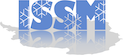 |
Ice Sheet System Model
4.18
Code documentation
|
#include <Numericalflux.h>
|
| Numericalflux () |
|
| Numericalflux (int numericalflux_id, int i, int index, IoModel *iomodel) |
|
| ~Numericalflux () |
|
Object * | copy () |
|
void | DeepEcho () |
|
void | Echo () |
|
int | Id () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | ObjectEnum () |
|
void | InputUpdateFromConstant (IssmDouble constant, int name) |
|
void | InputUpdateFromConstant (int constant, int name) |
|
void | InputUpdateFromConstant (bool constant, int name) |
|
void | InputUpdateFromIoModel (int index, IoModel *iomodel) |
|
void | InputUpdateFromMatrixDakota (IssmDouble *matrix, int nrows, int ncols, int name, int type) |
|
void | InputUpdateFromVector (IssmDouble *vector, int name, int type) |
|
void | InputUpdateFromVectorDakota (IssmDouble *vector, int name, int type) |
|
void | Configure (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | CreateJacobianMatrix (Matrix< IssmDouble > *Jff) |
|
void | CreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs) |
|
void | CreatePVector (Vector< IssmDouble > *pf) |
|
void | GetNodesLidList (int *lidlist) |
|
void | GetNodesSidList (int *sidlist) |
|
int | GetNumberOfNodes (void) |
|
int | GetNumberOfNodesOneSide (void) |
|
bool | IsPenalty (void) |
|
void | PenaltyCreateJacobianMatrix (Matrix< IssmDouble > *Jff, IssmDouble kmax) |
|
void | PenaltyCreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *kfs, IssmDouble kmax) |
|
void | PenaltyCreatePVector (Vector< IssmDouble > *pf, IssmDouble kmax) |
|
void | ResetHooks () |
|
void | SetCurrentConfiguration (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | SetwiseNodeConnectivity (int *d_nz, int *o_nz, Node *node, bool *flags, int *flagsindices, int set1_enum, int set2_enum) |
|
ElementMatrix * | CreateKMatrixAdjointBalancethickness (void) |
|
ElementMatrix * | CreateKMatrixAdjointBalancethicknessBoundary (void) |
|
ElementMatrix * | CreateKMatrixAdjointBalancethicknessInternal (void) |
|
ElementMatrix * | CreateKMatrixBalancethickness (void) |
|
ElementMatrix * | CreateKMatrixBalancethicknessBoundary (void) |
|
ElementMatrix * | CreateKMatrixBalancethicknessInternal (void) |
|
ElementMatrix * | CreateKMatrixMasstransport (void) |
|
ElementMatrix * | CreateKMatrixMasstransportBoundary (void) |
|
ElementMatrix * | CreateKMatrixMasstransportInternal (void) |
|
ElementVector * | CreatePVectorAdjointBalancethickness (void) |
|
ElementVector * | CreatePVectorBalancethickness (void) |
|
ElementVector * | CreatePVectorBalancethicknessBoundary (void) |
|
ElementVector * | CreatePVectorBalancethicknessInternal (void) |
|
ElementVector * | CreatePVectorMasstransport (void) |
|
ElementVector * | CreatePVectorMasstransportBoundary (void) |
|
ElementVector * | CreatePVectorMasstransportInternal (void) |
|
void | GetNormal (IssmDouble *normal, IssmDouble xyz_list[4][3]) |
|
virtual | ~Load () |
|
virtual | ~Object () |
|
Definition at line 18 of file Numericalflux.h.
◆ Numericalflux() [1/2]
Numericalflux::Numericalflux |
( |
| ) |
|
◆ Numericalflux() [2/2]
Numericalflux::Numericalflux |
( |
int |
numericalflux_id, |
|
|
int |
i, |
|
|
int |
index, |
|
|
IoModel * |
iomodel |
|
) |
| |
Definition at line 30 of file Numericalflux.cpp.
