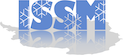 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
7 #include "../../shared/shared.h"
8 #include "../../toolkits/toolkits.h"
14 int found,sumfound,cpu_found,cpu;
24 for(
int i=0;i<elements->
Size();i++){
26 found=element->
NodalValue(&value,index,natureofdataenum);
35 if(!sumfound)
_error_(
"could not find element with vertex with id " << index <<
" to compute nodal value " <<
EnumToStringx(natureofdataenum));
Declaration of Vertices class.
Declaration of Nodes class.
int ISSM_MPI_Allreduce(void *sendbuf, void *recvbuf, int count, ISSM_MPI_Datatype datatype, ISSM_MPI_Op op, ISSM_MPI_Comm comm)
Declaration of Parameters class.
static ISSM_MPI_Comm GetComm(void)
Declaration of Elements class.
void NodalValuex(IssmDouble *pnodalvalue, int natureofdataenum, Elements *elements, Nodes *nodes, Vertices *vertices, Loads *loads, Materials *materials, Parameters *parameters)
header file for NodalValuex
virtual int NodalValue(IssmDouble *pvalue, int index, int natureofdataenum)=0
Declaration of Materials class.
const char * EnumToStringx(int enum_in)
int ISSM_MPI_Bcast(void *buffer, int count, ISSM_MPI_Datatype datatype, int root, ISSM_MPI_Comm comm)
Declaration of Loads class.
#define _error_(StreamArgs)
Object * GetObjectByOffset(int offset)
void FindParam(bool *pinteger, int enum_type)