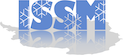 |
Ice Sheet System Model
4.18
Code documentation
|
#include <Channel.h>
|
| Channel () |
|
| Channel (int numericalflux_id, IssmDouble channelarea, int index, IoModel *iomodel) |
|
| ~Channel () |
|
Object * | copy () |
|
void | DeepEcho () |
|
void | Echo () |
|
int | Id () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | ObjectEnum () |
|
void | InputUpdateFromConstant (IssmDouble constant, int name) |
|
void | InputUpdateFromConstant (int constant, int name) |
|
void | InputUpdateFromConstant (bool constant, int name) |
|
void | InputUpdateFromIoModel (int index, IoModel *iomodel) |
|
void | InputUpdateFromMatrixDakota (IssmDouble *matrix, int nrows, int ncols, int name, int type) |
|
void | InputUpdateFromVector (IssmDouble *vector, int name, int type) |
|
void | InputUpdateFromVectorDakota (IssmDouble *vector, int name, int type) |
|
void | Configure (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | CreateJacobianMatrix (Matrix< IssmDouble > *Jff) |
|
void | CreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs) |
|
void | CreatePVector (Vector< IssmDouble > *pf) |
|
void | GetNodesLidList (int *lidlist) |
|
void | GetNodesSidList (int *sidlist) |
|
int | GetNumberOfNodes (void) |
|
bool | IsPenalty (void) |
|
void | PenaltyCreateJacobianMatrix (Matrix< IssmDouble > *Jff, IssmDouble kmax) |
|
void | PenaltyCreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *kfs, IssmDouble kmax) |
|
void | PenaltyCreatePVector (Vector< IssmDouble > *pf, IssmDouble kmax) |
|
void | ResetHooks () |
|
void | SetCurrentConfiguration (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | SetwiseNodeConnectivity (int *d_nz, int *o_nz, Node *node, bool *flags, int *flagsindices, int set1_enum, int set2_enum) |
|
void | SetChannelCrossSectionOld (void) |
|
void | UpdateChannelCrossSection (void) |
|
ElementVector * | CreatePVectorHydrologyGlaDS (void) |
|
ElementMatrix * | CreateKMatrixHydrologyGlaDS (void) |
|
void | WriteChannelCrossSection (IssmPDouble *values) |
|
virtual | ~Load () |
|
virtual | ~Object () |
|
Definition at line 18 of file Channel.h.
◆ Channel() [1/2]
◆ Channel() [2/2]
Channel::Channel |
( |
int |
numericalflux_id, |
|
|
IssmDouble |
channelarea, |
|
|
int |
index, |
|
|
IoModel * |
iomodel |
|
) |
| |
Definition at line 41 of file Channel.cpp.
45 this->
sid=channel_id-1;
53 this->
S = channelarea;
54 this->
Sold = channelarea;
57 int i1 = iomodel->
faces[4*index+0];
58 int i2 = iomodel->
faces[4*index+1];
59 int e1 = iomodel->
faces[4*index+2];
60 int e2 = iomodel->
faces[4*index+3];
73 int channel_vertex_ids[2];
74 channel_vertex_ids[0]=i1;
75 channel_vertex_ids[1]=i2;
79 int channel_node_ids[2];
80 channel_node_ids[0]=i1;
81 channel_node_ids[1]=i2;
◆ ~Channel()
◆ copy()
Object * Channel::copy |
( |
void |
| ) |
|
|
virtual |
◆ DeepEcho()
void Channel::DeepEcho |
( |
void |
| ) |
|
|
virtual |
◆ Echo()
void Channel::Echo |
( |
void |
| ) |
|
|
virtual |
◆ Id()
◆ Marshall()
void Channel::Marshall |
( |
char ** |
pmarshalled_data, |
|
|
int * |
pmarshalled_data_size, |
|
|
int |
marshall_direction |
|
) |
| |
|
virtual |
◆ ObjectEnum()
int Channel::ObjectEnum |
( |
void |
| ) |
|
|
virtual |
◆ InputUpdateFromConstant() [1/3]
void Channel::InputUpdateFromConstant |
( |
IssmDouble |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromConstant() [2/3]
void Channel::InputUpdateFromConstant |
( |
int |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromConstant() [3/3]
void Channel::InputUpdateFromConstant |
( |
bool |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromIoModel()
void Channel::InputUpdateFromIoModel |
( |
int |
index, |
|
|
IoModel * |
iomodel |
|
) |
| |
|
inline |
◆ InputUpdateFromMatrixDakota()
void Channel::InputUpdateFromMatrixDakota |
( |
IssmDouble * |
matrix, |
|
|
int |
nrows, |
|
|
int |
ncols, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ InputUpdateFromVector()
void Channel::InputUpdateFromVector |
( |
IssmDouble * |
vector, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ InputUpdateFromVectorDakota()
void Channel::InputUpdateFromVectorDakota |
( |
IssmDouble * |
vector, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ Configure()
Implements Load.
