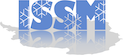 |
Ice Sheet System Model
4.18
Code documentation
|
#include <Friction.h>
|
| Friction () |
|
| Friction (Element *element_in, int dim_in) |
|
| ~Friction () |
|
void | Echo (void) |
|
void | GetAlphaComplement (IssmDouble *alpha_complement, Gauss *gauss) |
|
void | GetAlphaHydroComplement (IssmDouble *alpha_complement, Gauss *gauss) |
|
void | GetAlphaTempComplement (IssmDouble *alpha_complement, Gauss *gauss) |
|
void | GetAlphaViscousComplement (IssmDouble *alpha_complement, Gauss *gauss) |
|
void | GetAlpha2 (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Coulomb (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Hydro (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Josh (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Shakti (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Temp (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Viscous (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2WaterLayer (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Weertman (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2WeertmanTemp (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2PISM (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Schoof (IssmDouble *palpha2, Gauss *gauss) |
|
void | GetAlpha2Tsai (IssmDouble *palpha2, Gauss *gauss) |
|
IssmDouble | EffectivePressure (Gauss *gauss) |
|
IssmDouble | VelMag (Gauss *gauss) |
|
Definition at line 13 of file Friction.h.
◆ Friction() [1/2]
◆ Friction() [2/2]
Friction::Friction |
( |
Element * |
element_in, |
|
|
int |
dim_in |
|
) |
| |
◆ ~Friction()
◆ Echo()
void Friction::Echo |
( |
void |
| ) |
|
◆ GetAlphaComplement()
void Friction::GetAlphaComplement |
( |
IssmDouble * |
alpha_complement, |
|
|
Gauss * |
gauss |
|
) |
| |
Definition at line 42 of file Friction.cpp.
60 _assert_(!xIsNan<IssmDouble>(*palpha_complement));
61 _assert_(!xIsInf<IssmDouble>(*palpha_complement));
◆ GetAlphaHydroComplement()
void Friction::GetAlphaHydroComplement |
( |
IssmDouble * |
alpha_complement, |
|
|
Gauss * |
gauss |
|
) |
| |
Definition at line 64 of file Friction.cpp.
89 alpha=(pow(q_exp-1,q_exp-1))/pow(q_exp,q_exp);
91 Chi = vmag/(pow(C_param,n)*pow(Neff,n)*As);
92 Gamma = (Chi/(1.+
alpha*pow(Chi,q_exp)));
94 if(vmag==0.) alpha_complement=0.;
95 else if(Neff==0.) alpha_complement=0.;
96 else alpha_complement=-(C_param*Neff/(n*vmag)) *
97 pow(Gamma,((1.-n)/n)) *
98 (Gamma/As - (
alpha*q_exp*pow(Chi,q_exp-1.)* Gamma * Gamma/As));
101 *palpha_complement=alpha_complement;
◆ GetAlphaTempComplement()
void Friction::GetAlphaTempComplement |
( |
IssmDouble * |
alpha_complement, |
|
|
Gauss * |
gauss |
|
) |
| |
Definition at line 104 of file Friction.cpp.
126 alpha_complement = alpha_complement/ (exp((T-Tpmp)/gamma)+1e-3);
129 *palpha_complement=alpha_complement;
◆ GetAlphaViscousComplement()
void Friction::GetAlphaViscousComplement |
( |
IssmDouble * |
alpha_complement, |
|
|
Gauss * |
gauss |
|
) |
| |
Definition at line 131 of file Friction.cpp.
157 if(vmag==0. && (s-1.)<0.) alpha_complement=0.;
158 else alpha_complement=pow(Neff,r)*pow(vmag,(s-1));
161 *palpha_complement=alpha_complement;
◆ GetAlpha2()
◆ GetAlpha2Coulomb()
Definition at line 213 of file Friction.cpp.
