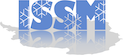 |
Ice Sheet System Model
4.18
Code documentation
|
Declaration of Observations class.
More...
#include <Observations.h>
|
| Observations () |
|
| Observations (IssmDouble *observations_list, IssmDouble *x, IssmDouble *y, int n, Options *options) |
|
| ~Observations () |
|
void | InitCovertree (IssmDouble *observations_list, IssmDouble *x, IssmDouble *y, int n, Options *options) |
|
void | InitQuadtree (IssmDouble *observations_list, IssmDouble *x, IssmDouble *y, int n, Options *options) |
|
void | ClosestObservation (IssmDouble *px, IssmDouble *py, IssmDouble *pobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius) |
|
void | ClosestObservationCovertree (IssmDouble *px, IssmDouble *py, IssmDouble *pobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius) |
|
void | ClosestObservationQuadtree (IssmDouble *px, IssmDouble *py, IssmDouble *pobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius) |
|
void | Distances (IssmPDouble *distances, IssmPDouble *x, IssmPDouble *y, int n, IssmPDouble radius) |
|
void | InterpolationIDW (IssmDouble *pprediction, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius, int mindata, int maxdata, IssmDouble power) |
|
void | InterpolationV4 (IssmDouble *pprediction, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius, int mindata, int maxdata) |
|
void | InterpolationKriging (IssmDouble *pprediction, IssmDouble *perror, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius, int mindata, int maxdata, Variogram *variogram) |
|
void | InterpolationNearestNeighbor (IssmDouble *pprediction, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius) |
|
void | ObservationList (IssmDouble **px, IssmDouble **py, IssmDouble **pobs, int *pnobs) |
|
void | ObservationList (IssmDouble **px, IssmDouble **py, IssmDouble **pobs, int *pnobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius, int maxdata) |
|
void | ObservationListCovertree (IssmDouble **px, IssmDouble **py, IssmDouble **pobs, int *pnobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius, int maxdata) |
|
void | ObservationListQuadtree (IssmDouble **px, IssmDouble **py, IssmDouble **pobs, int *pnobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius, int maxdata) |
|
void | QuadtreeColoring (IssmDouble *A, IssmDouble *x, IssmDouble *y, int n) |
|
void | Variomap (IssmDouble *gamma, IssmDouble *x, int n) |
|
| DataSet () |
|
| DataSet (int enum_type) |
|
| ~DataSet () |
|
void | Marshall (char **pmarshalled_data, int *pmarshalled_data_size, int marshall_direction) |
|
int | GetEnum () |
|
int | GetEnum (int offset) |
|
void | Echo () |
|
void | DeepEcho () |
|
int | AddObject (Object *object) |
|
int | DeleteObject (int id) |
|
int | Size () |
|
void | clear () |
|
Object * | GetObjectByOffset (int offset) |
|
Object * | GetObjectById (int *poffset, int eid) |
|
void | Presort () |
|
void | Sort () |
|
DataSet * | Copy (void) |
|
int | DeleteObject (Object *object) |
|
Declaration of Observations class.
Declaration of Observations class. Observations are vector lists (Containers) of Observation objects.
Definition at line 16 of file Observations.h.
◆ Observations() [1/2]
Observations::Observations |
( |
| ) |
|
◆ Observations() [2/2]
Definition at line 40 of file Observations.cpp.
43 if(n<=0)
_error_(
"No observation found");
47 options->
Get(&dtree,
"treetype",1.);
59 _error_(
"Tree type "<<this->
treetype<<
" not supported yet (1: quadtree, 2: covertree)");
◆ ~Observations()
Observations::~Observations |
( |
| ) |
|
◆ InitCovertree()
Definition at line 79 of file Observations.cpp.
