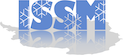 |
Ice Sheet System Model
4.18
Code documentation
|
#include <Moulin.h>
|
| Moulin () |
|
| Moulin (int index, int id, IoModel *iomodel) |
|
| ~Moulin () |
|
Object * | copy () |
|
void | DeepEcho () |
|
void | Echo () |
|
int | Id () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | ObjectEnum () |
|
void | InputUpdateFromConstant (IssmDouble constant, int name) |
|
void | InputUpdateFromConstant (int constant, int name) |
|
void | InputUpdateFromConstant (bool constant, int name) |
|
void | InputUpdateFromIoModel (int index, IoModel *iomodel) |
|
void | InputUpdateFromMatrixDakota (IssmDouble *matrix, int nrows, int ncols, int name, int type) |
|
void | InputUpdateFromVector (IssmDouble *vector, int name, int type) |
|
void | InputUpdateFromVectorDakota (IssmDouble *vector, int name, int type) |
|
void | Configure (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | CreateJacobianMatrix (Matrix< IssmDouble > *Jff) |
|
void | CreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs) |
|
void | CreatePVector (Vector< IssmDouble > *pf) |
|
void | GetNodesSidList (int *sidlist) |
|
void | GetNodesLidList (int *lidlist) |
|
int | GetNumberOfNodes (void) |
|
bool | IsPenalty (void) |
|
void | PenaltyCreateJacobianMatrix (Matrix< IssmDouble > *Jff, IssmDouble kmax) |
|
void | PenaltyCreateKMatrix (Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *kfs, IssmDouble kmax) |
|
void | PenaltyCreatePVector (Vector< IssmDouble > *pf, IssmDouble kmax) |
|
void | SetCurrentConfiguration (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | SetwiseNodeConnectivity (int *d_nz, int *o_nz, Node *node, bool *flags, int *flagsindices, int set1_enum, int set2_enum) |
|
void | ResetHooks () |
|
ElementMatrix * | CreateKMatrixHydrologyGlaDS (void) |
|
ElementVector * | CreatePVectorHydrologyShakti (void) |
|
ElementVector * | CreatePVectorHydrologyGlaDS (void) |
|
ElementVector * | CreatePVectorHydrologyDCInefficient (void) |
|
ElementVector * | CreatePVectorHydrologyDCEfficient (void) |
|
virtual | ~Load () |
|
virtual | ~Object () |
|
Definition at line 21 of file Moulin.h.
◆ Moulin() [1/2]
◆ Moulin() [2/2]
Moulin::Moulin |
( |
int |
index, |
|
|
int |
id, |
|
|
IoModel * |
iomodel |
|
) |
| |
Definition at line 31 of file Moulin.cpp.
34 int pengrid_element_id;
38 _assert_(index>=0 && index<iomodel->numberofvertices);
45 pengrid_node_id=index+1;
◆ ~Moulin()
◆ copy()
Object * Moulin::copy |
( |
void |
| ) |
|
|
virtual |
◆ DeepEcho()
void Moulin::DeepEcho |
( |
void |
| ) |
|
|
virtual |
◆ Echo()
void Moulin::Echo |
( |
void |
| ) |
|
|
virtual |
◆ Id()
◆ Marshall()
void Moulin::Marshall |
( |
char ** |
pmarshalled_data, |
|
|
int * |
pmarshalled_data_size, |
|
|
int |
marshall_direction |
|
) |
| |
|
virtual |
Implements Object.
Definition at line 111 of file Moulin.cpp.
125 this->
hnode->
Marshall(pmarshalled_data,pmarshalled_data_size,marshall_direction);
126 this->
hvertex->
Marshall(pmarshalled_data,pmarshalled_data_size,marshall_direction);
127 this->
helement->
Marshall(pmarshalled_data,pmarshalled_data_size,marshall_direction);
◆ ObjectEnum()
int Moulin::ObjectEnum |
( |
void |
| ) |
|
|
virtual |
◆ InputUpdateFromConstant() [1/3]
void Moulin::InputUpdateFromConstant |
( |
IssmDouble |
constant, |
|
|
int |
name |
|
) |
| |
◆ InputUpdateFromConstant() [2/3]
void Moulin::InputUpdateFromConstant |
( |
int |
constant, |
|
|
int |
name |
|
) |
| |
◆ InputUpdateFromConstant() [3/3]
void Moulin::InputUpdateFromConstant |
( |
bool |
constant, |
|
|
int |
name |
|
) |
| |
◆ InputUpdateFromIoModel()
void Moulin::InputUpdateFromIoModel |
( |
int |
index, |
|
|
IoModel * |
iomodel |
|
) |
| |
|
inline |
◆ InputUpdateFromMatrixDakota()
void Moulin::InputUpdateFromMatrixDakota |
( |
IssmDouble * |
matrix, |
|
|
int |
nrows, |
|
|
int |
ncols, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
◆ InputUpdateFromVector()
void Moulin::InputUpdateFromVector |
( |
IssmDouble * |
vector, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
◆ InputUpdateFromVectorDakota()
void Moulin::InputUpdateFromVectorDakota |
( |
IssmDouble * |
vector, |
|
|
int |
name, |
|
|
int |
type |
|
) |
| |
◆ Configure()
Implements Load.