221 IssmDouble thickness,base,floatation_thickness,sealevel;
222 IssmDouble drag_coefficient,drag_coefficient_coulomb;
246 if(vmag==0. && (s-1.)<0.){
250 alpha2=drag_coefficient*drag_coefficient*pow(Neff,r)*pow(vmag,(s-1.));
253 floatation_thickness=0;
254 if(base<0) floatation_thickness=-(rho_water/rho_ice)*base;
259 alpha2_coulomb=drag_coefficient_coulomb*drag_coefficient_coulomb*rho_ice*gravity*
max(0.,thickness-floatation_thickness)/vmag;
262 if(alpha2_coulomb<alpha2) alpha2=alpha2_coulomb;
◆ GetAlpha2Hydro()
Definition at line 267 of file Friction.cpp.
300 alpha=(pow(q_exp-1,q_exp-1))/pow(q_exp,q_exp);
302 Chi=vmag/(pow(C_param,n)*pow(Neff,n)*As);
303 Gamma=(Chi/(1. +
alpha * pow(Chi,q_exp)));
305 if(vmag==0.) alpha2=0.;
306 else if (Neff==0) alpha2=0.0;
307 else alpha2=Neff * C_param * pow(Gamma,1./n) * pow(vmag,-1);
◆ GetAlpha2Josh()
Definition at line 372 of file Friction.cpp.
381 IssmDouble T,Tpmp,deltaT,deltaTref,pressure,diff,drag_coefficient;
382 IssmDouble alpha2,time,gamma,ref,alp_new,alphascaled;
407 ref = exp(deltaTref/gamma);
408 alp_new = ref/exp(deltaT/gamma);
410 alphascaled = sqrt(alp_new)*drag_coefficient;
411 if (alphascaled > 300) alp_new = (300/drag_coefficient)*(300/drag_coefficient);
413 alp_new=alp_new*alpha2;
◆ GetAlpha2Shakti()
Definition at line 312 of file Friction.cpp.
334 pressure_ice = rho_ice*gravity*thickness;
335 pressure_water = rho_water*gravity*(head-base+sealevel);
336 Neff=pressure_ice-pressure_water;
339 alpha2=drag_coefficient*drag_coefficient*Neff;
◆ GetAlpha2Temp()
Definition at line 345 of file Friction.cpp.
367 alpha2 = alpha2 / (exp((T-Tpmp)/gamma)+1e-3);
◆ GetAlpha2Viscous()
Definition at line 418 of file Friction.cpp.
443 if(vmag==0. && (s-1.)<0.) alpha2=0.;
444 else alpha2=drag_coefficient*drag_coefficient*pow(Neff,r)*pow(vmag,(s-1.));
◆ GetAlpha2WaterLayer()
Definition at line 449 of file Friction.cpp.
481 if(water_layer==0) Neff=gravity*rho_ice*thickness+gravity*rho_water*(base-sealevel);
482 else if(water_layer>0) Neff=gravity*rho_ice*thickness*F;
483 else _error_(
"negative water layer thickness");
489 if(vmag==0. && (s-1.)<0.) alpha2=0.;
490 else alpha2=drag_coefficient*drag_coefficient*pow(Neff,r)*pow(vmag,(s-1.));
◆ GetAlpha2Weertman()
Definition at line 495 of file Friction.cpp.
511 if(vmag==0. && (1./m-1.)<0.) alpha2=0.;
512 else alpha2=pow(C,-1./m)*pow(vmag,(1./m-1.));
◆ GetAlpha2WeertmanTemp()
void Friction::GetAlpha2WeertmanTemp |
( |
IssmDouble * |
palpha2, |
|
|
Gauss * |
gauss |
|
) |
| |
Definition at line 517 of file Friction.cpp.
539 alpha2 = alpha2 / exp((T-Tpmp)/gamma);
◆ GetAlpha2PISM()
Definition at line 544 of file Friction.cpp.
571 P0 = gravity*rho_ice*thickness;
585 N = delta*P0*pow(10.,(e0/Cc)*(1.-W/Wmax));
605 IssmDouble alpha2 = tau_c/(pow(ub+1.e-10,1.-q)*pow(u0,q));
◆ GetAlpha2Schoof()
Definition at line 610 of file Friction.cpp.
