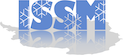 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
9 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
25 _assert_(vertex_sid>=0 && vertex_sid<iomodel->numberofvertices);
29 this->
sid = vertex_sid;
32 this->
clone = vertex_clone;
41 _assert_(iomodel->
Data(
"md.mesh.x") && iomodel->
Data(
"md.mesh.y") && iomodel->
Data(
"md.mesh.z"));
42 this->
x = iomodel->
Data(
"md.mesh.x")[vertex_sid];
43 this->
y = iomodel->
Data(
"md.mesh.y")[vertex_sid];
44 this->
z = iomodel->
Data(
"md.mesh.z")[vertex_sid];
45 if(iomodel->
Data(
"md.mesh.lat") && iomodel->
Data(
"md.mesh.long")){
46 this->
latitute = iomodel->
Data(
"md.mesh.lat")[vertex_sid];
52 _assert_(iomodel->
Data(
"md.geometry.base") && iomodel->
Data(
"md.geometry.thickness"));
53 this->
sigma = (iomodel->
Data(
"md.mesh.z")[vertex_sid]-iomodel->
Data(
"md.geometry.base")[vertex_sid])/(iomodel->
Data(
"md.geometry.thickness")[vertex_sid]);
56 _assert_(iomodel->
Data(
"md.mesh.lat") && iomodel->
Data(
"md.mesh.long") && iomodel->
Data(
"md.mesh.r"));
57 this->
latitute = iomodel->
Data(
"md.mesh.lat")[vertex_sid];
59 this->
R = iomodel->
Data(
"md.mesh.r")[vertex_sid];
65 _assert_(iomodel->
Data(
"md.geometry.base") && iomodel->
Data(
"md.geometry.thickness"));
66 this->
sigma = (iomodel->
Data(
"md.mesh.y")[vertex_sid]-iomodel->
Data(
"md.geometry.base")[vertex_sid])/(iomodel->
Data(
"md.geometry.thickness")[vertex_sid]);
116 void Vertex::Marshall(
char** pmarshalled_data,
int* pmarshalled_data_size,
int marshall_direction){
186 newy = bed[this->
pid]+
sigma*(surface[this->
pid] - bed[this->
pid]);
187 vely = (newy-oldy)/dt;
190 _assert_(!xIsNan<IssmDouble>(vely));
194 newz = bed[this->
pid]+
sigma*(surface[this->
pid] - bed[this->
pid]);
195 velz = (newz-oldz)/dt;
198 _assert_(!xIsNan<IssmDouble>(velz));
207 if(this->
clone==
true)
return;
231 for(
int i=0;i<numvertices;i++) {
232 xyz[i*3+0]=vertices[i]->
GetX();
233 xyz[i*3+1]=vertices[i]->
GetY();
234 xyz[i*3+2]=vertices[i]->
GetZ();
238 for(
int i=0;i<numvertices;i++) {
IssmDouble GetLongitude(void)
IssmDouble GetRadius(void)
int * numbernodetoelementconnectivity
#define _printf_(StreamArgs)
Declaration of Parameters class.
#define MARSHALLING_ENUM(EN)
@ TimesteppingTimeStepEnum
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
void UpdatePosition(Vector< IssmDouble > *vx, Vector< IssmDouble > *vy, Vector< IssmDouble > *vz, Parameters *parameters, IssmDouble *thickness, IssmDouble *bed)
#define MARSHALLING(FIELD)
void GetVerticesCoordinates(IssmDouble *xyz, Vertex **vertices, int numvertices, bool spherical)
IssmDouble * Data(const char *data_name)
#define _error_(StreamArgs)
IssmDouble GetLatitude(void)
void VertexCoordinates(Vector< IssmDouble > *vx, Vector< IssmDouble > *vy, Vector< IssmDouble > *vz, bool spherical=false)
void FindParam(bool *pinteger, int enum_type)
void SetValue(int dof, doubletype value, InsMode mode)