88 options->
Get(&mintrimming,
"mintrimming",-1.e+21);
89 options->
Get(&maxtrimming,
"maxtrimming",+1.e+21);
90 options->
Get(&minspacing,
"minspacing",0.01);
91 if(minspacing<=0)
_error_(
"minspacing must > 0");
102 for(
int i=1;i<n;i++){
103 if(x[i]<xmin) xmin=x[i];
104 if(x[i]>xmax) xmax=x[i];
105 if(y[i]<ymin) ymin=y[i];
106 if(y[i]>ymax) ymax=y[i];
108 IssmPDouble maxDist = sqrt(pow(xmax-xmin,2)+pow(ymax-ymin,2));
110 int maxdepth = ceilf(log(maxDist)/log(base));
112 _printf0_(
"Generating covertree with a maximum depth " << maxdepth <<
"... ");
115 for(
int i=0;i<n;i++){
118 if(observations_list[i]>maxtrimming)
continue;
119 if(observations_list[i]<mintrimming)
continue;
127 if(oldobs.
distance(newobs)<minspacing)
continue;
◆ InitQuadtree()
Definition at line 135 of file Observations.cpp.
138 int i,maxdepth,level,counter,index;
141 IssmPDouble offset,minlength,minspacing,mintrimming,maxtrimming;
148 xmin=x[0]; ymin=y[0];
149 xmax=x[0]; ymax=y[0];
151 xmin=
min(xmin,x[i]); ymin=
min(ymin,y[i]);
152 xmax=
max(xmax,x[i]); ymax=
max(ymax,y[i]);
154 offset=0.05*(xmax-xmin); xmin-=offset; xmax+=offset;
155 offset=0.05*(ymax-ymin); ymin-=offset; ymax+=offset;
158 options->
Get(&mintrimming,
"mintrimming",-1.e+21);
159 options->
Get(&maxtrimming,
"maxtrimming",+1.e+21);
160 options->
Get(&minspacing,
"minspacing",0.01);
161 if(minspacing<=0)
_error_(
"minspacing must > 0");
165 options->
Get(&minlength,
"boxlength");
166 if(minlength<=0)
_error_(
"boxlength should be a positive number");
167 maxdepth=reCast<int,IssmPDouble>(log(
max(xmax-xmin,ymax-ymin)/minlength +1)/log(2.0));
175 _printf0_(
"Generating quadtree with a maximum box size " << minlength <<
" (depth=" << maxdepth <<
")... ");
183 if(observations_list[i]>maxtrimming)
continue;
184 if(observations_list[i]<mintrimming)
continue;
190 if(pow(observation->
x-x[i],2)+pow(observation->
y-y[i],2) < minspacing)
continue;
195 if((
int)level <= maxdepth){
196 observation =
new Observation(x[i],y[i],xi,yi,counter++,observations_list[i]);
206 _printf0_(
"Initial number of observations: " << n <<
"\n");
◆ ClosestObservation()
◆ ClosestObservationCovertree()
◆ ClosestObservationQuadtree()
Definition at line 248 of file Observations.cpp.
266 hmin = sqrt((observation->
x-x_interp)*(observation->
x-x_interp) + (observation->
y-y_interp)*(observation->
y-y_interp));
267 if(hmin<radius) radius=hmin;
272 for (i=0;i<nobs;i++){
274 h2 = (observation->
x-x_interp)*(observation->
x-x_interp) + (observation->
y-y_interp)*(observation->
y-y_interp);
288 if(nobs || hmin==radius){
290 *px = observation->
x;
291 *py = observation->
y;
292 *pobs = observation->
value;
299 xDelete<int>(indices);
◆ Distances()
Definition at line 302 of file Observations.cpp.
306 for(
int i=0;i<n;i++){
312 distances[i]=sqrt( (x[i]-xi)*(x[i]-xi) + (y[i]-yi)*(y[i]-yi) );
◆ InterpolationIDW()
Definition at line 481 of file Observations.cpp.
497 this->
ObservationList(&x,&y,&obs,&n_obs,x_interp,y_interp,radius,maxdata);
506 for(i=0;i<n_obs;i++){
507 h = sqrt( (x[i]-x_interp)*(x[i]-x_interp) + (y[i]-y_interp)*(y[i]-y_interp));
513 weight = 1./pow(h,power);
514 numerator += weight*obs[i];
515 denominator += weight;
517 prediction = numerator/denominator;
521 *pprediction = prediction;
522 xDelete<IssmPDouble>(x);
523 xDelete<IssmPDouble>(y);
524 xDelete<IssmPDouble>(obs);
◆ InterpolationV4()
Definition at line 610 of file Observations.cpp.