Definition at line 179 of file Channel.cpp.
193 this->parameters=parametersin;
◆ CreateJacobianMatrix()
◆ CreateKMatrix()
Implements Load.
Definition at line 196 of file Channel.cpp.
203 switch(analysis_type){
208 _error_(
"Don't know why we should be here");
◆ CreatePVector()
Implements Load.
Definition at line 219 of file Channel.cpp.
226 switch(analysis_type){
231 _error_(
"Don't know why we should be here");
◆ GetNodesLidList()
void Channel::GetNodesLidList |
( |
int * |
lidlist | ) |
|
|
virtual |
◆ GetNodesSidList()
void Channel::GetNodesSidList |
( |
int * |
sidlist | ) |
|
|
virtual |
◆ GetNumberOfNodes()
int Channel::GetNumberOfNodes |
( |
void |
| ) |
|
|
virtual |
◆ IsPenalty()
bool Channel::IsPenalty |
( |
void |
| ) |
|
|
virtual |
◆ PenaltyCreateJacobianMatrix()
◆ PenaltyCreateKMatrix()
◆ PenaltyCreatePVector()
◆ ResetHooks()
void Channel::ResetHooks |
( |
| ) |
|
|
virtual |
◆ SetCurrentConfiguration()
◆ SetwiseNodeConnectivity()
void Channel::SetwiseNodeConnectivity |
( |
int * |
d_nz, |
|
|
int * |
o_nz, |
|
|
Node * |
node, |
|
|
bool * |
flags, |
|
|
int * |
flagsindices, |
|
|
int |
set1_enum, |
|
|
int |
set2_enum |
|
) |
| |
|
virtual |
Implements Load.
Definition at line 298 of file Channel.cpp.
307 if(!flags[this->
nodes[i]->Lid()]){
313 while(flagsindices[counter]>=0) counter++;
314 flagsindices[counter]=this->nodes[i]->Lid();
320 if(this->nodes[i]->IsClone())
328 if(this->nodes[i]->IsClone())
336 if(this->nodes[i]->IsClone())
342 default:
_error_(
"not supported");
◆ SetChannelCrossSectionOld()
void Channel::SetChannelCrossSectionOld |
( |
void |
| ) |
|
◆ UpdateChannelCrossSection()
void Channel::UpdateChannelCrossSection |
( |
void |
| ) |
|
Definition at line 601 of file Channel.cpp.
615 IssmDouble H,h,b,dphi[2],dphids,dphimds,db[2],dbds;
641 IssmDouble tx = xyz_list_tria[index2][0] - xyz_list_tria[index1][0];
642 IssmDouble ty = xyz_list_tria[index2][1] - xyz_list_tria[index1][1];
663 phi_0 = rho_water*g*b + rho_ice*g*H;
664 dphids = dphi[0]*tx + dphi[1]*ty;
665 dphimds = rho_water*g*(db[0]*tx + db[1]*ty);
666 Ngrad = fabs(dphids);
682 bool converged =
false;
691 if(this->
S>0. || qc*dPw>0.){
696 fabs(Qprime*pow(Snew,
ALPHA_C-1.)*dphids)
697 + C*Qprime*pow(Snew,
ALPHA_C-1.)*dPw
698 ) - 2./pow(n,n)*A*pow(fabs(N),n-1.)*N;
700 IssmDouble beta = 1./(rho_ice*L)*( fabs(lc*qc*dphids) + C*fFactor*dPw );
703 this->
S =
ODE1(alpha,beta,this->
Sold,dt,2);
704 if(this->
S<0.) this->
S = 0.;
709 if(fabs((this->
S - Snew)/(Snew+
AEPS))<1e-8 || count>=10) converged =
true;
◆ CreatePVectorHydrologyGlaDS()
Definition at line 487 of file Channel.cpp.
498 IssmDouble A,B,n,phi_old,phi,phi_0,dphimds,dphi[2];
499 IssmDouble H,h,b,db[2],dphids,qc,dPw,ks,Ngrad;
529 IssmDouble tx = xyz_list_tria[index2][0] - xyz_list_tria[index1][0];
530 IssmDouble ty = xyz_list_tria[index2][1] - xyz_list_tria[index1][1];
537 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
555 phi_0 = rho_water*g*b + rho_ice*g*H;
556 dphids = dphi[0]*tx + dphi[1]*ty;
557 dphimds = rho_water*g*(db[0]*tx + db[1]*ty);
558 Ngrad = fabs(dphids);
568 dPw = dphids - dphimds;
572 if(this->
S>0. || qc*dPw>0.){
577 Afactor =
C_W*c_t*rho_water;
578 Bfactor = 1./L * (1./rho_ice - 1./rho_water);
583 v2 = 2./pow(n,n)*A*this->
S*(pow(fabs(phi_0 - phi),n-1.)*(phi_0 +(n-1.)*phi));
585 for(
int i=0;i<numnodes;i++){
587 pe->
values[i]+= + Jdet*gauss->
weight*Afactor*Bfactor*fFactor*dphimds*basis[i];
◆ CreateKMatrixHydrologyGlaDS()
Definition at line 354 of file Channel.cpp.
