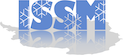 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
8 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
11 #include "../classes.h"
13 #include "../../shared/shared.h"
25 this->
values = xNew<bool>(size_in);
29 xDelete<bool>(this->
values);
38 xMemCpy<bool>(output->
values,this->values,this->size);
81 *pvalue = this->
values[index];
89 this->
values[index] = value;
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
#define MARSHALLING_DYNAMIC(FIELD, TYPE, SIZE)
#define MARSHALLING(FIELD)
void printarray(IssmPDouble *array, int lines, int cols=1)