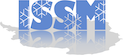 |
Ice Sheet System Model
4.18
Code documentation
|
#include <TransientInput2.h>
|
| TransientInput2 () |
|
| TransientInput2 (int in_enum_type, int nbe, int nbv, IssmDouble *times, int N) |
|
| ~TransientInput2 () |
|
void | AddTimeInput (Input2 *input, IssmDouble time) |
|
void | AddTriaTimeInput (IssmDouble time, int numindices, int *indices, IssmDouble *values_in, int interp_in) |
|
void | AddPentaTimeInput (IssmDouble time, int numindices, int *indices, IssmDouble *values_in, int interp_in) |
|
void | AddTriaTimeInput (int step, int numindices, int *indices, IssmDouble *values_in, int interp_in) |
|
void | AddPentaTimeInput (int step, int numindices, int *indices, IssmDouble *values_in, int interp_in) |
|
Input2 * | copy () |
|
void | Configure (Parameters *params) |
|
void | DeepEcho () |
|
void | Echo () |
|
int | Id () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | ObjectEnum () |
|
void | GetAllTimes (IssmDouble **ptimesteps, int *pnumtimesteps) |
|
TriaInput2 * | GetTriaInput () |
|
TriaInput2 * | GetTriaInput (IssmDouble time) |
|
TriaInput2 * | GetTriaInput (IssmDouble start_time, IssmDouble end_time, int averaging_method) |
|
TriaInput2 * | GetTriaInput (int offset) |
|
PentaInput2 * | GetPentaInput () |
|
PentaInput2 * | GetPentaInput (IssmDouble time) |
|
PentaInput2 * | GetPentaInput (int offset) |
|
PentaInput2 * | GetPentaInput (IssmDouble start_time, IssmDouble end_time, int averaging_method) |
|
Input2 * | GetTimeInput (IssmDouble time) |
|
IssmDouble | GetTimeByOffset (int offset) |
|
int | GetTimeInputOffset (IssmDouble time) |
|
void | SetCurrentTimeInput (IssmDouble time) |
|
void | SetAverageAsCurrentTimeInput (IssmDouble start_time, IssmDouble end_time, int averaging_method) |
|
int | InstanceEnum () |
|
void | ChangeEnum (int newenumtype) |
|
virtual | ~Input2 () |
|
virtual void | GetInputAverage (IssmDouble *pvalue) |
|
virtual IssmDouble | GetInputMax (void) |
|
virtual IssmDouble | GetInputMaxAbs (void) |
|
virtual IssmDouble | GetInputMin (void) |
|
virtual void | GetInputDerivativeValue (IssmDouble *derivativevalues, IssmDouble *xyz_list, Gauss *gauss) |
|
virtual void | GetInputValue (IssmDouble *pvalue, Gauss *gauss) |
|
virtual int | GetInputInterpolationType () |
|
virtual SegInput2 * | GetSegInput () |
|
virtual void | AXPY (Input2 *xinput, IssmDouble scalar) |
|
virtual void | PointWiseMult (Input2 *xinput) |
|
virtual void | Pow (IssmDouble scale_factor) |
|
virtual void | Scale (IssmDouble scale_factor) |
|
virtual int | GetResultArraySize (void) |
|
virtual int | GetResultInterpolation (void) |
|
virtual int | GetResultNumberOfNodes (void) |
|
virtual | ~Object () |
|
Definition at line 13 of file TransientInput2.h.
◆ TransientInput2() [1/2]
TransientInput2::TransientInput2 |
( |
| ) |
|
◆ TransientInput2() [2/2]
TransientInput2::TransientInput2 |
( |
int |
in_enum_type, |
|
|
int |
nbe, |
|
|
int |
nbv, |
|
|
IssmDouble * |
times, |
|
|
int |
N |
|
) |
| |
◆ ~TransientInput2()
TransientInput2::~TransientInput2 |
( |
| ) |
|
◆ AddTimeInput()
◆ AddTriaTimeInput() [1/2]
void TransientInput2::AddTriaTimeInput |
( |
IssmDouble |
time, |
|
|
int |
numindices, |
|
|
int * |
indices, |
|
|
IssmDouble * |
values_in, |
|
|
int |
interp_in |
|
) |
| |
◆ AddPentaTimeInput() [1/2]
void TransientInput2::AddPentaTimeInput |
( |
IssmDouble |
time, |
|
|
int |
numindices, |
|
|
int * |
indices, |
|
|
IssmDouble * |
values_in, |
|
|
int |
interp_in |
|
) |
| |
◆ AddTriaTimeInput() [2/2]
void TransientInput2::AddTriaTimeInput |
( |
int |
step, |
|
|
int |
numindices, |
|
|
int * |
indices, |
|
|
IssmDouble * |
values_in, |
|
|
int |
interp_in |
|
) |
| |
◆ AddPentaTimeInput() [2/2]
void TransientInput2::AddPentaTimeInput |
( |
int |
step, |
|
|
int |
numindices, |
|
|
int * |
indices, |
|
|
IssmDouble * |
values_in, |
|
|
int |
interp_in |
|
) |
| |
◆ copy()
Input2 * TransientInput2::copy |
( |
void |
| ) |
|
|
virtual |
◆ Configure()
void TransientInput2::Configure |
( |
Parameters * |
params | ) |
|
|
virtual |
◆ DeepEcho()
void TransientInput2::DeepEcho |
( |
void |
| ) |
|
|
virtual |
◆ Echo()
void TransientInput2::Echo |
( |
void |
| ) |
|
|
virtual |
◆ Id()
int TransientInput2::Id |
( |
void |
| ) |
|
|
virtual |
◆ Marshall()
void TransientInput2::Marshall |
( |
char ** |
pmarshalled_data, |
|
|
int * |
pmarshalled_data_size, |
|
|
int |
marshall_direction |
|
) |
| |
|
virtual |
◆ ObjectEnum()
int TransientInput2::ObjectEnum |
( |
void |
| ) |
|
|
virtual |
◆ GetAllTimes()
void TransientInput2::GetAllTimes |
( |
IssmDouble ** |
ptimesteps, |
|
|
int * |
pnumtimesteps |
|
) |
| |
◆ GetTriaInput() [1/4]
◆ GetTriaInput() [2/4]
◆ GetTriaInput() [3/4]
◆ GetTriaInput() [4/4]
TriaInput2 * TransientInput2::GetTriaInput |
( |
int |
offset | ) |
|
Definition at line 306 of file TransientInput2.cpp.