33 int pos1,pos2,pos3,pos4;
37 int numericalflux_elem_ids[2];
38 int numericalflux_vertex_ids[2];
39 int numericalflux_node_ids[4];
40 int numericalflux_type;
41 int numericalflux_degree;
44 int i1 = iomodel->
faces[4*index+0];
45 int i2 = iomodel->
faces[4*index+1];
46 int e1 = iomodel->
faces[4*index+2];
47 int e2 = iomodel->
faces[4*index+3];
53 numericalflux_elem_ids[0]=e1;
58 numericalflux_elem_ids[0]=e1;
59 numericalflux_elem_ids[1]=e2;
67 numericalflux_vertex_ids[0]=i1;
68 numericalflux_vertex_ids[1]=i2;
73 pos1=pos2=pos3=pos4=
UNDEF;
75 if(iomodel->
elements[3*(e1-1)+j]==i1) pos1=j+1;
76 if(iomodel->
elements[3*(e1-1)+j]==i2) pos2=j+1;
77 if(iomodel->
elements[3*(e2-1)+j]==i1) pos3=j+1;
78 if(iomodel->
elements[3*(e2-1)+j]==i2) pos4=j+1;
84 numericalflux_node_ids[0]=3*(e1-1)+pos1;
85 numericalflux_node_ids[1]=3*(e1-1)+pos2;
86 numericalflux_node_ids[2]=3*(e2-1)+pos3;
87 numericalflux_node_ids[3]=3*(e2-1)+pos4;
93 if(iomodel->
elements[3*(e1-1)+j]==i1) pos1=j+1;
94 if(iomodel->
elements[3*(e1-1)+j]==i2) pos2=j+1;
100 numericalflux_node_ids[0]=3*(e1-1)+pos1;
101 numericalflux_node_ids[1]=3*(e1-1)+pos2;
108 for(
int i=0;i<numnodes;i++) numericalflux_node_ids[i] = numericalflux_elem_ids[i];
109 numericalflux_node_ids[1] = numericalflux_elem_ids[1];
114 for(
int i=0;i<numnodes;i++) numericalflux_node_ids[i] = numericalflux_node_ids[i];
122 this->
id = numericalflux_id;
126 this->
hnodes =
new Hook(numericalflux_node_ids,numnodes);
◆ ~Numericalflux()
Numericalflux::~Numericalflux |
( |
| ) |
|
◆ copy()
Object * Numericalflux::copy |
( |
void |
| ) |
|
|
virtual |
◆ DeepEcho()
void Numericalflux::DeepEcho |
( |
void |
| ) |
|
|
virtual |
◆ Echo()
void Numericalflux::Echo |
( |
void |
| ) |
|
|
virtual |
◆ Id()
int Numericalflux::Id |
( |
void |
| ) |
|
|
virtual |
◆ Marshall()
void Numericalflux::Marshall |
( |
char ** |
pmarshalled_data, |
|
|
int * |
pmarshalled_data_size, |
|
|
int |
marshall_direction |
|
) |
| |
|
virtual |
◆ ObjectEnum()
int Numericalflux::ObjectEnum |
( |
void |
| ) |
|
|
virtual |
◆ InputUpdateFromConstant() [1/3]
void Numericalflux::InputUpdateFromConstant |
( |
IssmDouble |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromConstant() [2/3]
void Numericalflux::InputUpdateFromConstant |
( |
int |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromConstant() [3/3]
void Numericalflux::InputUpdateFromConstant |
( |
bool |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromIoModel()
void Numericalflux::InputUpdateFromIoModel |
( |
int |
index, |
|
|
IoModel * |
iomodel |
|
) |
| |
|
inline |
◆ InputUpdateFromMatrixDakota()
void Numericalflux::InputUpdateFromMatrixDakota |
( |
IssmDouble * |
matrix, |
|
|
int |
nrows, |
|
|
int |
ncols, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ InputUpdateFromVector()
void Numericalflux::InputUpdateFromVector |
( |
IssmDouble * |
vector, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ InputUpdateFromVectorDakota()
void Numericalflux::InputUpdateFromVectorDakota |
( |
IssmDouble * |
vector, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ Configure()
◆ CreateJacobianMatrix()
◆ CreateKMatrix()
◆ CreatePVector()
◆ GetNodesLidList()
void Numericalflux::GetNodesLidList |
( |
int * |
lidlist | ) |
|
|
virtual |
◆ GetNodesSidList()
void Numericalflux::GetNodesSidList |
( |
int * |
sidlist | ) |
|
|
virtual |
◆ GetNumberOfNodes()
int Numericalflux::GetNumberOfNodes |
( |
void |
| ) |
|
|
virtual |
◆ GetNumberOfNodesOneSide()
int Numericalflux::GetNumberOfNodesOneSide |
( |
void |
| ) |
|
◆ IsPenalty()
bool Numericalflux::IsPenalty |
( |
void |
| ) |
|
|
virtual |
◆ PenaltyCreateJacobianMatrix()
◆ PenaltyCreateKMatrix()
◆ PenaltyCreatePVector()
◆ ResetHooks()
void Numericalflux::ResetHooks |
( |
| ) |
|
|
virtual |
◆ SetCurrentConfiguration()
◆ SetwiseNodeConnectivity()
void Numericalflux::SetwiseNodeConnectivity |
( |
int * |
d_nz, |
|
|
int * |
o_nz, |
|
|
Node * |
node, |
|
|
bool * |
flags, |
|
|
int * |
flagsindices, |
|
|
int |
set1_enum, |
|
|
int |
set2_enum |
|
) |
| |
|
virtual |
Implements Load.
