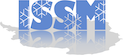 |
Ice Sheet System Model
4.18
Code documentation
|
Go to the documentation of this file.
10 #error "Cannot compile with HAVE_CONFIG_H symbol! run configure first!"
15 #include "../shared/shared.h"
23 memcpy(
altmode,
"clampToGround",(strlen(
"clampToGround")+1)*
sizeof(
char));
70 if(flag)
_printf0_(indent <<
"KML_LinearRing:\n");
73 if(flag)
_printf0_(indent <<
" extrude: " << (
extrude ?
"true" :
"false") <<
"\n");
87 fprintf(filout,
"%s<LinearRing",indent);
89 fprintf(filout,
">\n");
94 fprintf(filout,
"%s <extrude>%d</extrude>\n",indent,(
extrude ? 1 : 0));
95 fprintf(filout,
"%s <tessellate>%d</tessellate>\n",indent,(
tessellate ? 1 : 0));
96 fprintf(filout,
"%s <altitudeMode>%s</altitudeMode>\n",indent,
altmode);
97 fprintf(filout,
"%s <coordinates>\n",indent);
102 fprintf(filout,
"%s %0.16g,%0.16g,%0.16g\n",indent,
coords[3*i+0],
coords[3*i+1],
coords[3*i+2]);
104 fprintf(filout,
"%s </coordinates>\n",indent);
105 fprintf(filout,
"%s</LinearRing>\n",indent);
123 if (!strncmp(kstri,
"</LinearRing",12)){
124 xDelete<char>(kstri);
127 else if (!strncmp(kstri,
"</",2))
128 {
_error_(
"KML_LinearRing::Read -- Unexpected closing tag " << kstri <<
".\n");}
129 else if (strncmp(kstri,
"<",1))
130 {
_error_(
"KML_LinearRing::Read -- Unexpected field \"" << kstri <<
"\".\n");}
132 else if (!strcmp(kstri,
"<extrude>"))
134 else if (!strcmp(kstri,
"<tessellate>"))
136 else if (!strcmp(kstri,
"<altitudeMode>"))
138 else if (!strcmp(kstri,
"<coordinates>"))
140 else if (!strncmp(kstri,
"<",1))
143 xDelete<char>(kstri);
148 for(ncom=ncom; ncom>0; ncom--)
149 xDelete<char>(pcom[ncom-1]);
150 xDelete<char*>(pcom);
158 double *lat,*lon,*x,*y;
163 lat=xNew<IssmPDouble>(
ncoord);
164 lon=xNew<IssmPDouble>(
ncoord);
165 for (i=0; i<
ncoord; i++) {
172 x =xNew<IssmPDouble>(
ncoord);
173 y =xNew<IssmPDouble>(
ncoord);
184 memcpy(nstr2,nstr,(strlen(nstr)+1)*
sizeof(
char));
186 for (i=0; i<strlen(nstr2); i++)
187 if ((nstr2[i] ==
' ') || (nstr2[i] ==
'\t'))
189 fprintf(fid,
"## Name:%s\n",nstr2);
190 fprintf(fid,
"## Icon:0\n");
191 fprintf(fid,
"# Points Count Value\n");
192 if ((lat[
ncoord-1] != lat[0]) || (lon[
ncoord-1] != lon[0]))
193 fprintf(fid,
"%u %s\n",
ncoord+1,
"1.");
195 fprintf(fid,
"%u %s\n",
ncoord ,
"1.");
196 fprintf(fid,
"# X pos Y pos\n");
201 fprintf(fid,
"%lf\t%lf\n",x[i],y[i]);
202 if ((lat[
ncoord-1] != lat[0]) || (lon[
ncoord-1] != lon[0]))
203 fprintf(fid,
"%lf\t%lf\n",x[0],y[0]);
211 xDelete<double>(lon);
212 xDelete<double>(lat);
int Ll2xyx(double *x, double *y, double *lat, double *lon, int ncoord, int sgn)
#define _printf0_(StreamArgs)
virtual void WriteAttrib(FILE *fid, const char *indent)
void Write(FILE *fid, const char *indent)
#define _printf_(StreamArgs)
void WriteExp(FILE *fid, const char *nstr, int sgn, double cm, double sp)
char altmode[KML_LINEARRING_ALTMODE_LENGTH+1]
: header file for kml file reading utilities.
int KMLFileTagAttrib(KML_Object *kobj, char *ktag)
void Write(FILE *fid, const char *indent)
void Read(FILE *fid, char *kstr)
char * KMLFileToken(FILE *fid, int *pncom=NULL, char ***ppcom=NULL)
int KMLFileTokenParse(int *pival, char *ktag, FILE *fid)
#define _error_(StreamArgs)
: header file for kml_linearring object
#define KML_LINEARRING_ALTMODE_LENGTH
virtual void AddCommnt(int ncom, char **pcom)
virtual void WriteCommnt(FILE *fid, const char *indent)
void Read(FILE *fid, char *kstr)