310 _error_(
"Cannot return input for offset "<<offset);
317 return xDynamicCast<TriaInput2*>(input);
◆ GetPentaInput() [1/4]
◆ GetPentaInput() [2/4]
◆ GetPentaInput() [3/4]
PentaInput2 * TransientInput2::GetPentaInput |
( |
int |
offset | ) |
|
Definition at line 342 of file TransientInput2.cpp.
347 _error_(
"Cannot return input for offset "<<offset);
353 return xDynamicCast<PentaInput2*>(input);
◆ GetPentaInput() [4/4]
◆ GetTimeInput()
◆ GetTimeByOffset()
IssmDouble TransientInput2::GetTimeByOffset |
( |
int |
offset | ) |
|
◆ GetTimeInputOffset()
int TransientInput2::GetTimeInputOffset |
( |
IssmDouble |
time | ) |
|
◆ SetCurrentTimeInput()
void TransientInput2::SetCurrentTimeInput |
( |
IssmDouble |
time | ) |
|
Definition at line 372 of file TransientInput2.cpp.
381 _error_(
"Input not found (is TransientInput sorted ?)");
398 else if(offset==(this->
numtimesteps-1) || !linear_interp){
404 if(this->
current_step==reCast<IssmDouble>(offset))
return;
418 IssmDouble this_step = reCast<IssmDouble>(offset) + (time - this->timesteps[offset])/deltat;
427 IssmDouble alpha2=(time-this->timesteps[offset])/deltat;
◆ SetAverageAsCurrentTimeInput()
void TransientInput2::SetAverageAsCurrentTimeInput |
( |
IssmDouble |
start_time, |
|
|
IssmDouble |
end_time, |
|
|
int |
averaging_method |
|
) |
| |
Definition at line 439 of file TransientInput2.cpp.
444 int found,start_offset,end_offset,input_offset;
448 if(!found)
_error_(
"Input not found (is TransientInput sorted ?)");
450 if(!found)
_error_(
"Input not found (is TransientInput sorted ?)");
452 if(start_offset==-1){
453 timespan=this->
timesteps[end_offset]-start_time;
458 mid_step=reCast<IssmDouble>(start_offset)+0.5*timespan;
466 int offset=start_offset;
467 while(offset < end_offset){
468 if(offset==start_offset){
471 if(offset==end_offset-1){
472 dt=end_time-start_time;
476 else if(offset==end_offset-1){
486 switch(averaging_method){
488 if(offset==start_offset){
497 if(offset==start_offset){
502 stepinput->
Scale(dt);
507 if(offset==start_offset){
517 _error_(
"averaging method is not recognised");
525 switch(averaging_method){
536 _error_(
"averaging method is not recognised");
◆ numberofelements_local
int TransientInput2::numberofelements_local |
|
private |
◆ numberofvertices_local
int TransientInput2::numberofvertices_local |
|
private |
◆ enum_type
int TransientInput2::enum_type |
◆ numtimesteps
int TransientInput2::numtimesteps |
◆ inputs
Input2** TransientInput2::inputs |
◆ timesteps
◆ parameters
◆ current_step
◆ current_input
Input2* TransientInput2::current_input |
The documentation for this class was generated from the following files:
#define _printf_(StreamArgs)
#define MARSHALLING_ENUM(EN)
#define MARSHALLING_DYNAMIC(FIELD, TYPE, SIZE)
const char * EnumToStringx(int enum_in)
#define MARSHALLING(FIELD)
virtual int ObjectEnum()=0
@ TimesteppingInterpForcingsEnum
#define _error_(StreamArgs)
void FindParam(bool *pinteger, int enum_type)
T * xMemCpy(T *dest, const T *src, unsigned int size)
int binary_search(int *poffset, int target, int *sorted_integers, int num_integers)