Definition at line 411 of file Numericalflux.cpp.
420 if(!flags[this->
nodes[i]->Lid()]){
426 while(flagsindices[counter]>=0) counter++;
427 flagsindices[counter]=this->nodes[i]->Lid();
433 if(this->nodes[i]->IsClone())
441 if(this->nodes[i]->IsClone())
449 if(this->nodes[i]->IsClone())
455 default:
_error_(
"not supported");
◆ CreateKMatrixAdjointBalancethickness()
ElementMatrix * Numericalflux::CreateKMatrixAdjointBalancethickness |
( |
void |
| ) |
|
◆ CreateKMatrixAdjointBalancethicknessBoundary()
ElementMatrix * Numericalflux::CreateKMatrixAdjointBalancethicknessBoundary |
( |
void |
| ) |
|
◆ CreateKMatrixAdjointBalancethicknessInternal()
ElementMatrix * Numericalflux::CreateKMatrixAdjointBalancethicknessInternal |
( |
void |
| ) |
|
◆ CreateKMatrixBalancethickness()
ElementMatrix * Numericalflux::CreateKMatrixBalancethickness |
( |
void |
| ) |
|
◆ CreateKMatrixBalancethicknessBoundary()
ElementMatrix * Numericalflux::CreateKMatrixBalancethicknessBoundary |
( |
void |
| ) |
|
Definition at line 505 of file Numericalflux.cpp.
532 IssmDouble UdotN=mean_vx*normal[0]+mean_vy*normal[1];
540 IssmDouble *basis = xNew<IssmDouble>(numnodes);
544 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
552 UdotN=vx*normal[0]+vy*normal[1];
554 DL=gauss->
weight*Jdet*UdotN;
556 for(
int i=0;i<numnodes;i++){
557 for(
int j=0;j<numnodes;j++){
558 Ke->
values[i*numnodes+j]+=DL*basis[i]*basis[j];
564 xDelete<IssmDouble>(basis);
◆ CreateKMatrixBalancethicknessInternal()
ElementMatrix * Numericalflux::CreateKMatrixBalancethicknessInternal |
( |
void |
| ) |
|
Definition at line 569 of file Numericalflux.cpp.
583 int numnodes_minus = numnodes_plus;
584 _assert_(numnodes==numnodes_plus+numnodes_minus);
588 IssmDouble *basis_plus = xNew<IssmDouble>(numnodes_plus);
589 IssmDouble *basis_minus = xNew<IssmDouble>(numnodes_minus);
601 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
610 UdotN=vx*normal[0]+vy*normal[1];
612 A1=gauss->
weight*Jdet*UdotN/2;
613 A2=gauss->
weight*Jdet*fabs(UdotN)/2;
628 for(
int i=0;i<numnodes_plus;i++){
629 for(
int j=0;j<numnodes_plus;j++){
630 Ke->
values[i*numnodes+j] += A1*(basis_plus[j]*basis_plus[i]);
631 Ke->
values[i*numnodes+j] += A2*(basis_plus[j]*basis_plus[i]);
635 for(
int i=0;i<numnodes_plus;i++){
636 for(
int j=0;j<numnodes_minus;j++){
637 Ke->
values[i*numnodes+numnodes_plus+j] += A1*(basis_minus[j]*basis_plus[i]);
638 Ke->
values[i*numnodes+numnodes_plus+j] += -A2*(basis_minus[j]*basis_plus[i]);
642 for(
int i=0;i<numnodes_minus;i++){
643 for(
int j=0;j<numnodes_plus;j++){
644 Ke->
values[(numnodes_plus+i)*numnodes+j] += -A1*(basis_plus[j]*basis_minus[i]);
645 Ke->
values[(numnodes_plus+i)*numnodes+j] += -A2*(basis_plus[j]*basis_minus[i]);
649 for(
int i=0;i<numnodes_minus;i++){
650 for(
int j=0;j<numnodes_minus;j++){
651 Ke->
values[(numnodes_plus+i)*numnodes+numnodes_plus+j] += -A1*(basis_minus[j]*basis_minus[i]);
652 Ke->
values[(numnodes_plus+i)*numnodes+numnodes_plus+j] += A2*(basis_minus[j]*basis_minus[i]);
658 xDelete<IssmDouble>(basis_plus);
659 xDelete<IssmDouble>(basis_minus);
◆ CreateKMatrixMasstransport()
ElementMatrix * Numericalflux::CreateKMatrixMasstransport |
( |
void |
| ) |
|
◆ CreateKMatrixMasstransportBoundary()
ElementMatrix * Numericalflux::CreateKMatrixMasstransportBoundary |
( |
void |
| ) |
|
Definition at line 676 of file Numericalflux.cpp.