631 this->
ObservationList(&x,&y,&obs,&n_obs,x_interp,y_interp,radius,maxdata);
634 if(n_obs<mindata || n_obs<2){
640 Green = xNew<IssmPDouble>(n_obs*n_obs);
641 g = xNew<IssmPDouble>(n_obs);
644 for(i=0;i<n_obs;i++){
645 h = sqrt( (x[i]-x_interp)*(x[i]-x_interp) + (y[i]-y_interp)*(y[i]-y_interp) );
647 g[i] = h*h*(log(h)-1.);
655 for(i=0;i<n_obs;i++){
657 h = sqrt( (x[i]-x[j])*(x[i]-x[j]) + (y[i]-y[j])*(y[i]-y[j]) );
659 Green[j*n_obs+i] = h*h*(log(h)-1.);
662 Green[j*n_obs+i] = 0.;
664 Green[i*n_obs+j] = Green[j*n_obs+i];
667 Green[i*n_obs+i] = 0.;
679 for(i=0;i<n_obs;i++) prediction += weights[i]*g[i];
684 *pprediction = prediction;
685 xDelete<IssmPDouble>(x);
686 xDelete<IssmPDouble>(y);
687 xDelete<IssmPDouble>(obs);
688 xDelete<IssmPDouble>(Green);
689 xDelete<IssmPDouble>(g);
690 xDelete<IssmPDouble>(weights);
◆ InterpolationKriging()
Definition at line 526 of file Observations.cpp.
541 this->
ObservationList(&x,&y,&obs,&n_obs,x_interp,y_interp,radius,maxdata);
545 *pprediction = -999.0;
551 IssmPDouble* A = xNew<IssmPDouble>((n_obs+1)*(n_obs+1));
556 for(i=0;i<n_obs;i++){
559 A[i*(n_obs+1)+j] = variogram->
Covariance(x[i]-x[j],y[i]-y[j]);
560 A[j*(n_obs+1)+i] = A[i*(n_obs+1)+j];
562 A[i*(n_obs+1)+n_obs] = unbias;
565 for(i=0;i<n_obs;i++) A[n_obs*(n_obs+1)+i]=unbias;
567 A[n_obs*(n_obs+1)+n_obs] = 0.;
570 for(i=0;i<n_obs;i++) B[i] = variogram->
Covariance(x[i]-x_interp,y[i]-y_interp);
583 for(i=0;i<n_obs;i++) prediction += Lambda[i]*obs[i];
586 error = variogram->
Covariance(0.,0.)*(1. - Lambda[n_obs]);;
587 for(i=0;i<n_obs;i++) error += -Lambda[i]*B[i];
590 *pprediction = prediction;
592 xDelete<IssmPDouble>(x);
593 xDelete<IssmPDouble>(y);
594 xDelete<IssmPDouble>(obs);
595 xDelete<IssmPDouble>(A);
596 xDelete<IssmPDouble>(B);
597 xDelete<IssmPDouble>(Lambda);
◆ InterpolationNearestNeighbor()
◆ ObservationList() [1/2]
Definition at line 316 of file Observations.cpp.
328 x = xNew<IssmPDouble>(nobs);
329 y = xNew<IssmPDouble>(nobs);
330 obs = xNew<IssmPDouble>(nobs);
331 for(
int i=0;i<this->
Size();i++){
◆ ObservationList() [2/2]
◆ ObservationListCovertree()
Definition at line 356 of file Observations.cpp.
362 std::vector<Observation> kNN;
370 vector<Observation>::iterator it;
372 for (it = kNN.begin(); it != kNN.end(); ++it) {
375 if ((*it).distance(observation) > radius) {
379 kNN.erase(it, kNN.end());
383 x =
new double[kNN.size()];
384 y =
new double[kNN.size()];
385 obs =
new double[kNN.size()];
389 for(it = kNN.begin(); it != kNN.end(); ++it) {
390 (*it).WriteXYObs((*it), &x[i], &y[i], &obs[i]);
◆ ObservationListQuadtree()
Definition at line 399 of file Observations.cpp.
