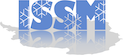 |
Ice Sheet System Model
4.18
Code documentation
|
#include <Neumannflux.h>
|
| Neumannflux () |
|
| Neumannflux (int numericalflux_id, int i, IoModel *iomodel, int *segments) |
|
| ~Neumannflux () |
|
Object * | copy () |
|
void | DeepEcho () |
|
void | Echo () |
|
int | Id () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | ObjectEnum () |
|
void | InputUpdateFromConstant (IssmDouble constant, int name) |
|
void | InputUpdateFromConstant (int constant, int name) |
|
void | InputUpdateFromConstant (bool constant, int name) |
|
void | InputUpdateFromIoModel (int index, IoModel *iomodel) |
|
void | InputUpdateFromMatrixDakota (IssmDouble *matrix, int nrows, int ncols, int name, int type) |
|
void | InputUpdateFromVector (IssmDouble *vector, int name, int type) |
|
void | InputUpdateFromVectorDakota (IssmDouble *vector, int name, int type) |
|
void | Configure (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | CreateJacobianMatrix (Matrix< IssmDouble > *Jff) |
|
void | CreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs) |
|
void | CreatePVector (Vector< IssmDouble > *pf) |
|
void | GetNodesLidList (int *lidlist) |
|
void | GetNodesSidList (int *sidlist) |
|
int | GetNumberOfNodes (void) |
|
bool | IsPenalty (void) |
|
void | PenaltyCreateJacobianMatrix (Matrix< IssmDouble > *Jff, IssmDouble kmax) |
|
void | PenaltyCreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *kfs, IssmDouble kmax) |
|
void | PenaltyCreatePVector (Vector< IssmDouble > *pf, IssmDouble kmax) |
|
void | ResetHooks () |
|
void | SetCurrentConfiguration (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | SetwiseNodeConnectivity (int *d_nz, int *o_nz, Node *node, bool *flags, int *flagsindices, int set1_enum, int set2_enum) |
|
ElementVector * | CreatePVectorHydrologyShakti (void) |
|
ElementVector * | CreatePVectorHydrologyGlaDS (void) |
|
virtual | ~Load () |
|
virtual | ~Object () |
|
Definition at line 18 of file Neumannflux.h.
◆ Neumannflux() [1/2]
Neumannflux::Neumannflux |
( |
| ) |
|
◆ Neumannflux() [2/2]
Neumannflux::Neumannflux |
( |
int |
numericalflux_id, |
|
|
int |
i, |
|
|
IoModel * |
iomodel, |
|
|
int * |
segments |
|
) |
| |
Definition at line 31 of file Neumannflux.cpp.
37 int neumannflux_elem_id;
38 int neumannflux_vertex_ids[2];
39 int neumannflux_node_ids[2];
42 neumannflux_vertex_ids[0]=segments[3*i+0];
43 neumannflux_vertex_ids[1]=segments[3*i+1];
46 neumannflux_node_ids[0]=neumannflux_vertex_ids[0];
47 neumannflux_node_ids[1]=neumannflux_vertex_ids[1];
50 neumannflux_elem_id = segments[3*i+2];
53 this->
id=neumannflux_id;
56 this->
hnodes =
new Hook(&neumannflux_node_ids[0],2);
◆ ~Neumannflux()
Neumannflux::~Neumannflux |
( |
| ) |
|
◆ copy()
Object * Neumannflux::copy |
( |
void |
| ) |
|
|
virtual |
◆ DeepEcho()
void Neumannflux::DeepEcho |
( |
void |
| ) |
|
|
virtual |
◆ Echo()
void Neumannflux::Echo |
( |
void |
| ) |
|
|
virtual |
◆ Id()
int Neumannflux::Id |
( |
void |
| ) |
|
|
virtual |
◆ Marshall()
void Neumannflux::Marshall |
( |
char ** |
pmarshalled_data, |
|
|
int * |
pmarshalled_data_size, |
|
|
int |
marshall_direction |
|
) |
| |
|
virtual |
◆ ObjectEnum()
int Neumannflux::ObjectEnum |
( |
void |
| ) |
|
|
virtual |
◆ InputUpdateFromConstant() [1/3]
void Neumannflux::InputUpdateFromConstant |
( |
IssmDouble |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromConstant() [2/3]
void Neumannflux::InputUpdateFromConstant |
( |
int |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromConstant() [3/3]
void Neumannflux::InputUpdateFromConstant |
( |
bool |
constant, |
|
|
int |
name |
|
) |
| |
|
inline |
◆ InputUpdateFromIoModel()
void Neumannflux::InputUpdateFromIoModel |
