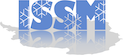 |
Ice Sheet System Model
4.18
Code documentation
|
#include <FreeSurfaceTopAnalysis.h>
|
void | CreateConstraints (Constraints *constraints, IoModel *iomodel) |
|
void | CreateLoads (Loads *loads, IoModel *iomodel) |
|
void | CreateNodes (Nodes *nodes, IoModel *iomodel, bool isamr=false) |
|
int | DofsPerNode (int **doflist, int domaintype, int approximation) |
|
void | UpdateElements (Elements *elements, Inputs2 *inputs2, IoModel *iomodel, int analysis_counter, int analysis_type) |
|
void | UpdateParameters (Parameters *parameters, IoModel *iomodel, int solution_enum, int analysis_enum) |
|
void | Core (FemModel *femmodel) |
|
ElementVector * | CreateDVector (Element *element) |
|
ElementMatrix * | CreateJacobianMatrix (Element *element) |
|
ElementMatrix * | CreateKMatrix (Element *element) |
|
ElementVector * | CreatePVector (Element *element) |
|
void | GetB (IssmDouble *B, Element *element, int dim, IssmDouble *xyz_list, Gauss *gauss) |
|
void | GetBprime (IssmDouble *B, Element *element, int dim, IssmDouble *xyz_list, Gauss *gauss) |
|
void | GetSolutionFromInputs (Vector< IssmDouble > *solution, Element *element) |
|
void | GradientJ (Vector< IssmDouble > *gradient, Element *element, int control_type, int control_index) |
|
void | InputUpdateFromSolution (IssmDouble *solution, Element *element) |
|
void | UpdateConstraints (FemModel *femmodel) |
|
virtual | ~Analysis () |
|
Definition at line 11 of file FreeSurfaceTopAnalysis.h.
◆ CreateConstraints()
void FreeSurfaceTopAnalysis::CreateConstraints |
( |
Constraints * |
constraints, |
|
|
IoModel * |
iomodel |
|
) |
| |
|
virtual |
◆ CreateLoads()
void FreeSurfaceTopAnalysis::CreateLoads |
( |
Loads * |
loads, |
|
|
IoModel * |
iomodel |
|
) |
| |
|
virtual |
Implements Analysis.
Definition at line 10 of file FreeSurfaceTopAnalysis.cpp.
15 int numvertex_pairing;
20 iomodel->
FetchData(&vertex_pairing,&numvertex_pairing,NULL,
"md.masstransport.vertex_pairing");
22 for(
int i=0;i<numvertex_pairing;i++){
24 if(iomodel->
my_vertices[reCast<int>(vertex_pairing[2*i+0])-1]){
31 if(!(reCast<bool>(nodeonsurface[reCast<int>(vertex_pairing[2*i+0])-1])) || !(reCast<bool>(nodeonsurface[reCast<int>(vertex_pairing[2*i+1])-1])))
continue;
35 penpair_ids[0]=reCast<int>(vertex_pairing[2*i+0]);
36 penpair_ids[1]=reCast<int>(vertex_pairing[2*i+1]);
45 iomodel->
DeleteData(vertex_pairing,
"md.masstransport.vertex_pairing");
46 iomodel->
DeleteData(nodeonsurface,
"md.mesh.vertexonsurface");
◆ CreateNodes()
void FreeSurfaceTopAnalysis::CreateNodes |
( |
Nodes * |
nodes, |
|
|
IoModel * |
iomodel, |
|
|
bool |
isamr = false |
|
) |
| |
|
virtual |
◆ DofsPerNode()
int FreeSurfaceTopAnalysis::DofsPerNode |
( |
int ** |
doflist, |
|
|
int |
domaintype, |
|
|
int |
approximation |
|
) |
| |
|
virtual |
◆ UpdateElements()
void FreeSurfaceTopAnalysis::UpdateElements |
( |
Elements * |
elements, |
|
|
Inputs2 * |
inputs2, |
|
|
IoModel * |
iomodel, |
|
|
int |
analysis_counter, |
|
|
int |
analysis_type |
|
) |
| |
|
virtual |
◆ UpdateParameters()
void FreeSurfaceTopAnalysis::UpdateParameters |
( |
Parameters * |
parameters, |
|
|
IoModel * |
iomodel, |
|
|
int |
solution_enum, |
|
|
int |
analysis_enum |
|
) |
| |
|
virtual |
◆ Core()
void FreeSurfaceTopAnalysis::Core |
( |
FemModel * |
femmodel | ) |
|
|
virtual |
◆ CreateDVector()
◆ CreateJacobianMatrix()
◆ CreateKMatrix()
Implements Analysis.
Definition at line 112 of file FreeSurfaceTopAnalysis.cpp.
