MPI_Scatterv(sendbuf,
471 assert(sendcnts[0]==recvcnt);
476 for(
int i=0;i<recvcnt;++i) activeRecvBuf[i]=activeSendBuf[i];
488 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
494 #
if !defined(_HAVE_ADJOINTMPI_) && !defined(_HAVE_MEDIPACK_)
523 ensureContiguousLocations(aSize);
525 fprintf(stderr,
"*** Codipack ISSM_MPI_ContiguousInAdolc()\n");
532 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
533 rc=MPI_Comm_split(comm, color, key, newcomm);
535 rc=MPI_Comm_split(comm, color, key, newcomm);
546 #if defined(_HAVE_AMPI_) && !defined(_WRAPPERS_)
547 rc=MPI_Intercomm_create(comm,local_leader,peer_comm,remote_leader,tag,newintercomm);
549 rc=MPI_Intercomm_create(comm,local_leader,peer_comm,remote_leader,tag,newintercomm);
int ISSM_MPI_Comm_split(ISSM_MPI_Comm comm, int color, int key, ISSM_MPI_Comm *newcomm)
int ISSM_MPI_Allreduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, ISSM_MPI_Comm comm)
int ISSM_MPI_Gatherv(void *sendbuf, int sendcnt, ISSM_MPI_Datatype sendtype, void *recvbuf, int *recvcnts, int *displs, ISSM_MPI_Datatype recvtype, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Comm_free(ISSM_MPI_Comm *comm)
int ISSM_MPI_Comm_rank(ISSM_MPI_Comm comm, int *rank)
int ISSM_MPI_Finalize(void)
int ISSM_MPI_Send(void *buf, int count, ISSM_MPI_Datatype datatype, int dest, int tag, ISSM_MPI_Comm comm)
void ISSM_MPI_ContiguousInAdolc(size_t aSize)
int ISSM_MPI_Intercomm_create(ISSM_MPI_Comm comm, int local_leader, ISSM_MPI_Comm peer_comm, int remote_leader, int tag, ISSM_MPI_Comm *newintercomm)
int ISSM_MPI_Init(int *argc, char ***argv)
int ISSM_MPI_Bcast(void *buffer, int count, ISSM_MPI_Datatype datatype, int root, ISSM_MPI_Comm comm)
size_t sizeHelper(ISSM_MPI_Datatype type)
int ISSM_MPI_Allgather(void *sendbuf, int sendcount, ISSM_MPI_Datatype sendtype, void *recvbuf, int recvcount, ISSM_MPI_Datatype recvtype, ISSM_MPI_Comm comm)
double ISSM_MPI_Wtime(void)
int ISSM_MPI_Allgatherv(void *sendbuf, int sendcount, ISSM_MPI_Datatype sendtype, void *recvbuf, int *recvcounts, int *displs, ISSM_MPI_Datatype recvtype, ISSM_MPI_Comm comm)
int ISSM_MPI_Barrier(ISSM_MPI_Comm comm)
int ISSM_MPI_Recv(void *buf, int count, ISSM_MPI_Datatype datatype, int source, int tag, ISSM_MPI_Comm comm, ISSM_MPI_Status *status)
ISSM_MPI_Status ourIssmMPIStatusIgnore
int ISSM_MPI_Reduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Scatter(void *sendbuf, int sendcnt, ISSM_MPI_Datatype sendtype, void *recvbuf, int recvcnt, ISSM_MPI_Datatype recvtype, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Gather(void *sendbuf, int sendcnt, ISSM_MPI_Datatype sendtype, void *recvbuf, int recvcnt, ISSM_MPI_Datatype recvtype, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Scatterv(void *sendbuf, int *sendcnts, int *displs, ISSM_MPI_Datatype sendtype, void *recvbuf, int recvcnt, ISSM_MPI_Datatype recvtype, int root, ISSM_MPI_Comm comm)
int ISSM_MPI_Comm_size(ISSM_MPI_Comm comm, int *size)