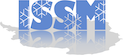 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
8 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
11 #include "../classes.h"
13 #include "../../shared/shared.h"
121 int sid = node->
Sid();
122 this->
value = vector[sid];
129 if(!this->
penalty)
_error_(
"cannot return dof and value for non penalty constraint");
141 if(xIsNan<IssmDouble>(value_out)) gdof = -1;
Declaration of Nodes class.
Object * GetObjectById(int *poffset, int eid)
#define _printf_(StreamArgs)
Declaration of Parameters class.
#define MARSHALLING_ENUM(EN)
void ConstrainNode(Nodes *nodes, Parameters *parameters)
abstract class for Constraint object This class is a place holder for constraints It is derived from ...
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)
const char * EnumToStringx(int enum_in)
#define MARSHALLING(FIELD)
#define _error_(StreamArgs)
void ApplyConstraint(int dof, IssmDouble value)
void ActivatePenaltyMethod(void)
@ BalancethicknessSpcthicknessEnum
void PenaltyDofAndValue(int *dof, IssmDouble *value, Nodes *nodes, Parameters *parameters)
void InputUpdateFromVectorDakota(IssmDouble *vector, Nodes *nodes, int name, int type)
int GetDof(int dofindex, int setenum)