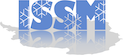 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
13 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
16 #include "../../shared/shared.h"
17 #include "../ToolkitOptions.h"
19 #include "../mumps/mumpsincludes.h"
28 template <
class doubletype>
class IssmVec;
34 template <
class doubletype>
53 _error_(
"MpiDense matrix requires compilation of MPI!");
60 _error_(
"MpiSparse matrix requires compilation of MPI!");
64 _error_(
"matrix type not supported yet!");
79 _error_(
"MpiDense matrix requires compilation of MPI!");
86 _error_(
"MpiSparse matrix requires compilation of MPI!");
90 _error_(
"matrix type not supported yet!");
94 IssmMat(
int M,
int N, doubletype sparsity){
105 _error_(
"MpiDense matrix requires compilation of MPI!");
112 _error_(
"MpiSparse matrix requires compilation of MPI!");
116 _error_(
"matrix type not supported yet!");
120 IssmMat(
int m,
int n,
int M,
int N,
int* d_nnz,
int* o_nnz){
131 _error_(
"MpiDense matrix requires compilation of MPI!");
138 _error_(
"MpiSparse matrix requires compilation of MPI!");
142 _error_(
"matrix type not supported yet!");
146 IssmMat(doubletype* serial_mat,
int M,
int N,doubletype sparsity){
157 _error_(
"MpiDense matrix requires compilation of MPI!");
164 _error_(
"MpiSparse matrix requires compilation of MPI!");
168 _error_(
"matrix type not supported yet!");
173 IssmMat(
int M,
int N,
int connectivity,
int numberofdofspernode){
184 _error_(
"MpiDense matrix requires compilation of MPI!");
191 _error_(
"MpiSparse matrix requires compilation of MPI!");
195 _error_(
"matrix type not supported yet!");
211 return matrix->Norm(mode);
218 matrix->GetLocalSize(pM,pN);
233 return matrix->ToSerial();
236 matrix->SetValues(m,idxm,n,idxn,values,mode);
244 #ifndef _HAVE_WRAPPERS_
259 #endif //#ifndef _ISSMMAT_H_
IssmMat(int m, int n, int M, int N, int *d_nnz, int *o_nnz)
IssmMat(doubletype *serial_mat, int M, int N, doubletype sparsity)
Declaration of Parameters class.
doubletype Norm(NormMode mode)
void SetValues(int m, int *idxm, int n, int *idxn, doubletype *values, InsMode mode)
virtual IssmAbsVec< IssmDouble > * Solve(IssmAbsVec< IssmDouble > *pf, Parameters *parameters)=0
IssmMat< doubletype > * Duplicate(void)
IssmMat(int M, int N, int connectivity, int numberofdofspernode)
IssmVec< doubletype > * Solve(IssmVec< doubletype > *pf, Parameters *parameters)
IssmAbsMat< doubletype > * matrix
IssmAbsVec< doubletype > * vector
doubletype * ToSerial(void)
virtual IssmAbsMat< doubletype > * Duplicate(void)=0
IssmMat(int M, int N, doubletype sparsity)
#define _error_(StreamArgs)
void MatMult(IssmVec< doubletype > *X, IssmVec< doubletype > *AX)
void Convert(MatrixType type)
void GetSize(int *pM, int *pN)
void GetLocalSize(int *pM, int *pN)