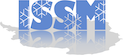 |
Ice Sheet System Model
4.18
Code documentation
|
#include <Matestar.h>
|
| Matestar () |
|
| Matestar (int mid, int i, IoModel *iomodel) |
|
| ~Matestar () |
|
Object * | copy () |
|
void | DeepEcho () |
|
void | Echo () |
|
int | Id () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | ObjectEnum () |
|
void | Configure (Elements *elements) |
|
Material * | copy2 (Element *element) |
|
void | GetViscosity (IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss) |
|
void | GetViscosityBar (IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss) |
|
void | GetViscosityComplement (IssmDouble *pviscosity_complement, IssmDouble *pepsilon, Gauss *gauss) |
|
void | GetViscosityDComplement (IssmDouble *, IssmDouble *, Gauss *gauss) |
|
void | GetViscosityDerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *pepsilon, Gauss *gauss) |
|
void | GetViscosity_B (IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss) |
|
void | GetViscosity_D (IssmDouble *pviscosity, IssmDouble eps_eff, Gauss *gauss) |
|
void | GetViscosity2dDerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *pepsilon, Gauss *gauss) |
|
IssmDouble | GetA (Gauss *gauss) |
|
IssmDouble | GetAbar (Gauss *gauss) |
|
IssmDouble | GetB (Gauss *gauss) |
|
IssmDouble | GetBbar (Gauss *gauss) |
|
IssmDouble | GetD (Gauss *gauss) |
|
IssmDouble | GetDbar (Gauss *gauss) |
|
IssmDouble | GetEc (Gauss *gauss) |
|
IssmDouble | GetEcbar (Gauss *gauss) |
|
IssmDouble | GetEs (Gauss *gauss) |
|
IssmDouble | GetEsbar (Gauss *gauss) |
|
IssmDouble | GetN () |
|
bool | IsDamage () |
|
bool | IsEnhanced () |
|
void | ResetHooks () |
|
void | SetCurrentConfiguration (Elements *elements, Loads *loads, Nodes *nodes, Vertices *vertices, Materials *materials, Parameters *parameters) |
|
void | ViscosityFSDerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *epsilon, Gauss *gauss) |
|
void | ViscosityHODerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *epsilon, Gauss *gauss) |
|
void | ViscositySSADerivativeEpsSquare (IssmDouble *pmu_prime, IssmDouble *epsilon, Gauss *gauss) |
|
void | ViscosityFS (IssmDouble *pviscosity, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, Input2 *vz_input) |
|
void | ViscosityHO (IssmDouble *pviscosity, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input) |
|
void | ViscosityL1L2 (IssmDouble *pviscosity, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, Input2 *surf) |
|
void | ViscositySSA (IssmDouble *pviscosity, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input) |
|
void | ViscosityBFS (IssmDouble *pmudB, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, Input2 *vz_input, IssmDouble eps_eff) |
|
void | ViscosityBHO (IssmDouble *pmudB, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, IssmDouble eps_eff) |
|
void | ViscosityBSSA (IssmDouble *pmudB, int dim, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, IssmDouble eps_eff) |
|
IssmDouble | GetViscosityGeneral (IssmDouble vx, IssmDouble vy, IssmDouble vz, IssmDouble *dvx, IssmDouble *dvy, IssmDouble *dvz, IssmDouble eps_eff, bool isdepthaveraged, Gauss *gauss) |
|
IssmDouble | GetViscosity_BGeneral (IssmDouble vx, IssmDouble vy, IssmDouble vz, IssmDouble *dvx, IssmDouble *dvy, IssmDouble *dvz, IssmDouble eps_eff, bool isdepthaveraged, Gauss *gauss) |
|
virtual | ~Material () |
|
virtual | ~Object () |
|
Definition at line 24 of file Matestar.h.
