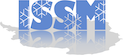 |
Ice Sheet System Model
4.18
Code documentation
|
#include <Pengrid.h>
|
| Pengrid () |
|
| Pengrid (int id, int index, IoModel *iomodel) |
|
| ~Pengrid () |
|
Object * | copy () |
|
void | DeepEcho () |
|
void | Echo () |
|
int | Id () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | ObjectEnum () |
|
void | Configure (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | CreateJacobianMatrix (Matrix< IssmDouble > *Jff) |
|
void | CreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs) |
|
void | CreatePVector (Vector< IssmDouble > *pf) |
|
void | GetNodesLidList (int *lidlist) |
|
void | GetNodesSidList (int *sidlist) |
|
int | GetNumberOfNodes (void) |
|
bool | IsPenalty (void) |
|
void | PenaltyCreateJacobianMatrix (Matrix< IssmDouble > *Jff, IssmDouble kmax) |
|
void | PenaltyCreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *kfs, IssmDouble kmax) |
|
void | PenaltyCreatePVector (Vector< IssmDouble > *pf, IssmDouble kmax) |
|
void | ResetHooks () |
|
void | SetCurrentConfiguration (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | SetwiseNodeConnectivity (int *d_nz, int *o_nz, Node *node, bool *flags, int *flagsindices, int set1_enum, int set2_enum) |
|
void | ConstraintActivate (int *punstable) |
|
void | ConstraintActivateHydrologyDCInefficient (int *punstable) |
|
void | ConstraintActivateThermal (int *punstable) |
|
ElementMatrix * | PenaltyCreateKMatrixHydrologyDCInefficient (IssmDouble kmax) |
|
ElementMatrix * | PenaltyCreateKMatrixMelting (IssmDouble kmax) |
|
ElementMatrix * | PenaltyCreateKMatrixThermal (IssmDouble kmax) |
|
ElementVector * | PenaltyCreatePVectorHydrologyDCInefficient (IssmDouble kmax) |
|
ElementVector * | PenaltyCreatePVectorMelting (IssmDouble kmax) |
|
ElementVector * | PenaltyCreatePVectorThermal (IssmDouble kmax) |
|
void | ResetConstraint (void) |
|
void | ResetZigzagCounter (void) |
|
virtual | ~Load () |
|
virtual | ~Object () |
|
Definition at line 21 of file Pengrid.h.
◆ Pengrid() [1/2]
◆ Pengrid() [2/2]
Pengrid::Pengrid |
( |
int |
id, |
|
|
int |
index, |
|
|
IoModel * |
iomodel |
|
) |
| |
Definition at line 35 of file Pengrid.cpp.
38 int pengrid_element_id;
42 _assert_(index>=0 && index<iomodel->numberofvertices);
49 pengrid_node_id=index+1;
◆ ~Pengrid()
◆ copy()
Object * Pengrid::copy |
( |
void |
| ) |
|
|
virtual |
◆ DeepEcho()
void Pengrid::DeepEcho |
( |
void |
| ) |
|
|
virtual |
◆ Echo()
void Pengrid::Echo |
( |
void |
| ) |
|
|
virtual |
◆ Id()
◆ Marshall()
void Pengrid::Marshall |
( |
char ** |
pmarshalled_data, |
|
|
int * |
pmarshalled_data_size, |
|
|
int |
marshall_direction |
|
) |
| |
|
virtual |
◆ ObjectEnum()
int Pengrid::ObjectEnum |
( |
void |
| ) |
|
|
virtual |
◆ Configure()
Implements Load.
Definition at line 153 of file Pengrid.cpp.
165 this->parameters=parametersin;
◆ CreateJacobianMatrix()
◆ CreateKMatrix()
◆ CreatePVector()
◆ GetNodesLidList()
void Pengrid::GetNodesLidList |
( |
int * |
lidlist | ) |
|
|
virtual |
◆ GetNodesSidList()
void Pengrid::GetNodesSidList |
( |
int * |
sidlist | ) |
|
|
virtual |
◆ GetNumberOfNodes()
int Pengrid::GetNumberOfNodes |
( |
void |
| ) |
|
|
virtual |
◆ IsPenalty()
bool Pengrid::IsPenalty |
( |
void |
| ) |
|
|
virtual |
◆ PenaltyCreateJacobianMatrix()
◆ PenaltyCreateKMatrix()
Implements Load.
Definition at line 206 of file Pengrid.cpp.
213 switch(analysis_type){
224 _error_(
"analysis " << analysis_type <<
" (" <<
EnumToStringx(analysis_type) <<
") not supported yet");
◆ PenaltyCreatePVector()
Implements Load.
Definition at line 234 of file Pengrid.cpp.