Definition at line 142 of file Moulin.cpp.
156 this->parameters=parametersin;
◆ CreateJacobianMatrix()
◆ CreateKMatrix()
Implements Load.
Definition at line 159 of file Moulin.cpp.
166 switch(analysis_type){
180 _error_(
"Don't know why we should be here");
◆ CreatePVector()
Implements Load.
Definition at line 191 of file Moulin.cpp.
197 switch(analysis_type){
211 _error_(
"Don't know why we should be here");
◆ GetNodesSidList()
void Moulin::GetNodesSidList |
( |
int * |
sidlist | ) |
|
|
virtual |
◆ GetNodesLidList()
void Moulin::GetNodesLidList |
( |
int * |
lidlist | ) |
|
|
virtual |
◆ GetNumberOfNodes()
int Moulin::GetNumberOfNodes |
( |
void |
| ) |
|
|
virtual |
◆ IsPenalty()
bool Moulin::IsPenalty |
( |
void |
| ) |
|
|
virtual |
◆ PenaltyCreateJacobianMatrix()
◆ PenaltyCreateKMatrix()
◆ PenaltyCreatePVector()
◆ SetCurrentConfiguration()
◆ SetwiseNodeConnectivity()
void Moulin::SetwiseNodeConnectivity |
( |
int * |
d_nz, |
|
|
int * |
o_nz, |
|
|
Node * |
node, |
|
|
bool * |
flags, |
|
|
int * |
flagsindices, |
|
|
int |
set1_enum, |
|
|
int |
set2_enum |
|
) |
| |
|
virtual |
Implements Load.
Definition at line 275 of file Moulin.cpp.
281 if(!flags[this->node->
Lid()]){
284 flags[this->node->
Lid()]=
true;
287 while(flagsindices[counter]>=0) counter++;
288 flagsindices[counter]=this->node->
Lid();
316 default:
_error_(
"not supported");
◆ ResetHooks()
void Moulin::ResetHooks |
( |
| ) |
|
|
virtual |
◆ CreateKMatrixHydrologyGlaDS()
◆ CreatePVectorHydrologyShakti()
◆ CreatePVectorHydrologyGlaDS()
Definition at line 375 of file Moulin.cpp.
395 pe->
values[0] = moulin_load -Am/(rho_water*g) * phi_old/dt;
◆ CreatePVectorHydrologyDCInefficient()
ElementVector * Moulin::CreatePVectorHydrologyDCInefficient |
( |
void |
| ) |
|
Definition at line 419 of file Moulin.cpp.
424 bool isefficientlayer;
435 if(isefficientlayer){
437 if(reCast<bool>(epl_active)){
438 pe->
values[0]=moulin_load*0.0;
441 pe->
values[0]=moulin_load*dt;
445 pe->
values[0]=moulin_load*dt;
◆ CreatePVectorHydrologyDCEfficient()
ElementVector * Moulin::CreatePVectorHydrologyDCEfficient |
( |
void |
| ) |
|
◆ id
◆ hnode
◆ hvertex
◆ helement
◆ node
◆ vertex
◆ element
◆ parameters
The documentation for this class was generated from the following files:
ElementMatrix * CreateKMatrixHydrologyGlaDS(void)
ElementVector * CreatePVectorHydrologyDCInefficient(void)
void FindParam(bool *pvalue, int paramenum)
#define _printf_(StreamArgs)
@ HydrologydcMaskEplactiveNodeEnum
@ MaterialsRhoFreshwaterEnum
#define MARSHALLING_ENUM(EN)
@ TimesteppingTimeStepEnum
@ HydrologydcIsefficientlayerEnum
@ HydrologyShaktiAnalysisEnum
ElementVector * CreatePVectorHydrologyShakti(void)
@ HydrologyDCInefficientAnalysisEnum
@ HydrologyGlaDSAnalysisEnum
ElementVector * CreatePVectorHydrologyGlaDS(void)
@ HydrologyMoulinInputEnum
void configure(DataSet *dataset)
#define MARSHALLING(FIELD)
@ HydrologyDCEfficientAnalysisEnum
int * singlenodetoelementconnectivity
#define _error_(StreamArgs)
@ HydrologydcBasalMoulinInputEnum
void GetInputValue(bool *pvalue, int enum_type)
void FindParam(bool *pinteger, int enum_type)
void AddToGlobal(Vector< IssmDouble > *pf)
void AddToGlobal(Matrix< IssmDouble > *Kff, Matrix< IssmDouble > *Kfs)
ElementVector * CreatePVectorHydrologyDCEfficient(void)
@ HydraulicPotentialOldEnum
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)