637 alpha2= (C*pow(ub,m-1.)) / pow(1.+ pow(C/(Cmax*N),1./m)*ub,m);
◆ GetAlpha2Tsai()
Definition at line 643 of file Friction.cpp.
670 if(alpha2>f*N) alpha2 = f*N;
◆ EffectivePressure()
Definition at line 679 of file Friction.cpp.
693 switch(coupled_flag){
701 p_ice = gravity*rho_ice*thickness;
702 p_water = rho_water*gravity*(sealevel-base);
703 Neff = p_ice - p_water;
710 p_ice = gravity*rho_ice*thickness;
712 Neff = p_ice - p_water;
722 p_ice = gravity*rho_ice*thickness;
723 p_water =
max(0.,rho_water*gravity*(sealevel-base));
724 Neff = p_ice - p_water;
732 p_ice = gravity*rho_ice*thickness;
740 p_ice = gravity*rho_ice*thickness;
748 if(Neff<Neff_limit*p_ice) Neff=Neff_limit*p_ice;
◆ VelMag()
Definition at line 754 of file Friction.cpp.
769 vmag=sqrt(vx*vx+vy*vy);
775 vmag=sqrt(vx*vx+vy*vy+vz*vz);
◆ element
◆ dim
◆ law
The documentation for this class was generated from the following files:
@ FrictionCoefficientEnum
void GetAlphaTempComplement(IssmDouble *alpha_complement, Gauss *gauss)
IssmDouble VelMag(Gauss *gauss)
void GetAlphaViscousComplement(IssmDouble *alpha_complement, Gauss *gauss)
@ FrictionEffectivePressureEnum
void FindParam(bool *pvalue, int paramenum)
#define _printf_(StreamArgs)
@ MaterialsRhoFreshwaterEnum
void GetAlpha2Shakti(IssmDouble *palpha2, Gauss *gauss)
void GetAlphaHydroComplement(IssmDouble *alpha_complement, Gauss *gauss)
void GetAlpha2WaterLayer(IssmDouble *palpha2, Gauss *gauss)
void GetAlpha2PISM(IssmDouble *palpha2, Gauss *gauss)
void GetAlpha2Temp(IssmDouble *palpha2, Gauss *gauss)
@ FrictionThresholdSpeedEnum
void GetAlpha2Weertman(IssmDouble *palpha2, Gauss *gauss)
@ FrictionPseudoplasticityExponentEnum
void GetAlpha2Josh(IssmDouble *palpha2, Gauss *gauss)
@ FrictionPressureAdjustedTemperatureEnum
void GetAlpha2Coulomb(IssmDouble *palpha2, Gauss *gauss)
IssmDouble alpha(IssmDouble x, IssmDouble y, IssmDouble z, int testid)
@ MaterialsRhoSeawaterEnum
IssmDouble TMeltingPoint(IssmDouble pressure)
@ FrictionSedimentCompressibilityCoefficientEnum
void GetAlpha2Hydro(IssmDouble *palpha2, Gauss *gauss)
#define _error_(StreamArgs)
@ HydrologyWatercolumnMaxEnum
@ FrictionEffectivePressureLimitEnum
@ FrictionTillFrictionAngleEnum
void GetInputValue(bool *pvalue, int enum_type)
void FindParam(bool *pinteger, int enum_type)
void GetAlpha2Tsai(IssmDouble *palpha2, Gauss *gauss)
@ FrictionCoefficientcoulombEnum
void GetAlpha2WeertmanTemp(IssmDouble *palpha2, Gauss *gauss)
IssmDouble max(IssmDouble a, IssmDouble b)
void GetAlpha2Schoof(IssmDouble *palpha2, Gauss *gauss)
void GetAlpha2Viscous(IssmDouble *palpha2, Gauss *gauss)
IssmDouble EffectivePressure(Gauss *gauss)