241 switch(analysis_type){
254 _error_(
"analysis " << analysis_type <<
" (" <<
EnumToStringx(analysis_type) <<
") not supported yet");
◆ ResetHooks()
void Pengrid::ResetHooks |
( |
| ) |
|
|
virtual |
◆ SetCurrentConfiguration()
◆ SetwiseNodeConnectivity()
void Pengrid::SetwiseNodeConnectivity |
( |
int * |
d_nz, |
|
|
int * |
o_nz, |
|
|
Node * |
node, |
|
|
bool * |
flags, |
|
|
int * |
flagsindices, |
|
|
int |
set1_enum, |
|
|
int |
set2_enum |
|
) |
| |
|
virtual |
Implements Load.
Definition at line 280 of file Pengrid.cpp.
286 if(!flags[this->node->
Lid()]){
289 flags[this->node->
Lid()]=
true;
292 while(flagsindices[counter]>=0) counter++;
293 flagsindices[counter]=this->node->
Lid();
321 default:
_error_(
"not supported");
◆ ConstraintActivate()
void Pengrid::ConstraintActivate |
( |
int * |
punstable | ) |
|
Definition at line 332 of file Pengrid.cpp.
339 switch(analysis_type){
◆ ConstraintActivateHydrologyDCInefficient()
void Pengrid::ConstraintActivateHydrologyDCInefficient |
( |
int * |
punstable | ) |
|
◆ ConstraintActivateThermal()
void Pengrid::ConstraintActivateThermal |
( |
int * |
punstable | ) |
|
Definition at line 417 of file Pengrid.cpp.
449 if (temperature>t_pmp){
◆ PenaltyCreateKMatrixHydrologyDCInefficient()
◆ PenaltyCreateKMatrixMelting()
Definition at line 496 of file Pengrid.cpp.
516 if (temperature<t_pmp){
517 Ke->
values[0]=kmax*pow(10.,penalty_factor);
◆ PenaltyCreateKMatrixThermal()
◆ PenaltyCreatePVectorHydrologyDCInefficient()
Definition at line 541 of file Pengrid.cpp.
548 if(!this->
active)
return NULL;
558 pe->
values[0]=kmax*pow(10.,penalty_factor)*h_max;
◆ PenaltyCreatePVectorMelting()
Definition at line 565 of file Pengrid.cpp.
594 if (temperature<t_pmp){
598 if (reCast<bool>(dt)) pe->
values[0]=melting_offset*pow(10.,penalty_factor)*(temperature-t_pmp)/dt;
599 else pe->
values[0]=melting_offset*pow(10.,penalty_factor)*(temperature-t_pmp);
◆ PenaltyCreatePVectorThermal()
Definition at line 606 of file Pengrid.cpp.
615 if(!this->
active)
return NULL;
625 pe->
values[0]=kmax*pow(10.,penalty_factor)*t_pmp;
◆ ResetConstraint()
void Pengrid::ResetConstraint |
( |
void |
| ) |
|
◆ ResetZigzagCounter()
void Pengrid::ResetZigzagCounter |
( |
void |
| ) |
|
◆ id
◆ hnode
◆ helement
◆ node
◆ element
◆ parameters
◆ active
◆ zigzag_counter
int Pengrid::zigzag_counter |
|
private |
The documentation for this class was generated from the following files:
ElementMatrix * PenaltyCreateKMatrixHydrologyDCInefficient(IssmDouble kmax)
@ ThermalPenaltyFactorEnum
@ StressbalanceAnalysisEnum
#define _printf_(StreamArgs)
@ MaterialsMeltingpointEnum
#define MARSHALLING_ENUM(EN)
@ TimesteppingTimeStepEnum
void ConstraintActivateThermal(int *punstable)
@ HydrologydcPenaltyFactorEnum
ElementMatrix * PenaltyCreateKMatrixThermal(IssmDouble kmax)
ElementVector * PenaltyCreatePVectorMelting(IssmDouble kmax)
ElementVector * PenaltyCreatePVectorThermal(IssmDouble kmax)
@ HydrologyDCInefficientAnalysisEnum
@ AdjointHorizAnalysisEnum
const char * EnumToStringx(int enum_in)
void configure(DataSet *dataset)
void ConstraintActivateHydrologyDCInefficient(int *punstable)
#define MARSHALLING(FIELD)
void GetHydrologyDCInefficientHmax(IssmDouble *ph_max, Element *element, Node *innode)
@ HydrologydcPenaltyLockEnum
IssmDouble TMeltingPoint(IssmDouble pressure)
int * singlenodetoelementconnectivity
@ SedimentHeadSubstepEnum
#define _error_(StreamArgs)
ElementVector * PenaltyCreatePVectorHydrologyDCInefficient(IssmDouble kmax)
void GetInputValue(bool *pvalue, int enum_type)
void FindParam(bool *pinteger, int enum_type)
void AddToGlobal(Vector< IssmDouble > *pf)
void GetInputValue(IssmDouble *pvalue, Vertex *vertex, int enumtype)
void AddToGlobal(Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs)
ElementMatrix * PenaltyCreateKMatrixMelting(IssmDouble kmax)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)