115 int domaintype,dim,stabilization;
125 topelement = element;
146 IssmDouble* basis = xNew<IssmDouble>(numnodes);
147 IssmDouble* B = xNew<IssmDouble>(dim*numnodes);
148 IssmDouble* Bprime = xNew<IssmDouble>(dim*numnodes);
162 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
171 D_scalar=gauss->
weight*Jdet;
177 GetB(B,topelement,dim,xyz_list,gauss);
178 GetBprime(Bprime,topelement,dim,xyz_list,gauss);
180 D_scalar=dt*gauss->
weight*Jdet;
181 for(
int i=0;i<dim*dim;i++) D[i]=0.;
183 if(dim==2) D[1*dim+1]=D_scalar*vy;
187 Bprime,dim,numnodes,0,
190 if(stabilization==2){
194 D[0] = h/(2.*vel)*vx*vx;
197 vel=sqrt(vx*vx+vy*vy)+1.e-8;
198 D[0*dim+0]=h/(2*vel)*vx*vx;
199 D[1*dim+0]=h/(2*vel)*vy*vx;
200 D[0*dim+1]=h/(2*vel)*vx*vy;
201 D[1*dim+1]=h/(2*vel)*vy*vy;
204 else if(stabilization==1){
214 D[0*dim+0]=h/2.0*fabs(vx);
215 D[1*dim+1]=h/2.0*fabs(vy);
218 if(stabilization==1 || stabilization==2){
219 for(
int i=0;i<dim*dim;i++) D[i]=D_scalar*D[i];
222 Bprime,dim,numnodes,0,
228 xDelete<IssmDouble>(xyz_list);
229 xDelete<IssmDouble>(basis);
230 xDelete<IssmDouble>(B);
231 xDelete<IssmDouble>(Bprime);
232 xDelete<IssmDouble>(D);
◆ CreatePVector()
Implements Analysis.
Definition at line 237 of file FreeSurfaceTopAnalysis.cpp.
249 topelement = element;
270 IssmDouble* basis = xNew<IssmDouble>(numnodes);
281 default:
_error_(
"not implemented");
287 for(
int ig=gauss->
begin();ig<gauss->end();ig++){
297 for(
int i=0;i<numnodes;i++) pe->
values[i]+=Jdet*gauss->
weight*(surface + dt*ms + dt*vz)*basis[i];
301 xDelete<IssmDouble>(xyz_list);
302 xDelete<IssmDouble>(basis);
◆ GetB()
Definition at line 308 of file FreeSurfaceTopAnalysis.cpp.
327 for(
int i=0;i<numnodes;i++){
328 for(
int j=0;j<dim;j++){
329 B[numnodes*j+i] = basis[i];
334 xDelete<IssmDouble>(basis);
◆ GetBprime()
Definition at line 336 of file FreeSurfaceTopAnalysis.cpp.
351 IssmDouble* dbasis=xNew<IssmDouble>(dim*numnodes);
355 for(
int i=0;i<numnodes;i++){
356 for(
int j=0;j<dim;j++){
357 Bprime[numnodes*j+i] = dbasis[j*numnodes+i];
362 xDelete<IssmDouble>(dbasis);
◆ GetSolutionFromInputs()
◆ GradientJ()
void FreeSurfaceTopAnalysis::GradientJ |
( |
Vector< IssmDouble > * |
gradient, |
|
|
Element * |
element, |
|
|
int |
control_type, |
|
|
int |
control_index |
|
) |
| |
|
virtual |
◆ InputUpdateFromSolution()
void FreeSurfaceTopAnalysis::InputUpdateFromSolution |
( |
IssmDouble * |
solution, |
|
|
Element * |
element |
|
) |
| |
|
virtual |
◆ UpdateConstraints()
void FreeSurfaceTopAnalysis::UpdateConstraints |
( |
FemModel * |
femmodel | ) |
|
|
virtual |
The documentation for this class was generated from the following files:
@ FreeSurfaceTopAnalysisEnum
virtual int GetNumberOfNodes(void)=0
void FindParam(bool *pvalue, int paramenum)
int AddObject(Object *object)
@ TimesteppingTimeStepEnum
int TripleMultiply(IssmDouble *a, int nrowa, int ncola, int itrna, IssmDouble *b, int nrowb, int ncolb, int itrnb, IssmDouble *c, int nrowc, int ncolc, int itrnc, IssmDouble *d, int iaddd)
@ MasstransportStabilizationEnum
virtual Input2 * GetInput2(int inputenum)=0
void DeleteMaterials(void)
virtual void NodalFunctions(IssmDouble *basis, Gauss *gauss)=0
ElementVector * NewElementVector(int approximation_enum=NoneApproximationEnum)
void DeleteData(int num,...)
virtual Gauss * NewGauss(void)=0
virtual void InputUpdateFromSolutionOneDof(IssmDouble *solution, int inputenum)=0
const char * EnumToStringx(int enum_in)
void FindConstant(bool *pvalue, const char *constant_name)
void GetVerticesCoordinates(IssmDouble **xyz_list)
void GetB(IssmDouble *B, Element *element, int dim, IssmDouble *xyz_list, Gauss *gauss)
void FetchData(bool *pboolean, const char *data_name)
void GetBprime(IssmDouble *B, Element *element, int dim, IssmDouble *xyz_list, Gauss *gauss)
void CreateNodes(Nodes *nodes, IoModel *iomodel, bool isamr=false)
#define _error_(StreamArgs)
virtual Element * SpawnTopElement(void)=0
virtual int begin(void)=0
Object * GetObjectByOffset(int offset)
virtual IssmDouble CharacteristicLength(void)=0
@ MeshVertexonsurfaceEnum
virtual void JacobianDeterminant(IssmDouble *Jdet, IssmDouble *xyz_list, Gauss *gauss)=0
virtual void GaussPoint(int ig)=0
virtual void Update(Inputs2 *inputs2, int index, IoModel *iomodel, int analysis_counter, int analysis_type, int finite_element)=0
void FetchDataToInput(Inputs2 *inputs2, Elements *elements, const char *vector_name, int input_enum)
virtual void NodalFunctionsDerivatives(IssmDouble *dbasis, IssmDouble *xyz_list, Gauss *gauss)=0
ElementMatrix * NewElementMatrix(int approximation_enum=NoneApproximationEnum)