364 IssmDouble Jdet,v1,qc,fFactor,Afactor,Bfactor,Xifactor;
365 IssmDouble A,B,n,phi_old,phi,phi_0,dPw,ks,Ngrad;
366 IssmDouble H,h,b,dphi[2],dphids,dphimds,db[2],dbds;
398 IssmDouble tx = xyz_list_tria[index2][0] - xyz_list_tria[index1][0];
399 IssmDouble ty = xyz_list_tria[index2][1] - xyz_list_tria[index1][1];
406 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
412 dbasisds[0] = dbasisdx[0*2+0]*tx + dbasisdx[0*2+1]*ty;
413 dbasisds[1] = dbasisdx[1*2+0]*tx + dbasisdx[1*2+1]*ty;
427 phi_0 = rho_water*g*b + rho_ice*g*H;
428 dphids = dphi[0]*tx + dphi[1]*ty;
429 dphimds = rho_water*g*(db[0]*tx + db[1]*ty);
430 Ngrad = fabs(dphids);
441 dPw = dphids - dphimds;
445 if(this->
S>0. || qc*dPw>0.){
450 Afactor =
C_W*c_t*rho_water;
451 Bfactor = 1./L * (1./rho_ice - 1./rho_water);
453 Xifactor = + Bfactor * (fabs(-Kc*dphids) + fabs(lc*qc));
456 Xifactor = - Bfactor * (fabs(-Kc*dphids) + fabs(lc*qc));
460 for(
int i=0;i<numnodes;i++){
461 for(
int j=0;j<numnodes;j++){
464 +Kc*dbasisds[i]*dbasisds[j]
465 - Afactor * Bfactor* Kc * dPw * basis[i] * dbasisds[j]
466 + Afactor * fFactor * Bfactor * basis[i] * dbasisds[j]
467 + Xifactor* basis[i] * dbasisds[j]
474 v1 = 2./pow(n,n)*A*
S*(pow(fabs(phi_0 - phi),n-1.)*( - n));
475 for(
int i=0;i<numnodes;i++){
476 for(
int j=0;j<numnodes;j++){
477 Ke->
values[i*numnodes+j] += gauss->
weight*Jdet*(-v1)*basis[i]*basis[j];
◆ WriteChannelCrossSection()
void Channel::WriteChannelCrossSection |
( |
IssmPDouble * |
values | ) |
|
Definition at line 716 of file Channel.cpp.
719 values[this->
sid] = reCast<IssmPDouble>(this->
S);
◆ Sold
◆ boundary
◆ sid
◆ id
◆ helement
◆ hnodes
◆ hvertices
◆ element
◆ vertices
◆ nodes
◆ parameters
The documentation for this class was generated from the following files:
int GetNumberOfNodes(void)
void FindParam(bool *pvalue, int paramenum)
ElementVector * CreatePVectorHydrologyGlaDS(void)
void GetSegmentJacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, Gauss *gauss)
#define _printf_(StreamArgs)
ElementMatrix * CreateKMatrixHydrologyGlaDS(void)
@ MaterialsRhoFreshwaterEnum
#define MARSHALLING_ENUM(EN)
@ TimesteppingTimeStepEnum
@ HydrologyPressureMeltCoefficientEnum
@ MaterialsLatentheatEnum
void GetSegmentNodalFunctions(IssmDouble *basis, Gauss *gauss, int index1, int index2, int finiteelement)
virtual Input2 * GetInput2(int inputenum)=0
@ HydrologyGlaDSAnalysisEnum
@ HydrologySheetConductivityEnum
@ HydrologySheetThicknessEnum
void configure(DataSet *dataset)
IssmDouble alpha(IssmDouble x, IssmDouble y, IssmDouble z, int testid)
#define MARSHALLING(FIELD)
void GetVerticesCoordinates(IssmDouble *xyz, Vertex **vertices, int numvertices, bool spherical)
IssmDouble ODE1(IssmDouble alpha, IssmDouble beta, IssmDouble Si, IssmDouble dt, int method)
#define _error_(StreamArgs)
@ HydrologyChannelConductivityEnum
virtual int begin(void)=0
@ HydrologyChannelSheetWidthEnum
void GetSegmentNodalFunctionsDerivatives(IssmDouble *dbasis, IssmDouble *xyz_list_tria, Gauss *gauss, int index1, int index2, int finiteelement)
virtual void GaussPoint(int ig)=0
void GaussEdgeCenter(int index1, int index2)
void FindParam(bool *pinteger, int enum_type)
void AddToGlobal(Vector< IssmDouble > *pf)
void AddToGlobal(Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs)
Declaration of DataSet class.
int GetVertexIndex(Vertex *vertex)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)