( |
int |
index, |
|
|
IoModel * |
iomodel |
|
) |
| |
|
inline |
◆ InputUpdateFromMatrixDakota()
void Neumannflux::InputUpdateFromMatrixDakota |
( |
IssmDouble * |
matrix, |
|
|
int |
nrows, |
|
|
int |
ncols, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ InputUpdateFromVector()
void Neumannflux::InputUpdateFromVector |
( |
IssmDouble * |
vector, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ InputUpdateFromVectorDakota()
void Neumannflux::InputUpdateFromVectorDakota |
( |
IssmDouble * |
vector, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
|
inline |
◆ Configure()
◆ CreateJacobianMatrix()
◆ CreateKMatrix()
◆ CreatePVector()
◆ GetNodesLidList()
void Neumannflux::GetNodesLidList |
( |
int * |
lidlist | ) |
|
|
virtual |
◆ GetNodesSidList()
void Neumannflux::GetNodesSidList |
( |
int * |
sidlist | ) |
|
|
virtual |
◆ GetNumberOfNodes()
int Neumannflux::GetNumberOfNodes |
( |
void |
| ) |
|
|
virtual |
◆ IsPenalty()
bool Neumannflux::IsPenalty |
( |
void |
| ) |
|
|
virtual |
◆ PenaltyCreateJacobianMatrix()
◆ PenaltyCreateKMatrix()
◆ PenaltyCreatePVector()
◆ ResetHooks()
void Neumannflux::ResetHooks |
( |
| ) |
|
|
virtual |
◆ SetCurrentConfiguration()
◆ SetwiseNodeConnectivity()
void Neumannflux::SetwiseNodeConnectivity |
( |
int * |
d_nz, |
|
|
int * |
o_nz, |
|
|
Node * |
node, |
|
|
bool * |
flags, |
|
|
int * |
flagsindices, |
|
|
int |
set1_enum, |
|
|
int |
set2_enum |
|
) |
| |
|
virtual |
Implements Load.
Definition at line 287 of file Neumannflux.cpp.
296 if(!flags[this->
nodes[i]->Lid()]){
302 while(flagsindices[counter]>=0) counter++;
303 flagsindices[counter]=this->nodes[i]->Lid();
309 if(this->nodes[i]->IsClone())
317 if(this->nodes[i]->IsClone())
325 if(this->nodes[i]->IsClone())
331 default:
_error_(
"not supported");
◆ CreatePVectorHydrologyShakti()
ElementVector * Neumannflux::CreatePVectorHydrologyShakti |
( |
void |
| ) |
|
Definition at line 343 of file Neumannflux.cpp.
371 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
379 for(
int i=0;i<numdof;i++) pe->
values[i] += gauss->
weight*Jdet*flux*basis[i];
◆ CreatePVectorHydrologyGlaDS()
ElementVector * Neumannflux::CreatePVectorHydrologyGlaDS |
( |
void |
| ) |
|
Definition at line 387 of file Neumannflux.cpp.
415 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
423 for(
int i=0;i<numdof;i++) pe->
values[i] += gauss->
weight*Jdet*flux*basis[i];
◆ id
◆ helement
Hook* Neumannflux::helement |
◆ hnodes
Hook* Neumannflux::hnodes |
◆ hvertices
Hook* Neumannflux::hvertices |
◆ element
◆ vertices
Vertex** Neumannflux::vertices |
◆ nodes
Node** Neumannflux::nodes |
◆ parameters
The documentation for this class was generated from the following files:
void GetSegmentJacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, Gauss *gauss)
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
@ HydrologyShaktiAnalysisEnum
void GetSegmentNodalFunctions(IssmDouble *basis, Gauss *gauss, int index1, int index2, int finiteelement)
@ HydrologyNeumannfluxEnum
int GetNumberOfNodes(void)
@ HydrologyGlaDSAnalysisEnum
ElementVector * CreatePVectorHydrologyGlaDS(void)
const char * EnumToStringx(int enum_in)
void configure(DataSet *dataset)
#define MARSHALLING(FIELD)
void GetVerticesCoordinates(IssmDouble *xyz, Vertex **vertices, int numvertices, bool spherical)
ElementVector * CreatePVectorHydrologyShakti(void)
#define _error_(StreamArgs)
#define NUMNODES_BOUNDARY
void FindParam(bool *pinteger, int enum_type)
void AddToGlobal(Vector< IssmDouble > *pf)
void AddToGlobal(Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs)
Declaration of DataSet class.
Input2 * GetInput2(int enumtype)
int GetVertexIndex(Vertex *vertex)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)