403 int nobs,tempnobs,i,j,k,n,counter;
406 int *tempindices = NULL;
417 radius2 = radius*radius;
422 indices = xNew<int>(tempnobs);
423 dists = xNew<IssmPDouble>(tempnobs);
426 for(i=0;i<tempnobs;i++){
428 h2 = (observation->
x-x_interp)*(observation->
x-x_interp) + (observation->
y-y_interp)*(observation->
y-y_interp);
430 if(nobs==maxdata && h2>radius2)
continue;
432 indices[nobs] = tempindices[i];
436 if(nobs==1)
continue;
444 for(
int jj=k;jj<n;jj++){
446 dists[j+1] = dists[j];
447 indices[j+1] = indices[j];
451 indices[k] = tempindices[i];
458 xDelete<IssmPDouble>(dists);
459 xDelete<int>(tempindices);
463 x = xNew<IssmPDouble>(nobs);
464 y = xNew<IssmPDouble>(nobs);
465 obs = xNew<IssmPDouble>(nobs);
475 xDelete<int>(indices);
◆ QuadtreeColoring()
◆ Variomap()
Definition at line 704 of file Observations.cpp.
713 for(j=0;j<n;j++) counter[j] = 0.0;
714 for(j=0;j<n;j++) gamma[j] = 0.0;
716 for(i=0;i<this->
Size();i++){
719 for(j=i+1;j<this->
Size();j++){
722 distance=sqrt(pow(observation1->
x - observation2->
x,2) + pow(observation1->
y - observation2->
y,2));
723 if(distance>x[n-1])
continue;
725 int index = int(distance/(x[1]-x[0]));
726 if(index>n-1) index = n-1;
727 if(index<0) index = 0;
729 gamma[index] += 1./2.*pow(observation1->
value - observation2->
value,2);
730 counter[index] += 1.;
737 if(counter[k]) gamma[k] = gamma[k]/counter[k];
741 xDelete<IssmPDouble>(counter);
◆ treetype
int Observations::treetype |
|
private |
◆ quadtree
◆ covertree
The documentation for this class was generated from the following files:
double distance(const Observation &ob) const
void insert(const Observation &newObservation)
#define _printf0_(StreamArgs)
int AddObject(Object *object)
#define _printf_(StreamArgs)
void QuadtreeDepth(int *A, int xi, int yi)
virtual double Covariance(double deltax, double deltay)=0
CoverTreeNode * getRoot() const
void ClosestObservation(IssmDouble *px, IssmDouble *py, IssmDouble *pobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius)
void ClosestObservationQuadtree(IssmDouble *px, IssmDouble *py, IssmDouble *pobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius)
void WriteXYObs(const Observation &ob, double *px, double *py, double *pobs)
void ObservationListCovertree(IssmDouble **px, IssmDouble **py, IssmDouble **pobs, int *pnobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius, int maxdata)
void InitCovertree(IssmDouble *observations_list, IssmDouble *x, IssmDouble *y, int n, Options *options)
void AddAndAverage(double x, double y, double value)
void ClosestObservationCovertree(IssmDouble *px, IssmDouble *py, IssmDouble *pobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius)
void InitQuadtree(IssmDouble *observations_list, IssmDouble *x, IssmDouble *y, int n, Options *options)
void Get(OptionType *pvalue, const char *name)
void ObservationList(IssmDouble **px, IssmDouble **py, IssmDouble **pobs, int *pnobs)
Option * GetOption(const char *name)
void ClosestObs(int *pindex, double x, double y)
void RangeSearch(int **pindices, int *pnobs, double x, double y, double range)
#define _error_(StreamArgs)
void Add(Observation *observation)
Object * GetObjectByOffset(int offset)
IssmDouble min(IssmDouble a, IssmDouble b)
void IntergerCoordinates(int *xi, int *yi, double x, double y)
void QuadtreeDepth2(int *A, int xi, int yi)
std::vector< Observation > kNearestNeighbors(const Observation &p, const unsigned int &k) const
void DenseGslSolve(IssmPDouble **pX, IssmPDouble *A, IssmPDouble *B, int n)
IssmDouble max(IssmDouble a, IssmDouble b)
void ObservationListQuadtree(IssmDouble **px, IssmDouble **py, IssmDouble **pobs, int *pnobs, IssmDouble x_interp, IssmDouble y_interp, IssmDouble radius, int maxdata)