◆ Matestar() [1/2]
◆ Matestar() [2/2]
Matestar::Matestar |
( |
int |
mid, |
|
|
int |
i, |
|
|
IoModel * |
iomodel |
|
) |
| |
◆ ~Matestar()
◆ copy()
Object * Matestar::copy |
( |
void |
| ) |
|
|
virtual |
◆ DeepEcho()
void Matestar::DeepEcho |
( |
void |
| ) |
|
|
virtual |
◆ Echo()
void Matestar::Echo |
( |
void |
| ) |
|
|
virtual |
◆ Id()
int Matestar::Id |
( |
void |
| ) |
|
|
virtual |
◆ Marshall()
void Matestar::Marshall |
( |
char ** |
pmarshalled_data, |
|
|
int * |
pmarshalled_data_size, |
|
|
int |
marshall_direction |
|
) |
| |
|
virtual |
◆ ObjectEnum()
int Matestar::ObjectEnum |
( |
void |
| ) |
|
|
virtual |
◆ Configure()
void Matestar::Configure |
( |
Elements * |
elements | ) |
|
|
virtual |
◆ copy2()
◆ GetViscosity()
◆ GetViscosityBar()
◆ GetViscosityComplement()
◆ GetViscosityDComplement()
◆ GetViscosityDerivativeEpsSquare()
◆ GetViscosity_B()
◆ GetViscosity_D()
◆ GetViscosity2dDerivativeEpsSquare()
◆ GetA()
◆ GetAbar()
◆ GetB()
◆ GetBbar()
◆ GetD()
◆ GetDbar()
◆ GetEc()
◆ GetEcbar()
◆ GetEs()
◆ GetEsbar()
◆ GetN()
◆ IsDamage()
bool Matestar::IsDamage |
( |
| ) |
|
|
virtual |
◆ IsEnhanced()
bool Matestar::IsEnhanced |
( |
| ) |
|
|
inlinevirtual |
◆ ResetHooks()
void Matestar::ResetHooks |
( |
| ) |
|
|
virtual |
◆ SetCurrentConfiguration()
◆ ViscosityFSDerivativeEpsSquare()
◆ ViscosityHODerivativeEpsSquare()
◆ ViscositySSADerivativeEpsSquare()
◆ ViscosityFS()
Implements Material.
Definition at line 482 of file Matestar.cpp.
490 bool isdepthaveraged=0.;
507 dvz[0] = 0.; dvz[1] = 0.; dvz[2] = 0.;
513 eps_eff = sqrt(epsilon3d[0]*epsilon3d[0] + epsilon3d[1]*epsilon3d[1] + epsilon3d[3]*epsilon3d[3] + epsilon3d[4]*epsilon3d[4] + epsilon3d[5]*epsilon3d[5] + epsilon3d[0]*epsilon3d[1]+eps0*eps0);
518 eps_eff = 1./sqrt(2.)*sqrt(epsilon2d[0]*epsilon2d[0] + epsilon2d[1]*epsilon2d[1] + 2.*epsilon2d[2]*epsilon2d[2]);
522 *pviscosity=
GetViscosityGeneral(vx,vy,vz,&dvx[0],&dvy[0],&dvz[0],eps_eff,isdepthaveraged,gauss);
◆ ViscosityHO()
Implements Material.
Definition at line 525 of file Matestar.cpp.
533 bool isdepthaveraged=0.;
538 eps_eff = sqrt(epsilon3d[0]*epsilon3d[0] + epsilon3d[1]*epsilon3d[1] + epsilon3d[2]*epsilon3d[2] + epsilon3d[3]*epsilon3d[3] + epsilon3d[4]*epsilon3d[4] + epsilon3d[0]*epsilon3d[1]);
543 eps_eff = 1./sqrt(2.)*sqrt(2*epsilon2d[0]*epsilon2d[0] + 2*epsilon2d[1]*epsilon2d[1]);
559 dvy[0] = 0.; dvy[1] = 0.; dvy[2] = 0.;
562 dvz[0] = 0.; dvz[1] = 0.; dvz[2] = -dvx[0]-dvy[1];
565 *pviscosity=
GetViscosityGeneral(vx,vy,vz,&dvx[0],&dvy[0],&dvz[0],eps_eff,isdepthaveraged,gauss);
◆ ViscosityL1L2()
◆ ViscositySSA()
Implements Material.
Definition at line 570 of file Matestar.cpp.
578 bool isdepthaveraged=1.;
594 dvy[0] = 0.; dvy[1] = 0.; dvy[2] = 0.;
599 dvz[0] = 0.; dvz[1] = 0.; dvz[2] = -dvx[0]-dvy[1];
604 eps_eff = sqrt(epsilon2d[0]*epsilon2d[0] + epsilon2d[1]*epsilon2d[1] + epsilon2d[2]*epsilon2d[2] + epsilon2d[0]*epsilon2d[1]);
609 eps_eff = fabs(epsilon1d);
613 *pviscosity=
GetViscosityGeneral(vx,vy,vz,&dvx[0],&dvy[0],&dvz[0],eps_eff,isdepthaveraged,gauss);
◆ ViscosityBFS()
Implements Material.
Definition at line 395 of file Matestar.cpp.
400 bool isdepthaveraged=0.;
417 dvz[0] = 0.; dvz[1] = 0.; dvz[2] = 0.;
◆ ViscosityBHO()
Implements Material.
Definition at line 424 of file Matestar.cpp.
429 bool isdepthaveraged=0.;
444 dvy[0] = 0.; dvy[1] = 0.; dvy[2] = 0.;
447 dvz[0] = 0.; dvz[1] = 0.; dvz[2] = -dvx[0]-dvy[1];
◆ ViscosityBSSA()
Implements Material.
