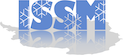 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
10 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
13 #include "../classes.h"
29 xMemCpy<int>(
value,in_value,
M*
N);
51 _printf_(
" matrix size: " << this->
M <<
"x" << this->
N <<
"\n");
52 for(i=0;i<this->
M;i++){
53 for(j=0;j<this->
N;j++){
54 _printf_(
"(" << i <<
"," << j <<
") " << *(this->
value+N*i+j) <<
"\n");
63 _printf_(
" matrix size: " << this->
M <<
"x" << this->
N <<
"\n");
90 output=xNew<int>(
M*
N);
102 xDelete<int>(this->
value);
104 this->
value=xNew<int>(in_M*in_N);
105 xMemCpy<int>(this->
value,intarray,in_M*in_N);
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
void SetValue(bool boolean)
#define MARSHALLING_DYNAMIC(FIELD, TYPE, SIZE)
void GetParameterValue(bool *pbool)
const char * EnumToStringx(int enum_in)
#define MARSHALLING(FIELD)
void Marshall(char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction)