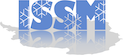 |
Ice Sheet System Model
4.18
Code documentation
|
#include <Matice.h>
|
| Matice () |
|
| Matice (int mid, int i, IoModel *iomodel) |
|
| Matice (int mid, int i, int materialtype) |
|
| ~Matice () |
|
void | Init (int mid, int i, int materialtype) |
|
Object * | copy () |
|
void | DeepEcho () |
|
void | Echo () |
|
int | Id () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | ObjectEnum () |
|
void | Configure (Elements *elements) |
|
Material * | copy2 (Element *element) |
|
void | GetViscosity (IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss) |
|
void | GetViscosityBar (IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss) |
|
void | GetViscosityComplement (IssmDouble *pviscosity_complement, IssmDouble *pepsilon, Gauss *gauss) |
|
void | GetViscosityDComplement (IssmDouble *, IssmDouble *, Gauss *gauss) |
|
void | GetViscosityDerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *pepsilon, Gauss *gauss) |
|
void | GetViscosity_B (IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss) |
|
void | GetViscosity_D (IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss) |
|
void | GetViscosity2dDerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *pepsilon, Gauss *gauss) |
|
IssmDouble | GetA (Gauss *gauss) |
|
IssmDouble | GetAbar (Gauss *gauss) |
|
IssmDouble | GetB (Gauss *gauss) |
|
IssmDouble | GetBbar (Gauss *gauss) |
|
IssmDouble | GetD (Gauss *gauss) |
|
IssmDouble | GetDbar (Gauss *gauss) |
|
IssmDouble | GetE (Gauss *gauss) |
|
IssmDouble | GetEbar (Gauss *gauss) |
|
IssmDouble | GetN () |
|
bool | IsDamage () |
|
bool | IsEnhanced () |
|
void | ResetHooks () |
|
void | SetCurrentConfiguration (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | ViscosityFSDerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *epsilon, Gauss *gauss) |
|
void | ViscosityHODerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *epsilon, Gauss *gauss) |
|
void | ViscositySSADerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *epsilon, Gauss *gauss) |
|
void | ViscosityFS (IssmDouble *pviscosity, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, Input2 *vz_input) |
|
void | ViscosityHO (IssmDouble *pviscosity, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input) |
|
void | ViscosityL1L2 (IssmDouble *pviscosity, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, Input2 *surf) |
|
void | ViscositySSA (IssmDouble *pviscosity, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input) |
|
void | ViscosityBFS (IssmDouble *pmudB, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, Input2 *vz_input, IssmDouble eps_eff) |
|
void | ViscosityBHO (IssmDouble *pmudB, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, IssmDouble eps_eff) |
|
void | ViscosityBSSA (IssmDouble *pmudB, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, IssmDouble eps_eff) |
|
virtual | ~Material () |
|
virtual | ~Object () |
|
Definition at line 24 of file Matice.h.
◆ Matice() [1/3]
◆ Matice() [2/3]
Matice::Matice |
( |
int |
mid, |
|
|
int |
i, |
|
|
IoModel * |
iomodel |
|
) |
| |
Definition at line 32 of file Matice.cpp.
36 iomodel->
FindConstant(&materialtype,
"md.materials.type");
37 this->
Init(matice_mid,index,materialtype);
◆ Matice() [3/3]
Matice::Matice |
( |
int |
mid, |
|
|
int |
i, |
|
|
int |
materialtype |
|
) |
| |
Definition at line 41 of file Matice.cpp.
43 this->
Init(matice_mid,index,materialtype);
◆ ~Matice()
◆ Init()
void Matice::Init |
( |
int |
mid, |
|
|
int |
i, |
|
|
int |
materialtype |
|
) |
| |
Definition at line 51 of file Matice.cpp.
57 int matice_eid=index+1;
76 _error_(
"Material type not recognized");
◆ copy()
Object * Matice::copy |
( |
void |
| ) |
|
|
virtual |
◆ DeepEcho()
void Matice::DeepEcho |
( |
void |
| ) |
|
|
virtual |
Implements Object.
Definition at line 119 of file Matice.cpp.
129 _printf_(
" note: helement not printed to avoid recursion.\n");
134 _printf_(
" note: element not printed to avoid recursion.\n");
◆ Echo()
void Matice::Echo |
( |
void |
| ) |
|
|
virtual |
Implements Object.
Definition at line 139 of file Matice.cpp.
