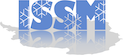 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
13 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
16 #include "../../shared/Enum/Enum.h"
17 #include "../../shared/Exceptions/exceptions.h"
18 #include "../../shared/MemOps/MemOps.h"
33 template <
class doubletype>
56 _error_(
"Mpi vector requires compilation of MPI!");
60 _error_(
"vector type not supported yet!");
75 _error_(
"Mpi vector requires compilation of MPI!");
79 _error_(
"vector type not supported yet!");
94 _error_(
"Mpi vector requires compilation of MPI!");
98 _error_(
"vector type not supported yet!");
113 _error_(
"Mpi vector requires compilation of MPI!");
117 _error_(
"vector type not supported yet!");
136 vector->SetValues(ssize,list,values,mode);
140 vector->SetValue(dof,value,mode);
144 vector->GetValue(pvalue,dof);
156 vector->GetLocalVector(pvector,pindices);
169 void Set(doubletype value){
182 return vector->ToMPISerial();
186 return vector->ToMPISerial0();
198 return vector->Norm(mode);
201 void Scale(doubletype scale_factor){
202 vector->Scale(scale_factor);
215 #endif //#ifndef _ISSMVEC_H_
void AYPX(IssmVec *X, doubletype a)
void SetValue(int dof, doubletype value, InsMode mode)
IssmVec(doubletype *buffer, int M)
void Shift(doubletype shift)
virtual void Echo(void)=0
void SetValues(int ssize, int *list, doubletype *values, InsMode mode)
doubletype Norm(NormMode mode)
void Set(doubletype value)
void Scale(doubletype scale_factor)
void GetLocalSize(int *pM)
IssmAbsVec< doubletype > * vector
int IssmVecTypeFromToolkitOptions(void)
doubletype Dot(IssmVec *input)
void PointwiseDivide(IssmVec *x, IssmVec *y)
void AXPY(IssmVec *X, doubletype a)
#define _error_(StreamArgs)
virtual IssmAbsVec< doubletype > * Duplicate(void)=0
IssmVec(int M, bool fromlocalsize)
virtual void Copy(IssmAbsVec *to)=0
doubletype * ToMPISerial0(void)
doubletype * ToMPISerial(void)
void GetValue(doubletype *pvalue, int dof)
void GetLocalVector(doubletype **pvector, int **pindices)
IssmVec< doubletype > * Duplicate(void)