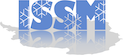 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
15 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
18 #include "../petscincludes.h"
33 PetscVec(
int M,
bool fromlocalsize=
false);
65 #endif //#ifndef _PETSCVEC_H_
void AYPX(PetscVec *X, IssmDouble a)
void SetValues(int ssize, int *list, IssmDouble *values, InsMode mode)
void GetLocalSize(int *pM)
void PointwiseMult(PetscVec *x, PetscVec *y)
void GetValue(IssmDouble *pvalue, int dof)
IssmDouble Dot(PetscVec *vector)
void Scale(IssmDouble scale_factor)
void Shift(IssmDouble shift)
IssmDouble * ToMPISerial0(void)
PetscVec * Duplicate(void)
void GetLocalVector(IssmDouble **pvector, int **pindices)
IssmDouble Norm(NormMode norm_type)
void PointwiseDivide(PetscVec *x, PetscVec *y)
void AXPY(PetscVec *X, IssmDouble a)
void Pow(IssmDouble scale_factor)
IssmDouble * ToMPISerial(void)
void Set(IssmDouble value)
void SetValue(int dof, IssmDouble value, InsMode mode)