704 IssmDouble UdotN=mean_vx*normal[0]+mean_vy*normal[1];
712 IssmDouble *basis = xNew<IssmDouble>(numnodes);
716 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
724 UdotN=vx*normal[0]+vy*normal[1];
726 DL=gauss->
weight*Jdet*dt*UdotN;
728 for(
int i=0;i<numnodes;i++){
729 for(
int j=0;j<numnodes;j++){
730 Ke->
values[i*numnodes+j]+=DL*basis[i]*basis[j];
736 xDelete<IssmDouble>(basis);
◆ CreateKMatrixMasstransportInternal()
ElementMatrix * Numericalflux::CreateKMatrixMasstransportInternal |
( |
void |
| ) |
|
Definition at line 741 of file Numericalflux.cpp.
755 int numnodes_minus = numnodes_plus;
756 _assert_(numnodes==numnodes_plus+numnodes_minus);
760 IssmDouble *basis_plus = xNew<IssmDouble>(numnodes_plus);
761 IssmDouble *basis_minus = xNew<IssmDouble>(numnodes_minus);
774 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
783 UdotN=vx*normal[0]+vy*normal[1];
785 A1=gauss->
weight*Jdet*dt*UdotN/2;
786 A2=gauss->
weight*Jdet*dt*fabs(UdotN)/2;
801 for(
int i=0;i<numnodes_plus;i++){
802 for(
int j=0;j<numnodes_plus;j++){
803 Ke->
values[i*numnodes+j] += A1*(basis_plus[j]*basis_plus[i]);
804 Ke->
values[i*numnodes+j] += A2*(basis_plus[j]*basis_plus[i]);
808 for(
int i=0;i<numnodes_plus;i++){
809 for(
int j=0;j<numnodes_minus;j++){
810 Ke->
values[i*numnodes+numnodes_plus+j] += A1*(basis_minus[j]*basis_plus[i]);
811 Ke->
values[i*numnodes+numnodes_plus+j] += -A2*(basis_minus[j]*basis_plus[i]);
815 for(
int i=0;i<numnodes_minus;i++){
816 for(
int j=0;j<numnodes_plus;j++){
817 Ke->
values[(numnodes_plus+i)*numnodes+j] += -A1*(basis_plus[j]*basis_minus[i]);
818 Ke->
values[(numnodes_plus+i)*numnodes+j] += -A2*(basis_plus[j]*basis_minus[i]);
822 for(
int i=0;i<numnodes_minus;i++){
823 for(
int j=0;j<numnodes_minus;j++){
824 Ke->
values[(numnodes_plus+i)*numnodes+numnodes_plus+j] += -A1*(basis_minus[j]*basis_minus[i]);
825 Ke->
values[(numnodes_plus+i)*numnodes+numnodes_plus+j] += A2*(basis_minus[j]*basis_minus[i]);
831 xDelete<IssmDouble>(basis_plus);
832 xDelete<IssmDouble>(basis_minus);
◆ CreatePVectorAdjointBalancethickness()
ElementVector * Numericalflux::CreatePVectorAdjointBalancethickness |
( |
void |
| ) |
|
◆ CreatePVectorBalancethickness()
ElementVector * Numericalflux::CreatePVectorBalancethickness |
( |
void |
| ) |
|
◆ CreatePVectorBalancethicknessBoundary()
ElementVector * Numericalflux::CreatePVectorBalancethicknessBoundary |
( |
void |
| ) |
|
Definition at line 855 of file Numericalflux.cpp.
862 IssmDouble DL,Jdet,vx,vy,mean_vx,mean_vy,thickness;
881 IssmDouble UdotN=mean_vx*normal[0]+mean_vy*normal[1];
889 IssmDouble *basis = xNew<IssmDouble>(numnodes);
893 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
903 UdotN=vx*normal[0]+vy*normal[1];
905 DL= - gauss->
weight*Jdet*UdotN*thickness;
907 for(
int i=0;i<numnodes;i++) pe->
values[i] += DL*basis[i];
911 xDelete<IssmDouble>(basis);
◆ CreatePVectorBalancethicknessInternal()
ElementVector * Numericalflux::CreatePVectorBalancethicknessInternal |
( |
void |
| ) |
|
◆ CreatePVectorMasstransport()
ElementVector * Numericalflux::CreatePVectorMasstransport |
( |
void |
| ) |
|
◆ CreatePVectorMasstransportBoundary()
ElementVector * Numericalflux::CreatePVectorMasstransportBoundary |
( |
void |
| ) |
|
Definition at line 935 of file Numericalflux.cpp.