149 _printf_(
" note: helement not printed to avoid recursion.\n");
154 _printf_(
" note: element not printed to avoid recursion.\n");
◆ Id()
◆ Marshall()
void Matice::Marshall |
( |
char ** |
pmarshalled_data, |
|
|
int * |
pmarshalled_data_size, |
|
|
int |
marshall_direction |
|
) |
| |
|
virtual |
◆ ObjectEnum()
int Matice::ObjectEnum |
( |
void |
| ) |
|
|
virtual |
◆ Configure()
void Matice::Configure |
( |
Elements * |
elements | ) |
|
|
virtual |
◆ copy2()
◆ GetViscosity()
Implements Material.
Definition at line 304 of file Matice.cpp.
337 viscosity=(1.-D)*B/(2.*E);
343 viscosity = 1.e+14/2.;
349 viscosity=(1.-D)*B/(2.*pow(E,1./n)*pow(eps_eff,(n-1.)/n));
354 if(viscosity<=0)
_error_(
"Negative viscosity");
357 *pviscosity=viscosity;
◆ GetViscosityBar()
Implements Material.
Definition at line 360 of file Matice.cpp.
393 viscosity=(1.-D)*B/(2.*E);
399 viscosity = 1.e+14/2.;
404 viscosity=(1.-D)*B/(2.*pow(E,1./n)*pow(eps_eff,(n-1.)/n));
409 if(viscosity<=0)
_error_(
"Negative viscosity");
412 *pviscosity=viscosity;
◆ GetViscosityComplement()
Implements Material.
Definition at line 415 of file Matice.cpp.
449 A=pow(exx,2)+pow(eyy,2)+pow(exy,2)+exx*eyy;
452 viscosity_complement=2.25*pow(10.,17);
457 viscosity_complement=(1-D)/(2*pow(A,e));
461 viscosity_complement=4.5*pow(10.,17);
470 *pviscosity_complement=viscosity_complement;
◆ GetViscosityDComplement()
Implements Material.
Definition at line 473 of file Matice.cpp.
504 A=pow(exx,2)+pow(eyy,2)+pow(exy,2)+exx*eyy;
507 viscosity_complement=- 2.25*pow(10.,17);
512 viscosity_complement=- B/(2*pow(A,e));
516 viscosity_complement=- 4.5*pow(10.,17);
525 *pviscosity_complement=viscosity_complement;
◆ GetViscosityDerivativeEpsSquare()
Implements Material.
Definition at line 528 of file Matice.cpp.
537 if((epsilon[0]==0) && (epsilon[1]==0) && (epsilon[2]==0) &&
538 (epsilon[3]==0) && (epsilon[4]==0)){
539 mu_prime=0.5*pow(10.,14);
549 eff2 = exx*exx + eyy*eyy + exx*eyy + exy*exy + exz*exz + eyz*eyz;
553 mu_prime=(1.-n)/(2.*n) * mu/eff2;
◆ GetViscosity_B()
Implements Material.
Definition at line 560 of file Matice.cpp.
584 if(eps_eff==0.) dmudB = 0.;
585 else dmudB = (1.-D)/(2.*pow(E,1./n)*pow(eps_eff,(n-1.)/n));
◆ GetViscosity_D()
Implements Material.
Definition at line 592 of file Matice.cpp.
614 if(eps_eff==0.) dmudD = 0.;
615 else dmudD = -B/(2.*pow(E,1./n)*pow(eps_eff,(n-1.)/n));
◆ GetViscosity2dDerivativeEpsSquare()
Implements Material.
Definition at line 622 of file Matice.cpp.
631 if((epsilon[0]==0) && (epsilon[1]==0) && (epsilon[2]==0)){
632 mu_prime=0.5*pow(10.,14);
639 eff2 = exx*exx + eyy*eyy + exx*eyy + exy*exy ;
643 mu_prime=(1.-n)/(2.*n)*mu/eff2;
◆ GetA()
◆ GetAbar()
◆ GetB()
◆ GetBbar()
◆ GetD()
◆ GetDbar()
◆ GetE()
◆ GetEbar()
◆ GetN()
◆ IsDamage()
bool Matice::IsDamage |
( |
| ) |
|
|
virtual |
◆ IsEnhanced()
bool Matice::IsEnhanced |
( |
| ) |
|
|
virtual |
◆ ResetHooks()
void Matice::ResetHooks |
( |
| ) |
|
|
virtual |
◆ SetCurrentConfiguration()
◆ ViscosityFSDerivativeEpsSquare()
◆ ViscosityHODerivativeEpsSquare()
◆ ViscositySSADerivativeEpsSquare()
◆ ViscosityFS()
Implements Material.
