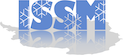 |
Ice Sheet System Model
4.18
Code documentation
|
#include <L2ProjectionEPLAnalysis.h>
|
void | CreateConstraints (Constraints *constraints, IoModel *iomodel) |
|
void | CreateLoads (Loads *loads, IoModel *iomodel) |
|
void | CreateNodes (Nodes *nodes, IoModel *iomodel, bool isamr=false) |
|
int | DofsPerNode (int **doflist, int domaintype, int approximation) |
|
void | UpdateElements (Elements *elements, Inputs2 *inputs2, IoModel *iomodel, int analysis_counter, int analysis_type) |
|
void | UpdateParameters (Parameters *parameters, IoModel *iomodel, int solution_enum, int analysis_enum) |
|
void | Core (FemModel *femmodel) |
|
ElementVector * | CreateDVector (Element *element) |
|
ElementMatrix * | CreateJacobianMatrix (Element *element) |
|
ElementMatrix * | CreateKMatrix (Element *element) |
|
ElementVector * | CreatePVector (Element *element) |
|
void | GetSolutionFromInputs (Vector< IssmDouble > *solution, Element *element) |
|
void | GradientJ (Vector< IssmDouble > *gradient, Element *element, int control_type, int control_index) |
|
void | InputUpdateFromSolution (IssmDouble *solution, Element *element) |
|
void | UpdateConstraints (FemModel *femmodel) |
|
virtual | ~Analysis () |
|
Definition at line 11 of file L2ProjectionEPLAnalysis.h.
◆ CreateConstraints()
void L2ProjectionEPLAnalysis::CreateConstraints |
( |
Constraints * |
constraints, |
|
|
IoModel * |
iomodel |
|
) |
| |
|
virtual |
◆ CreateLoads()
void L2ProjectionEPLAnalysis::CreateLoads |
( |
Loads * |
loads, |
|
|
IoModel * |
iomodel |
|
) |
| |
|
virtual |
◆ CreateNodes()
void L2ProjectionEPLAnalysis::CreateNodes |
( |
Nodes * |
nodes, |
|
|
IoModel * |
iomodel, |
|
|
bool |
isamr = false |
|
) |
| |
|
virtual |
◆ DofsPerNode()
int L2ProjectionEPLAnalysis::DofsPerNode |
( |
int ** |
doflist, |
|
|
int |
domaintype, |
|
|
int |
approximation |
|
) |
| |
|
virtual |
◆ UpdateElements()
void L2ProjectionEPLAnalysis::UpdateElements |
( |
Elements * |
elements, |
|
|
Inputs2 * |
inputs2, |
|
|
IoModel * |
iomodel, |
|
|
int |
analysis_counter, |
|
|
int |
analysis_type |
|
) |
| |
|
virtual |
◆ UpdateParameters()
void L2ProjectionEPLAnalysis::UpdateParameters |
( |
Parameters * |
parameters, |
|
|
IoModel * |
iomodel, |
|
|
int |
solution_enum, |
|
|
int |
analysis_enum |
|
) |
| |
|
virtual |
◆ Core()
void L2ProjectionEPLAnalysis::Core |
( |
FemModel * |
femmodel | ) |
|
|
virtual |
◆ CreateDVector()
◆ CreateJacobianMatrix()
◆ CreateKMatrix()
Implements Analysis.
Definition at line 83 of file L2ProjectionEPLAnalysis.cpp.
94 basalelement = element;
97 if(!element->
IsOnBase())
return NULL;
101 if(!element->
IsOnBase())
return NULL;
127 IssmDouble* basis = xNew<IssmDouble>(numnodes);
134 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
148 xDelete<IssmDouble>(xyz_list);
149 xDelete<IssmDouble>(basis);
◆ CreatePVector()
Implements Analysis.
Definition at line 154 of file L2ProjectionEPLAnalysis.cpp.
165 basalelement = element;
168 if(!element->
IsOnBase())
return NULL;
177 if(!active_element) {
186 int input_enum,index;
196 IssmDouble* basis = xNew<IssmDouble>(numnodes);
204 default:
_error_(
"not implemented");
209 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
216 for(
int i=0;i<numnodes;i++) pe->
values[i]+=Jdet*gauss->
weight*slopes[index]*basis[i];
220 xDelete<IssmDouble>(xyz_list);
221 xDelete<IssmDouble>(basis);
◆ GetSolutionFromInputs()
◆ GradientJ()
void L2ProjectionEPLAnalysis::GradientJ |
( |
Vector< IssmDouble > * |
gradient, |
|
|
Element * |
element, |
|
|
int |
control_type, |
|
|
int |
control_index |
|
) |
| |
|
virtual |
◆ InputUpdateFromSolution()
void L2ProjectionEPLAnalysis::InputUpdateFromSolution |
( |
IssmDouble * |
solution, |
|
|
Element * |
element |
|
) |
| |
|
virtual |
◆ UpdateConstraints()
void L2ProjectionEPLAnalysis::UpdateConstraints |
( |
FemModel * |
femmodel | ) |
|
|
virtual |
The documentation for this class was generated from the following files:
virtual int GetNumberOfNodes(void)=0
void FindParam(bool *pvalue, int paramenum)
virtual Element * SpawnBasalElement(void)=0
int TripleMultiply(IssmDouble *a, int nrowa, int ncola, int itrna, IssmDouble *b, int nrowb, int ncolb, int itrnb, IssmDouble *c, int nrowc, int ncolc, int itrnc, IssmDouble *d, int iaddd)
virtual Input2 * GetInput2(int inputenum)=0
void DeleteMaterials(void)
virtual void NodalFunctions(IssmDouble *basis, Gauss *gauss)=0
ElementVector * NewElementVector(int approximation_enum=NoneApproximationEnum)
void DeleteData(int num,...)
virtual Gauss * NewGauss(void)=0
virtual void InputUpdateFromSolutionOneDof(IssmDouble *solution, int inputenum)=0
const char * EnumToStringx(int enum_in)
void FindConstant(bool *pvalue, const char *constant_name)
void GetInput2Value(bool *pvalue, int enum_type)
void GetVerticesCoordinates(IssmDouble **xyz_list)
void FetchData(bool *pboolean, const char *data_name)
@ L2ProjectionEPLAnalysisEnum
#define _error_(StreamArgs)
virtual int begin(void)=0
Object * GetObjectByOffset(int offset)
@ MeshVertexonsurfaceEnum
virtual void JacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, Gauss *gauss)=0
virtual void GaussPoint(int ig)=0
virtual void Update(Inputs2 *inputs2, int index, IoModel *iomodel, int analysis_counter, int analysis_type, int finite_element)=0
void CreateNodes(Nodes *nodes, IoModel *iomodel, bool isamr=false)
void FetchDataToInput(Inputs2 *inputs2, Elements *elements, const char *vector_name, int input_enum)
virtual void InputUpdateFromSolutionOneDofCollapsed(IssmDouble *solution, int inputenum)=0
@ HydrologydcMaskEplactiveEltEnum
ElementMatrix * NewElementMatrix(int approximation_enum=NoneApproximationEnum)