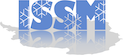 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
10 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
15 #include "../Params/Parameters.h"
16 #include "../ExternalResults/Results.h"
17 #include "../ExternalResults/GenericExternalResult.h"
18 #include "../../toolkits/toolkits.h"
19 #include "../../shared/shared.h"
38 vector<Object*>::iterator object;
40 for(
object=objects.begin() ;
object < objects.end();
object++ ){
41 Element* element=xDynamicCast<Element*>((*
object));
42 element->
Configure(elements,loads,nodes,vertices,materials,parameters,inputs2);
53 for(
int i=0;i<this->Size();i++){
55 Element* element=xDynamicCast<Element*>(this->GetObjectByOffset(i));
57 if(numnodes>
max)
max=numnodes;
72 local_nelem=this->Size();
75 return numberofelements;
80 vector<Object*>::iterator object;
83 for (
object=objects.begin() ;
object < objects.end();
object++ ){
85 element=xDynamicCast<Element*>((*
object));
94 vector<Object*>::iterator object;
97 for (
object=objects.begin() ;
object < objects.end();
object++ ){
99 element=xDynamicCast<Element*>((*
object));
Declaration of Vertices class.
void Configure(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters, Inputs2 *inputs2)
Declaration of Nodes class.
virtual int GetNumberOfNodes(void)=0
int ISSM_MPI_Allreduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, ISSM_MPI_Comm comm)
Declaration of Parameters class.
static ISSM_MPI_Comm GetComm(void)
Declaration of Elements class.
abstract class for Element object This class is a place holder for the Tria and the Penta elements....
Declaration of Materials class.
virtual void ResetHooks()=0
Declaration of Loads class.
void SetCurrentConfiguration(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters)
virtual void SetCurrentConfiguration(Elements *elements, Loads *loads, Nodes *nodes, Materials *materials, Parameters *parameters)=0
int NumberOfElements(void)
IssmDouble max(IssmDouble a, IssmDouble b)
virtual void Configure(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters, Inputs2 *inputs2in)=0