Definition at line 673 of file Matice.cpp.
697 eps_eff = sqrt(epsilon3d[0]*epsilon3d[0] + epsilon3d[1]*epsilon3d[1] + epsilon3d[3]*epsilon3d[3] + epsilon3d[4]*epsilon3d[4] + epsilon3d[5]*epsilon3d[5] + epsilon3d[0]*epsilon3d[1]+eps0*eps0);
702 eps_eff = 1./sqrt(2.)*sqrt(epsilon2d[0]*epsilon2d[0] + epsilon2d[1]*epsilon2d[1] + 2.*epsilon2d[2]*epsilon2d[2]);
709 *pviscosity=viscosity;
◆ ViscosityHO()
Implements Material.
Definition at line 712 of file Matice.cpp.
723 eps_eff = sqrt(epsilon3d[0]*epsilon3d[0] + epsilon3d[1]*epsilon3d[1] + epsilon3d[2]*epsilon3d[2] + epsilon3d[3]*epsilon3d[3] + epsilon3d[4]*epsilon3d[4] + epsilon3d[0]*epsilon3d[1]);
728 eps_eff = 1./sqrt(2.)*sqrt(2*epsilon2d[0]*epsilon2d[0] + 2*epsilon2d[1]*epsilon2d[1]);
733 _assert_(!xIsNan<IssmDouble>(viscosity));
736 *pviscosity=viscosity;
◆ ViscosityL1L2()
Implements Material.
Definition at line 738 of file Matice.cpp.
762 if (!vx_input || !vy_input || !surface_input)
_error_(
"Input missing");
765 surface_input->GetInputValue(&s,gauss);
766 surface_input->GetInputDerivativeValue(&slope[0],xyz_list,gauss);
772 eps_b = sqrt(epsilon[0]*epsilon[0] + epsilon[1]*epsilon[1] + epsilon[0]*epsilon[1] + epsilon[2]*epsilon[2]);
774 *pviscosity = 2.5e+17;
783 p = tau_perp *tau_perp;
785 delta = q *q + p*p*p*4./27.;
787 tau_par = pow(0.5*(-q+sqrt(delta)),1./3.) - pow(0.5*(q+sqrt(delta)),1./3.);
790 viscosity = 1./(2.*A*(tau_par*tau_par + tau_perp*tau_perp));
795 *pviscosity = viscosity;
◆ ViscositySSA()
Implements Material.
Definition at line 797 of file Matice.cpp.
808 eps_eff = sqrt(epsilon2d[0]*epsilon2d[0] + epsilon2d[1]*epsilon2d[1] + epsilon2d[2]*epsilon2d[2] + epsilon2d[0]*epsilon2d[1]);
813 eps_eff = fabs(epsilon1d);
820 *pviscosity=viscosity;
◆ ViscosityBFS()
◆ ViscosityBHO()
◆ ViscosityBSSA()
◆ mid
◆ isdamaged
◆ isenhanced
◆ rheology_law
◆ helement
◆ element
The documentation for this class was generated from the following files:
void Init(int mid, int i, int materialtype)
void FindParam(bool *pvalue, int paramenum)
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
@ MaterialsRheologyEbarEnum
void StrainRateFS(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, Input2 *vz_input)
void GetViscosity2dDerivativeEpsSquare(IssmDouble *pmu_prime, IssmDouble *pepsilon, Gauss *gauss)
IssmDouble GetZcoord(IssmDouble *xyz_list, Gauss *gauss)
void GetViscosityDerivativeEpsSquare(IssmDouble *pmu_prime, IssmDouble *pepsilon, Gauss *gauss)
IssmDouble GetBbar(Gauss *gauss)
IssmDouble GetDbar(Gauss *gauss)
IssmDouble GetB(Gauss *gauss)
@ MaterialsRheologyBbarEnum
virtual Input2 * GetInput2(int inputenum)=0
IssmDouble GetEbar(Gauss *gauss)
void GetViscosity(IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss)
void configure(DataSet *dataset)
void FindConstant(bool *pvalue, const char *constant_name)
#define MARSHALLING(FIELD)
void StrainRateSSA1d(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input)
IssmDouble GetE(Gauss *gauss)
void StrainRateHO(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input)
#define _error_(StreamArgs)
IssmDouble GetD(Gauss *gauss)
void StrainRateSSA(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input)
void StrainRateHO2dvertical(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input)
IssmDouble GetA(Gauss *gauss)
Declaration of DataSet class.
void GetViscosityBar(IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)