942 IssmDouble DL,Jdet,vx,vy,mean_vx,mean_vy,thickness;
962 IssmDouble UdotN=mean_vx*normal[0]+mean_vy*normal[1];
970 IssmDouble *basis = xNew<IssmDouble>(numnodes);
974 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
983 if(xIsNan<IssmDouble>(thickness))
_error_(
"Cannot weakly apply constraint because NaN was provided");
985 UdotN=vx*normal[0]+vy*normal[1];
987 DL= - gauss->
weight*Jdet*dt*UdotN*thickness;
989 for(
int i=0;i<numnodes;i++) pe->
values[i] += DL*basis[i];
993 xDelete<IssmDouble>(basis);
◆ CreatePVectorMasstransportInternal()
ElementVector * Numericalflux::CreatePVectorMasstransportInternal |
( |
void |
| ) |
|
◆ GetNormal()
Definition at line 1005 of file Numericalflux.cpp.
1010 vector[0]=xyz_list[1][0] - xyz_list[0][0];
1011 vector[1]=xyz_list[1][1] - xyz_list[0][1];
1013 IssmDouble norm=sqrt(pow(vector[0],2.0)+pow(vector[1],2.0));
1015 normal[0]= + vector[1]/norm;
1016 normal[1]= - vector[0]/norm;
◆ id
◆ flux_type
int Numericalflux::flux_type |
◆ flux_degree
int Numericalflux::flux_degree |
◆ helement
Hook* Numericalflux::helement |
◆ hnodes
Hook* Numericalflux::hnodes |
◆ hvertices
Hook* Numericalflux::hvertices |
◆ element
◆ vertices
Vertex** Numericalflux::vertices |
◆ nodes
Node** Numericalflux::nodes |
◆ parameters
The documentation for this class was generated from the following files:
@ BalancethicknessAnalysisEnum
ElementMatrix * CreateKMatrixBalancethicknessInternal(void)
@ MasstransportAnalysisEnum
void GetSegmentJacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, Gauss *gauss)
ElementVector * CreatePVectorMasstransportBoundary(void)
ElementVector * CreatePVectorAdjointBalancethickness(void)
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
@ TimesteppingTimeStepEnum
void GetNormal(IssmDouble *normal, IssmDouble xyz_list[4][3])
int GetNumberOfNodes(void)
ElementVector * CreatePVectorMasstransportInternal(void)
ElementMatrix * CreateKMatrixMasstransportInternal(void)
void GetSegmentNodalFunctions(IssmDouble *basis, Gauss *gauss, int index1, int index2, int finiteelement)
@ AdjointBalancethicknessAnalysisEnum
@ MasstransportSpcthicknessEnum
ElementMatrix * CreateKMatrixAdjointBalancethicknessInternal(void)
ElementMatrix * CreateKMatrixAdjointBalancethicknessBoundary(void)
ElementMatrix * CreateKMatrixBalancethickness(void)
const char * EnumToStringx(int enum_in)
ElementMatrix * CreateKMatrixMasstransportBoundary(void)
void configure(DataSet *dataset)
ElementMatrix * CreateKMatrixAdjointBalancethickness(void)
ElementMatrix * CreateKMatrixMasstransport(void)
#define MARSHALLING(FIELD)
int GetNumberOfNodesOneSide(void)
void GetVerticesCoordinates(IssmDouble *xyz, Vertex **vertices, int numvertices, bool spherical)
ElementVector * CreatePVectorBalancethickness(void)
ElementVector * CreatePVectorBalancethicknessBoundary(void)
ElementVector * CreatePVectorMasstransport(void)
#define _error_(StreamArgs)
ElementVector * CreatePVectorBalancethicknessInternal(void)
void GaussEdgeCenter(int index1, int index2)
void FindParam(bool *pinteger, int enum_type)
void AddToGlobal(Vector< IssmDouble > *pf)
void AddToGlobal(Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs)
Declaration of DataSet class.
ElementMatrix * CreateKMatrixBalancethicknessBoundary(void)
Input2 * GetInput2(int enumtype)
int GetVertexIndex(Vertex *vertex)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)