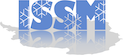 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
10 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
13 #include "../classes.h"
14 #include "../../shared/shared.h"
32 xMemCpy<IssmDouble>(
values,in_values,
M*
N);
39 xDelete<IssmDouble>(
values);
54 _printf_(
" number of time steps: " << this->
N <<
"\n");
55 _printf_(
" number of rows: " << this->
M <<
"\n");
56 for(
int i=0;i<this->
N;i++){
58 for(
int k=0;k<this->
M;k++){
69 _printf_(
" size: " << this->
M <<
" by " << this->
N <<
"\n");
108 output=this->
values[row*this->
N];
111 else if(time>this->timesteps[this->
N-1]){
113 output=this->
values[(row+1)*this->
N-1];
118 for(
int i=0;i<this->
N;i++){
119 if(time==this->timesteps[i]){
121 output = this->
values[row*this->N+i];
126 if(this->timesteps[i]<time && time<this->
timesteps[i+1]){
128 IssmDouble deltat = this->timesteps[i+1]-this->timesteps[i];
131 else output=this->
values[row*this->N+i];
139 if(!found)
_error_(
"did not find time interval on which to interpolate values");
void GetParameterValue(bool *pbool)
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
@ TransientArrayParamEnum
#define MARSHALLING_ARRAY(FIELD, TYPE, SIZE)
const char * EnumToStringx(int enum_in)
IssmDouble alpha(IssmDouble x, IssmDouble y, IssmDouble z, int testid)
#define MARSHALLING(FIELD)
#define _error_(StreamArgs)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)