Definition at line 452 of file Matestar.cpp.
456 bool isdepthaveraged=1.;
472 dvy[0] = 0.; dvy[1] = 0.; dvy[2] = 0.;
477 dvz[0] = 0.; dvz[1] = 0.; dvz[2] = -dvx[0]-dvy[1];
◆ GetViscosityGeneral()
Definition at line 248 of file Matestar.cpp.
260 vmag = sqrt(vx*vx+vy*vy+vz*vz);
267 dvmag[0]=1./(2*sqrt(vmag))*(2*vx*dvx[0]+2*vy*dvy[0]+2*vz*dvz[0]);
268 dvmag[1]=1./(2*sqrt(vmag))*(2*vx*dvx[1]+2*vy*dvy[1]+2*vz*dvz[1]);
269 dvmag[2]=1./(2*sqrt(vmag))*(2*vx*dvx[2]+2*vy*dvy[2]+2*vz*dvz[2]);
277 if (isdepthaveraged==0.){
288 E = Ec + (Es-Ec)*lambdas*lambdas;
_assert_(E>0.);
293 viscosity = 1.e+14/2.;
298 viscosity = B/(2.*pow(E,1./n)*pow(eps_eff,2./n));
302 if(viscosity<=0)
_error_(
"Negative viscosity");
◆ GetViscosity_BGeneral()
Definition at line 308 of file Matestar.cpp.
318 vmag = sqrt(vx*vx+vy*vy+vz*vz);
325 dvmag[0]=1./(2*sqrt(vmag))*(2*vx*dvx[0]+2*vy*dvy[0]+2*vz*dvz[0]);
326 dvmag[1]=1./(2*sqrt(vmag))*(2*vx*dvx[1]+2*vy*dvy[1]+2*vz*dvz[1]);
327 dvmag[2]=1./(2*sqrt(vmag))*(2*vx*dvx[2]+2*vy*dvy[2]+2*vz*dvz[2]);
334 if (isdepthaveraged==0.){
344 E = Ec + (Es-Ec)*lambdas*lambdas;
_assert_(E>0.);
347 if(eps_eff==0.) dmudB = 0.;
348 else dmudB = 1./(2.*pow(E,1./3.)*pow(eps_eff,2./3.));
◆ mid
◆ helement
◆ element
◆ rheology_law
int Matestar::rheology_law |
|
private |
The documentation for this class was generated from the following files:
IssmDouble EstarLambdaS(IssmDouble epseff, IssmDouble epsprime_norm)
@ MaterialsRheologyEsbarEnum
IssmDouble GetViscosityGeneral(IssmDouble vx, IssmDouble vy, IssmDouble vz, IssmDouble *dvx, IssmDouble *dvy, IssmDouble *dvz, IssmDouble eps_eff, bool isdepthaveraged, Gauss *gauss)
@ MaterialsRheologyEcEnum
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
IssmDouble GetEcbar(Gauss *gauss)
IssmDouble GetEc(Gauss *gauss)
void EstarStrainrateQuantities(IssmDouble *pepsprime_norm, IssmDouble vx, IssmDouble vy, IssmDouble vz, IssmDouble vmag, IssmDouble *dvx, IssmDouble *dvy, IssmDouble *dvz, IssmDouble *dvmag)
void StrainRateFS(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input, Input2 *vz_input)
IssmDouble GetBbar(Gauss *gauss)
@ MaterialsRheologyBbarEnum
virtual Input2 * GetInput2(int inputenum)=0
IssmDouble GetB(Gauss *gauss)
IssmDouble GetEs(Gauss *gauss)
void configure(DataSet *dataset)
IssmDouble GetViscosity_BGeneral(IssmDouble vx, IssmDouble vy, IssmDouble vz, IssmDouble *dvx, IssmDouble *dvy, IssmDouble *dvz, IssmDouble eps_eff, bool isdepthaveraged, Gauss *gauss)
#define MARSHALLING(FIELD)
void StrainRateSSA1d(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input)
@ MaterialsRheologyEsEnum
void GetViscosityDerivativeEpsSquare(IssmDouble *pmu_prime, IssmDouble *pepsilon, Gauss *gauss)
void StrainRateHO(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input)
#define _error_(StreamArgs)
void StrainRateSSA(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input)
@ MaterialsRheologyEcbarEnum
void StrainRateHO2dvertical(IssmDouble *epsilon, IssmDouble *xyz_list, Gauss *gauss, Input2 *vx_input, Input2 *vy_input)
Declaration of DataSet class.
IssmDouble GetEsbar(Gauss *gauss)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)