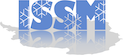 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
51 void Marshall(
char** pmarshalled_data,
int* pmarshalled_data_size,
int marshall_direction);
int GetNumberOfNodes(void)
void GetNodesSidList(int *sidlist)
Declaration of Vertices class.
void InputUpdateFromVectorDakota(IssmDouble *vector, int name, int type)
Declaration of Nodes class.
ElementVector * CreatePVectorHydrologyGlaDS(void)
ElementMatrix * CreateKMatrixHydrologyGlaDS(void)
Declaration of Parameters class.
Declaration of Elements class.
void PenaltyCreateJacobianMatrix(Matrix< IssmDouble > *Jff, IssmDouble kmax)
void GetNodesLidList(int *lidlist)
void InputUpdateFromConstant(bool constant, int name)
void PenaltyCreatePVector(Vector< IssmDouble > *pf, IssmDouble kmax)
void SetwiseNodeConnectivity(int *d_nz, int *o_nz, Node *node, bool *flags, int *flagsindices, int set1_enum, int set2_enum)
void CreateJacobianMatrix(Matrix< IssmDouble > *Jff)
Declaration of Materials class.
void Configure(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters)
abstract class for Load object This class is a place holder for the Icefront and the Penpair loads....
void CreateKMatrix(Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs)
void CreatePVector(Vector< IssmDouble > *pf)
void InputUpdateFromConstant(int constant, int name)
void InputUpdateFromIoModel(int index, IoModel *iomodel)
void SetChannelCrossSectionOld(void)
void InputUpdateFromConstant(IssmDouble constant, int name)
void SetCurrentConfiguration(Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters)
Declaration of Loads class.
void InputUpdateFromMatrixDakota(IssmDouble *matrix, int nrows, int ncols, int name, int type)
#define _error_(StreamArgs)
void WriteChannelCrossSection(IssmPDouble *values)
void PenaltyCreateKMatrix(Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *kfs, IssmDouble kmax)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
void UpdateChannelCrossSection(void)
void InputUpdateFromVector(IssmDouble *vector, int name, int type)